Python Switch Statement - A Comprehensive Guide
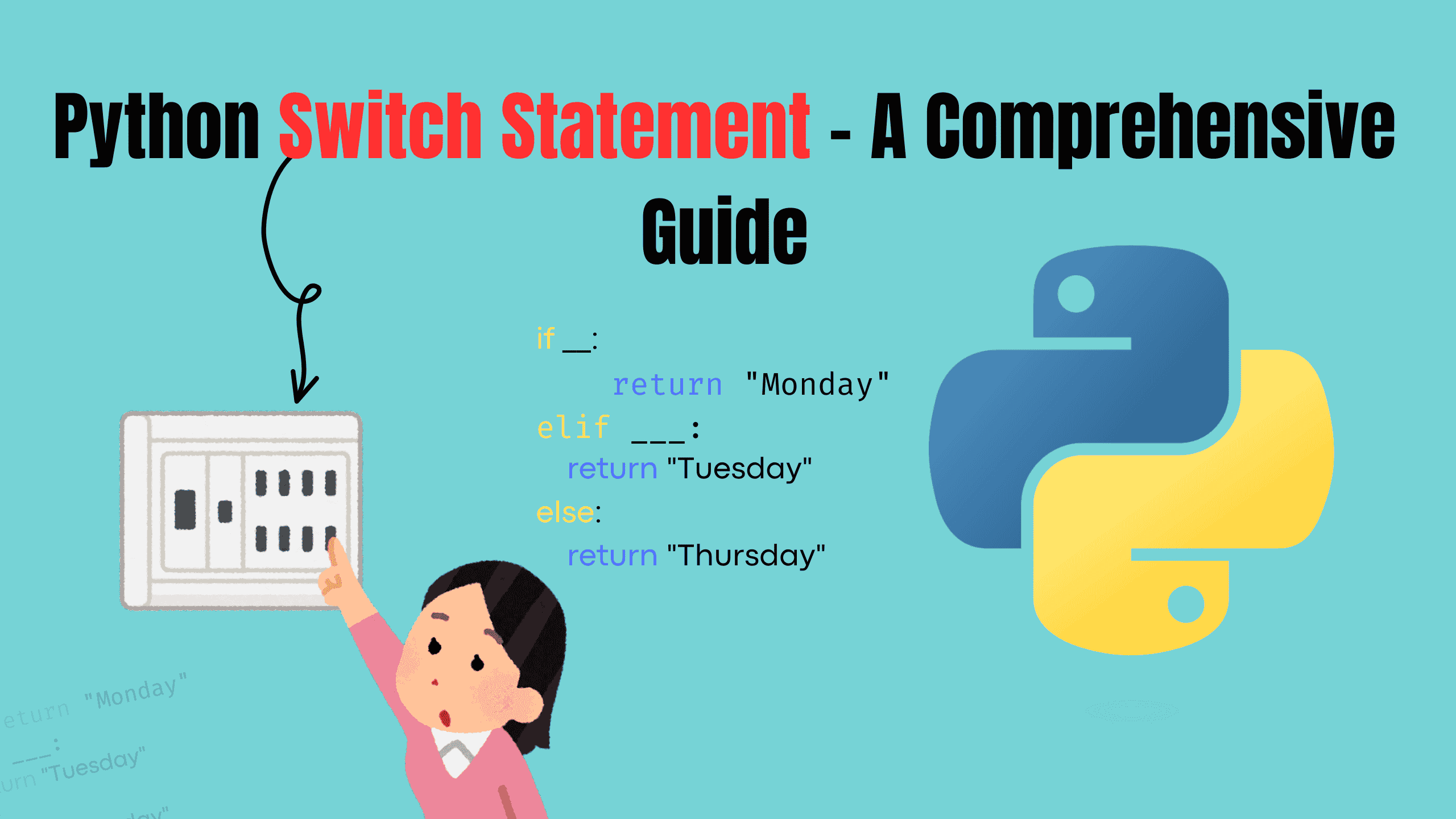
Hey there, Python explorers!
