Python List - A Comprehensive Guide
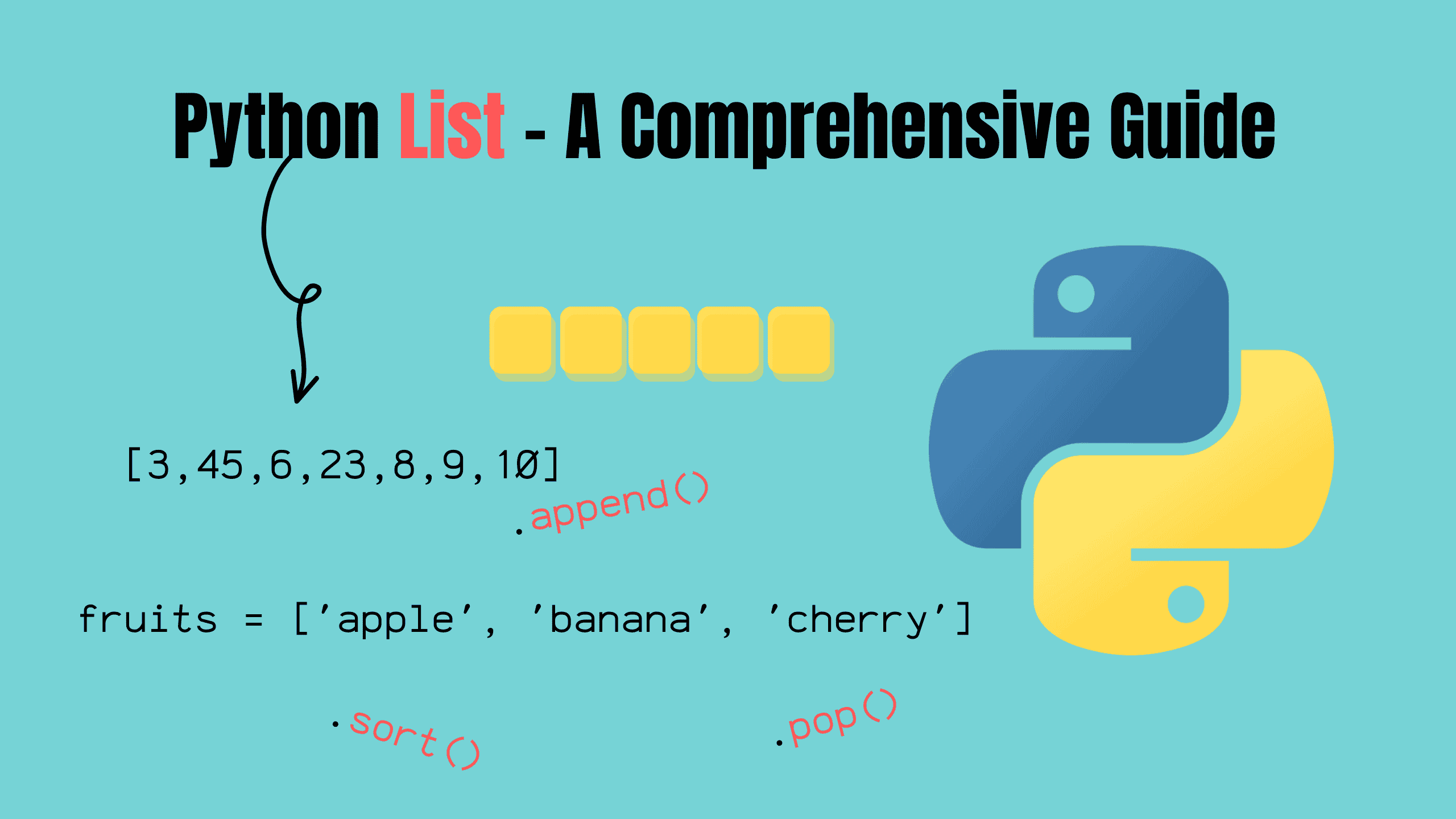
Hey there, Python explorers!
Python lists are a fundamental and versatile feature of the language, enabling developers to store and manipulate collections of items with ease. In this comprehensive guide, we'll explore the ins and outs of Python lists, from their creation and manipulation to advanced techniques that can enhance your coding efficiency.
What Is a Python List?
A Python list is an ordered collection of items, which can be of different data types, enclosed in square brackets [] and separated by commas. Lists are mutable, meaning their elements can be changed after creation, and they allow duplicate values.
py# Example of a Python list fruits = ['apple', 'banana', 'cherry']
fruits is a list containing three string elements.
Creating Lists
Lists can be created in multiple ways to suit different needs and situations. You can define a list using square brackets [], separating items with commas. Lists can also be created by using built-in functions like list(), which can convert other iterable types such as tuples or strings into lists. Whether you populate it with numbers, strings, or mixed data types, lists are versatile and easy to work with in Python.
