JavaScript Copy Output to Clipboard
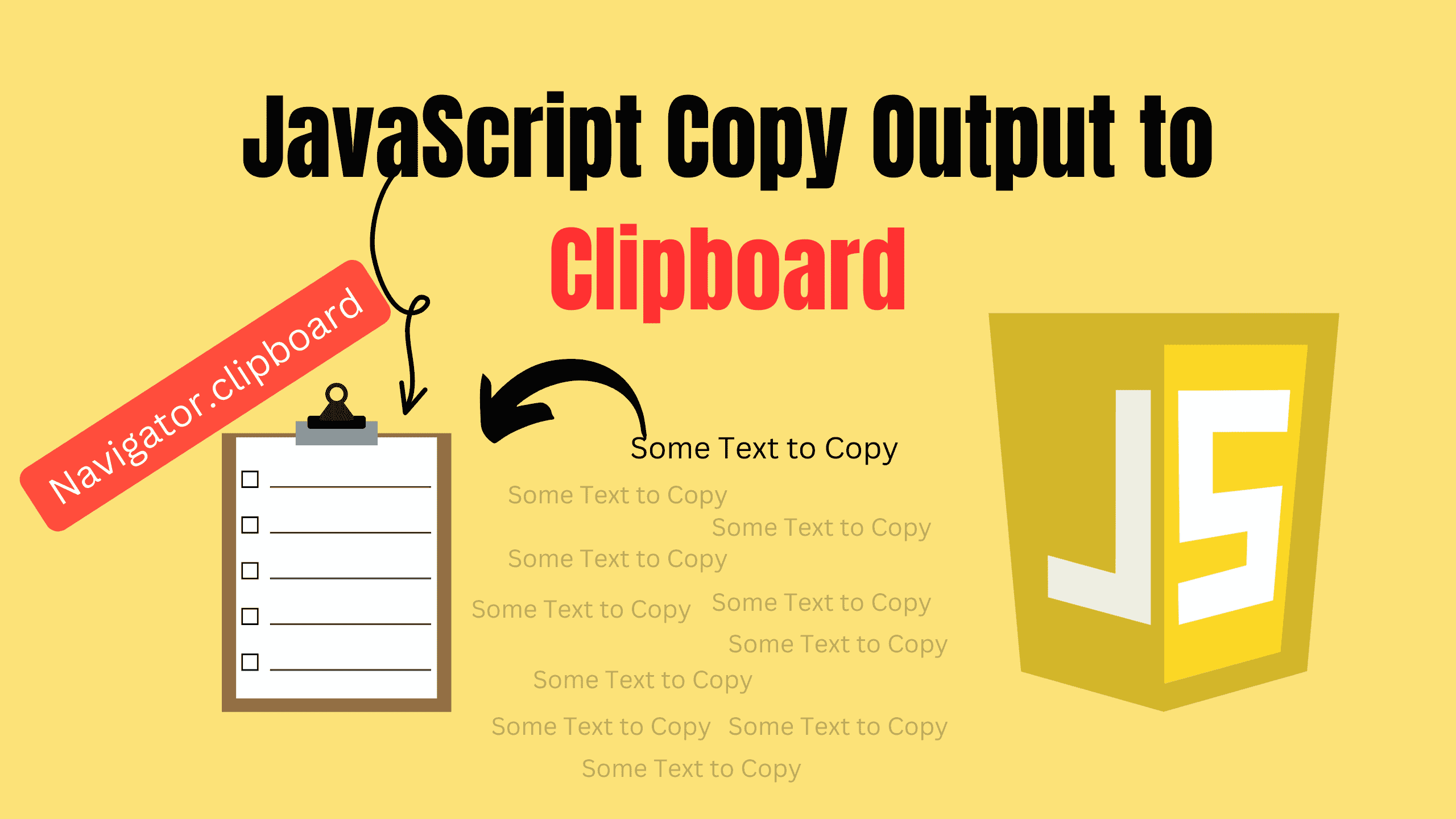
Have you ever visited a website where you clicked a “Copy” button and magically, text was available for pasting anywhere? It’s a small thing, but it makes life easier—no selecting, no right-clicking, no mistakes. In this post, we’ll dive into the how and why of copying text to the clipboard with JavaScript. Spoiler alert: it’s simpler than you think!
