Python Split Method - A Comprehensive Guide
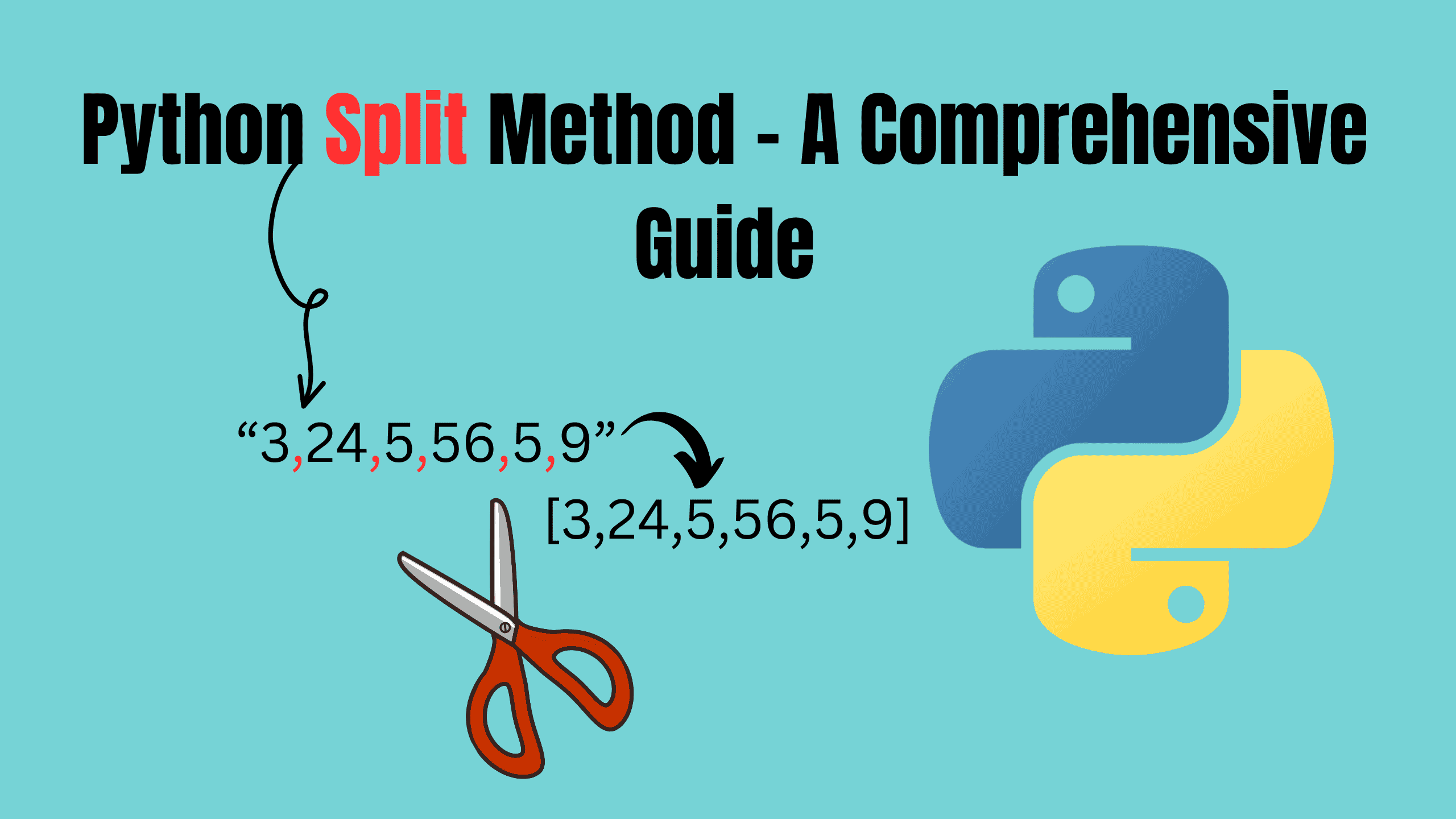
Python is one of the most widely used programming languages, celebrated for its simplicity and versatility. Among its many built-in features, the split() method is a powerful tool for manipulating strings.
This blog will dive deep into understanding how the split() method works, its various use cases, and how it can make your string-handling tasks more efficient. By the end, you’ll be equipped with a solid grasp of this essential Python function.
What is the split() Method in Python?
The split() method in Python is a built-in string method that divides a string into a list of substrings based on a specified delimiter. If no delimiter is provided, it defaults to splitting the string by whitespace (spaces, tabs, or newlines).
Its syntax is straightforward:
pythonstring.split(separator, maxsplit)
- separator(optional): The delimiter or character(s) used to split the string. It is optional and defaults to any whitespace.
- maxsplit(optional): The maximum number of splits to perform. It is optional and defaults to -1, which means "no limit."
The method returns a list of substrings derived from the original string.
Basic Usage of split()
Let’s look at a few simple examples to get started.