1. Using Square Brackets:
The most common and straightforward way to create a list is by using square brackets []. Simply enclose the items you want in the list, separated by commas.
pynumbers = [1, 2, 3, 4, 5]
2. Using the list() Constructor:
You can also create a list using the list() function, which is particularly useful when converting other iterable types into lists.
pyletters = list(['a', 'b', 'c'])
3. From Iterables:
Lists can be constructed from any iterable, such as strings, tuples, or other collections. For instance, converting a string into a list of characters:
py# From a string char_list = list('hello') # Output: ['h', 'e', 'l', 'l', 'o']
Accessing List Elements
Each item in a list has an index, starting from 0 for the first item. You can access elements using their index:
pyprint(fruits[0]) # Output: apple print(fruits[2]) # Output: cherry
Python also supports negative indexing, where -1 refers to the last item, -2 to the second last, and so on.
pyprint(fruits[-1]) # Output: cherry print(fruits[-2]) # Output: banana
Modifying Lists
Lists in Python are mutable, which means you can modify their content after they are created. This flexibility makes lists one of the most versatile data structures in Python. Here’s a how to modify lists:
Changing an Element
You can directly update any element in a list by referencing its index and assigning it a new value.
pyfruits[1] = 'blueberry' print(fruits) # Output: ['apple', 'blueberry', 'cherry']
Adding Elements
Python provides several ways to add items to a list, depending on your needs:
1. Using append()
The append() method adds a single element to the end of the list.
pyfruits.append('date') print(fruits) # Output: ['apple', 'blueberry', 'cherry', 'date']
2. Using insert()
The insert() method lets you add an element at a specific position in the list.
pyfruits.insert(1, 'banana') print(fruits) # Output: ['apple', 'banana', 'blueberry', 'cherry', 'date']
3. Using extend()
The extend() method appends multiple elements from another iterable (like a list or a tuple) to the end of the list.
pymore_fruits = ['elderberry', 'fig'] fruits.extend(more_fruits) print(fruits) # Output: ['apple', 'banana', 'blueberry', 'cherry', 'date', 'elderberry', 'fig']
Removing Elements
You can also remove elements from a list in different ways:
1. Using remove()
The remove() method deletes the first occurrence of a specific value.
pyfruits.remove('banana') print(fruits) # Output: ['apple', 'blueberry', 'cherry', 'date', 'elderberry', 'fig']
2. Using pop()
The pop() method removes an element at a specific index (default is the last element) and returns it.
pylast_fruit = fruits.pop() print(last_fruit) # Output: fig print(fruits) # Output: ['apple', 'blueberry', 'cherry', 'date', 'elderberry']
3. Using clear()
If you want to remove all elements from the list, the clear() method is your go-to.
pyfruits.clear() print(fruits) # Output: []
List Methods
Python provides a variety of built-in methods to work with lists:
- append(x): Adds an item x to the end of the list.
- extend(iterable): Extends the list by appending elements from the iterable.
- insert(i, x): Inserts an item x at a given position i.
- remove(x): Removes the first occurrence of an item x.
- pop([i]): Removes and returns the item at position i (default is the last item).
- clear(): Removes all items from the list.
- index(x[, start[, end]]): Returns the index of the first occurrence of an item x.
- count(x): Returns the number of times x appears in the list.
- sort(key=None, reverse=False): Sorts the items of the list in place.
- reverse(): Reverses the elements of the list in place.
- copy(): Returns a shallow copy of the list.
Iterating Over Lists
You can iterate over the items in a list using a for loop:
pyfor fruit in fruits: print(fruit)
This will print each fruit in the list on a new line.
List Comprehensions
List comprehensions provide a concise way to create lists. Common applications include making new lists where each element is the result of some operation applied to each member of another sequence or iterable.
py# Create a list of squares squares = [x**2 for x in range(1, 6)] print(squares) # Output: [1, 4, 9, 16, 25]
List comprehensions can also include conditions:
py# Create a list of even numbers evens = [x for x in range(10) if x % 2 == 0] print(evens) # Output: [0, 2, 4, 6, 8]
Nested Lists
Python allows the creation of nested lists, meaning lists within lists. This feature is particularly useful for representing complex structures like matrices, tables, or grids.
pymatrix = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ] # Accessing elements in nested lists print(matrix[0][1]) # Output: 2 (first row, second column)
Nested lists can be iterated using nested loops:
pyfor row in matrix: for item in row: print(item, end=" ") # Output: 1 2 3 4 5 6 7 8 9
Practical Uses of Nested Lists
- Storing tabular data: Like spreadsheet data with rows and columns.
- Graph representation: Adjacency lists for nodes.
- Game boards: Representing grids like in chess or tic-tac-toe.
Advanced List Techniques
Python lists offer a range of advanced techniques that can help you manipulate and process data efficiently. Below are some powerful methods to take your list handling to the next level.
Slicing Lists
Slicing allows you to extract specific parts of a list, providing a flexible way to access subsets of data. The syntax is list[start:end:step], where:
- start is the index to begin the slice (inclusive).
- end is the index to stop the slice (exclusive).