Understanding the Missing Puzzle in Python
If you’ve dabbled with programming languages like C, C++, or Java, you’ve probably encountered the versatile switch statement. It’s one of those constructs that make code not only look tidy but also run efficiently. So, naturally, when transitioning to Python, many developers are baffled by its absence.
Yes, you read that right: Python doesn’t have a native switch statement. At first glance, this omission might feel limiting, especially if you’re crafting conditional-heavy logic. But trust me, the story doesn’t end here. In this blog, we’ll dive deep into why Python doesn’t have a switch statement and how you can implement equivalent solutions using its rich set of features.
Why Doesn't Python Have a Switch Statement?
The absence of a switch statement isn’t a random oversight. Python’s philosophy revolves around simplicity and readability, as articulated in The Zen of Python. Many believe that traditional switch-case constructs from other languages can often be replaced by Python’s powerful if-elif-else structure.
Additionally, Python's dictionary-based approach provides a more Pythonic way to mimic the behavior of a switch, making your code both elegant and dynamic. That said, let’s explore these alternatives.
Approaches to Mimic a Switch in Python
While Python does not have a built-in switch statement, there are several ways to achieve similar functionality. These approaches leverage Python's flexibility and readability, allowing you to write clean and efficient code. Let's explore some of these methods.
1. If-Elif-Else Statements: The Classic Approach
The simplest way to emulate a switch statement is to use if-elif-else constructs. Consider this example:
pydef day_of_week(day): if day == 1: return "Monday" elif day == 2: return "Tuesday" elif day == 3: return "Wednesday" elif day == 4: return "Thursday" elif day == 5: return "Friday" elif day == 6: return "Saturday" elif day == 7: return "Sunday" else: return "Invalid day"
This method works well but can become unwieldy with many cases. Plus, it lacks the direct mapping clarity offered by other approaches.
Best for simple logic or when Python versions below 3.10 are in play.
2. Dictionaries: The Pythonic Way
Using dictionaries is a more concise and Pythonic way to mimic a switch. Here's an example:
pydef day_of_week(day): switch = { 1: "Monday", 2: "Tuesday", 3: "Wednesday", 4: "Thursday", 5: "Friday", 6: "Saturday", 7: "Sunday" } return switch.get(day, "Invalid day")
Doesn’t that look cleaner? This approach leverages the dict.get() method to handle default values elegantly.
Ideal for static mappings, offering clarity and brevity.
3. Functions in Dictionaries: Adding Dynamism
What if your switch logic involves more than just returning values? Python dictionaries can store functions, adding a layer of dynamic behavior:
pydef case_one(): return "Case One Executed" def case_two(): return "Case Two Executed" def default_case(): return "Default Case Executed" def switch_case(case): switch = { 1: case_one, 2: case_two } return switch.get(case, default_case)()
By storing functions as values, you’re opening the door to highly modular and reusable code. Plus, the default case adds a safety net for unexpected inputs.
Perfect for dynamic logic or complex operations.
4. Match-Case: A Game Changer in Python 3.10+
Python 3.10 introduced a long-awaited feature: the match-case statement. While it’s not officially called a switch, it serves the same purpose:
pydef day_of_week(day): match day: case 1: return "Monday" case 2: return "Tuesday" case 3: return "Wednesday" case 4: return "Thursday" case 5: return "Friday" case 6: return "Saturday" case 7: return "Sunday" case _: return "Invalid day"
Notice the use of the underscore _ for the default case—elegant, isn’t it? The match-case statement is arguably the closest Python has come to implementing a switch, combining simplicity with power.
The go-to option if you’re using Python 3.10 or newer.
Conclusion
While Python lacks a native switch statement, its alternatives more than make up for it. Whether you choose the traditional if-elif-else approach, the elegance of dictionaries, or the futuristic match-case, Python empowers you to write clear and maintainable code.
So, the next time someone asks, “Where’s Python’s switch statement?” smile confidently and show them these creative solutions. Remember, Python is all about finding beauty in simplicity—and these alternatives prove just that.
Happy coding!
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!