1. Splitting by Default Whitespace
When no separator is provided, split() defaults to using any whitespace as the delimiter, making it ideal for splitting sentences into words.
pythontext = "Python is easy to learn" words = text.split() print(words) # Output # ['Python', 'is', 'easy', 'to', 'learn']
In this example, the method identifies spaces in the string and divides it into individual words.
2. Splitting Using a Specific Delimiter
Specifying a custom separator, such as a comma, to divide the string. This is especially useful when dealing with comma-separated data like CSV files.
pythontext = "apple,banana,cherry" fruits = text.split(',') print(fruits) # Output # ['apple', 'banana', 'cherry']
Here, the string is split by commas, creating a list of fruit names.
3. Using the maxsplit Parameter
The maxsplit parameter limits the number of splits performed, leaving the rest of the string intact.
pythontext = "one two three four" words = text.split(' ', 2) print(words) # Output # ['one', 'two', 'three four']
The maxsplit parameter limits the number of splits to 2, so only the first two spaces are used to divide the string. The remaining part of the string is left as-is.
Advanced Use Cases
The split() method is not limited to basic string manipulation. It can handle complex operations and scenarios when paired with other Python functionalities.
1. Splitting Multi-line Strings
In many cases, strings may span multiple lines, such as when reading data from text files. The split() method can divide the content based on newline characters.
pythontext = "Line1\nLine2\nLine3" lines = text.split('\n') print(lines) # Output # ['Line1', 'Line2', 'Line3']
This use case is common in text parsing or data extraction tasks where content is organized across lines.
2. Handling Mixed Delimiters
Strings may sometimes include multiple types of delimiters. The split() method handles only one delimiter at a time, but you can combine it with the re.split() function from Python’s regular expressions module for more advanced scenarios.
pythonimport re text = "apple;banana|cherry,grape" fruits = re.split(';|,|\|', text) print(fruits) # Output # ['apple', 'banana', 'cherry', 'grape']
Here, the re.split() function uses a pattern to identify semicolons, commas, or pipe characters as valid delimiters.
3. Stripping Extra Whitespace Before Splitting
Sometimes strings include unwanted leading or trailing spaces, which can interfere with splitting. In such cases, use the strip() method before calling split().
pythontext = " Python is fun " words = text.strip().split() print(words) # Output # ['Python', 'is', 'fun']
This ensures cleaner results when processing user input or poorly formatted text data.
Comparison with Other String Methods
The split() method is often compared with other string manipulation methods. Let’s explore how it stacks up against similar functions like splitlines() and partition().
1. split() vs splitlines()
The splitlines() method splits a string into a list based on line breaks. This is different from split(), which requires a specific separator.
pythontext = "Line1\nLine2\nLine3" print(text.split()) # Default whitespace print(text.splitlines()) # Line breaks # Output # ['Line1', 'Line2', 'Line3'] from split() # ['Line1', 'Line2', 'Line3'] from splitlines()
2. split() vs partition()
While split() divides a string into multiple parts, the partition() method splits it into exactly three parts: the part before the first occurrence of the separator, the separator itself, and the part after.
pythontext = "Python is fun" print(text.split('is')) print(text.partition('is')) # Output # ['Python ', ' fun'] from split() # ('Python ', 'is', ' fun') from partition()
Common Pitfalls to Avoid
- Using an Empty Separator Passing an empty string ('') as a separator causes a ValueError, as Python doesn’t support splitting strings in this way.
pythontext = "hello" print(text.split('')) # Error: # ValueError: empty separator
- Confusing maxsplit with List Length The maxsplit parameter controls the number of splits, not the total number of list items. Be cautious when using it.
pythontext = "one two three" print(text.split(' ', 1)) # Output # ['one', 'two three']
- Overlooking Default Behavior When no separator is provided, split() treats all whitespace as valid delimiters, including tabs and newlines.
pythontext = "one\ttwo\nthree" print(text.split()) # Output # ['one', 'two', 'three']
Practical Applications
1. Reading CSV Data
Splitting comma-separated values is a common task when processing CSV files.
pythondata = "name,age,city" fields = data.split(',') print(fields) # Output # ['name', 'age', 'city']
2. Tokenizing Text
Tokenization is the process of breaking down text into smaller units, like words or phrases. The split() method is a simple yet effective tool for this.
pythontext = "Natural Language Processing with Python" tokens = text.split() print(tokens) # Output # ['Natural', 'Language', 'Processing', 'with', 'Python']
3. Parsing Log Files
When working with server logs, you might need to extract information from log lines.
pythonlog = "2024-12-16 10:00:00 INFO User logged in" parts = log.split(' ') print(parts) # Output # ['2024-12-16', '10:00:00', 'INFO', 'User', 'logged', 'in']
Conclusion
The split method is a fundamental tool in Python’s string-handling arsenal. Its versatility, simplicity, and power make it indispensable for developers dealing with text data. Whether you are tokenizing text, processing logs, or reading CSV files, understanding the nuances of split() will significantly enhance your productivity.
By mastering the basics and exploring advanced use cases, you can unlock its full potential and apply it to a wide range of programming scenarios.
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!