- step specifies the interval between indices.
py#### Slicing syntax: list[start:end:step] numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9] print(numbers[2:5]) # Output: [2, 3, 4] print(numbers[:4]) # Output: [0, 1, 2, 3] print(numbers[::2]) # Output: [0, 2, 4, 6, 8] print(numbers[::-1]) # Output: [9, 8, 7, 6, 5, 4, 3, 2, 1, 0] (reversed list)
List Comprehensions with Conditions
List comprehensions provide a concise way to create lists. Adding conditions makes them even more powerful for filtering and transforming data in a single line of code.
py# Filter and process data nums = [1, 2, 3, 4, 5, 6] squared_evens = [n**2 for n in nums if n % 2 == 0] print(squared_evens) # Output: [4, 16, 36]
Zipping and Unzipping Lists
The zip() function combines two or more lists into a sequence of tuples, pairing elements by their positions. This is particularly useful when you need to process related data together.
pynames = ['Alice', 'Bob', 'Charlie'] scores = [85, 90, 95] # Combine lists zipped = zip(names, scores) print(list(zipped)) # Output: [('Alice', 85), ('Bob', 90), ('Charlie', 95)]
To unzip, use zip(*iterables):
pyzipped = [('Alice', 85), ('Bob', 90), ('Charlie', 95)] names, scores = zip(*zipped) print(names) # Output: ('Alice', 'Bob', 'Charlie') print(scores) # Output: (85, 90, 95)
Copying Lists
Copying a list creates a new list with the same elements as the original. This ensures modifications to one list won’t affect the other. Lists can be copied using:
1. Slicing:
Slicing creates a shallow copy of the list:
pyoriginal = [1, 2, 3] copy = original[:]
2. list() constructor:
Another way to create a shallow copy:
pycopy = list(original)
3. copy() method:
The copy() method, available in Python 3.3 and later, is a convenient built-in option:
pycopy = original.copy()
4. Using copy module:
For nested lists or complex structures, use the copy.deepcopy() method to create an independent copy:
pyimport copy deep_copy = copy.deepcopy(original) # For nested lists
Sorting Lists
Python’s sorting features allow you to organize lists efficiently:
1. In-place sorting:
The sort() method modifies the original list:
pynums = [3, 1, 4, 1, 5] nums.sort() print(nums) # Output: [1, 1, 3, 4, 5]
2. Using sorted():
The sorted() function returns a sorted copy of the list, leaving the original list unchanged:
pynums = [3, 1, 4, 1, 5] sorted_nums = sorted(nums) print(sorted_nums) # Output: [1, 1, 3, 4, 5]
3. Custom Sorting with key
You can sort based on specific criteria using the key parameter:
pywords = ['banana', 'apple', 'cherry'] words.sort(key=len) print(words) # Output: ['apple', 'banana', 'cherry']
Using any() and all()
- any(): Returns True if any element in the list is truthy.
- all(): Returns True if all elements in the list are truthy.
pynums = [0, 1, 2, 3] print(any(nums)) # Output: True (at least one truthy element) print(all(nums)) # Output: False (0 is falsy)
Combining Lists with + or *
You can merge or repeat lists using + and * operators.
py# Merge lists a = [1, 2] b = [3, 4] print(a + b) # Output: [1, 2, 3, 4] # Repeat list print(a * 3) # Output: [1, 2, 1, 2, 1, 2]
Real-World Applications of Lists
Python lists are incredibly versatile and find applications in a wide range of fields. Their ability to store and manipulate ordered collections of data makes them a fundamental data structure in programming. Here’s how they are used across various domains:
- Data Analysis: Lists serve as the backbone for storing and processing datasets in Python. Often used with libraries like Pandas and NumPy for advanced analysis.
- Web Development: Lists are commonly used to manage data like user input, form fields, and database results.
- Game Development: In games, lists are often used for managing player inventories, game levels, or storing coordinates.
Common Pitfalls with Lists
While Python lists are flexible, they come with a few pitfalls:
- Performance Issues: Lists aren't ideal for heavy computational tasks involving frequent insertions/deletions, especially for large datasets. Consider deque from collections for such cases.
- Mutable Default Arguments: Be cautious when using lists as default function arguments.
pydef add_item(item, my_list=[]): my_list.append(item) return my_list print(add_item(1)) # Output: [1] print(add_item(2)) # Output: [1, 2]
Instead, use None as the default:
pydef add_item(item, my_list=None): if my_list is None: my_list = [] my_list.append(item) return my_list
Conclusion
Python lists are a cornerstone of the language, providing developers with an incredible amount of flexibility and power. From basic operations to advanced techniques like slicing, comprehensions, and nested structures, mastering lists is essential for any Python programmer. Whether you’re building a data pipeline, creating a game, or manipulating text, lists will be your go-to tool.
So go ahead—experiment with lists, play with their methods, and integrate them into your next project. They’re versatile, efficient, and quintessentially Pythonic!
Happy coding!
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!