Why Should You Care About Copying to the Clipboard?
Let’s face it—users crave convenience. Whether they’re copying a password, sharing a link, or grabbing a code snippet, streamlining the process leaves them with a smile. And happy users? They stick around.
Think of scenarios like:
- Copy-to-share: Social media apps often allow copying post links or captions.
- Code snippets: Developer platforms like GitHub let users copy code blocks in one click.
- Forms: Users might need to copy order IDs, tracking numbers, or promo codes.
The takeaway? Adding a “Copy to Clipboard” feature is not just a nice-to-have—it’s practically essential for modern, user-friendly interfaces.
Clipboard API
The Clipboard interface of the Clipboard API provides read and write access to the contents of the system clipboard. This allows a web application to implement cut, copy, and paste features.
The system clipboard is exposed through the global Navigator.clipboard property.
Methods:
All of the Clipboard API methods operate asynchronously; they return a Promise which is resolved once the clipboard access has been completed. The promise is rejected if clipboard access is denied.
1. read()
Requests arbitrary data (such as images) from the clipboard, returning a Promise that resolves with an array of ClipboardItem objects containing the clipboard's contents.
2. readText()
Requests text from the system clipboard, returning a Promise that is fulfilled with a string containing the clipboard's text once it's available.
3. write()
Writes arbitrary data to the system clipboard, returning a Promise that resolves when the operation completes.
4. writeText()
Writes text to the system clipboard, returning a Promise that is resolved once the text is fully copied into the clipboard.
Implementing a Copy-to-Clipboard Feature
Imagine you’re building a simple web app with a button to copy some text. This is one of the most common and straightforward features to implement, but it can greatly improve the usability and user experience of your application. Users are often presented with text that they want to quickly copy to their clipboard—whether it’s a discount code, a referral link, or some important information.
Having a button that does this in one click saves them the hassle of selecting the text manually and right-clicking to copy. It’s a simple, but powerful feature.
html<div> <p id="text-to-copy">This is the text to copy!</p> <button id="copy-btn">Copy</button> </div>
Here a paragraph of text (or any element with text) and a button that will trigger the copy action when clicked. The button’s role is simple: once clicked, it will run a JavaScript function that accesses the text inside the designated element and sends it to the system clipboard.
jsconst button = document.getElementById("copy-btn"); button.addEventListener("click", () => { const text = document.getElementById("text-to-copy").textContent; navigator.clipboard.writeText(text) .then(() => { console.log("Text copied successfully!"); }) .catch(err => { console.error("Failed to copy text: ", err); }); });
In this example:
- Get the text: We grab it from the p element using textContent.
- Write to clipboard: We call navigator.clipboard.writeText() with the fetched text.
- Handle success and errors: A friendly alert confirms success, while errors get logged. It’s simple, reusable, and enhances the user experience significantly.
Enhancements for Real-World
The above solution is functional, but a polished app goes beyond functionality. Here are a few enhancements to consider:
1. Feedback UI:
Add visual cues like changing button text to
jsconst button = document.getElementById("copy-btn"); button.addEventListener("click", () => { const text = document.getElementById("text-to-copy").textContent; navigator.clipboard.writeText(text) .then(() => { console.log("Text copied successfully!"); button.textContent = "Copied!"; setTimeout(() => button.textContent = "Copy to Clipboard", 2000); }) .catch(err => { console.error("Failed to copy text: ", err); }); });
2. Accessibility:
Ensure buttons and alerts are accessible. Use ARIA attributes for users relying on screen readers.
3. Security Considerations:
Browsers restrict clipboard access for security reasons, so always trigger clipboard actions via a user interaction (like a button click).
Conclusion
Copying text to the clipboard is one of those “small but mighty” features that can elevate your web app from functional to delightful. With JavaScript’s Clipboard API, it’s easier than ever to implement.
As developers, our goal should always be to make tasks simpler and faster for users. Adding a copy-to-clipboard feature is one way to do just that. And hey, it’s also pretty fun to build!
So, what are you waiting for? Go on and add some copy magic to your app! Your users will thank you for it.
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!