Understanding Try and Except in Python
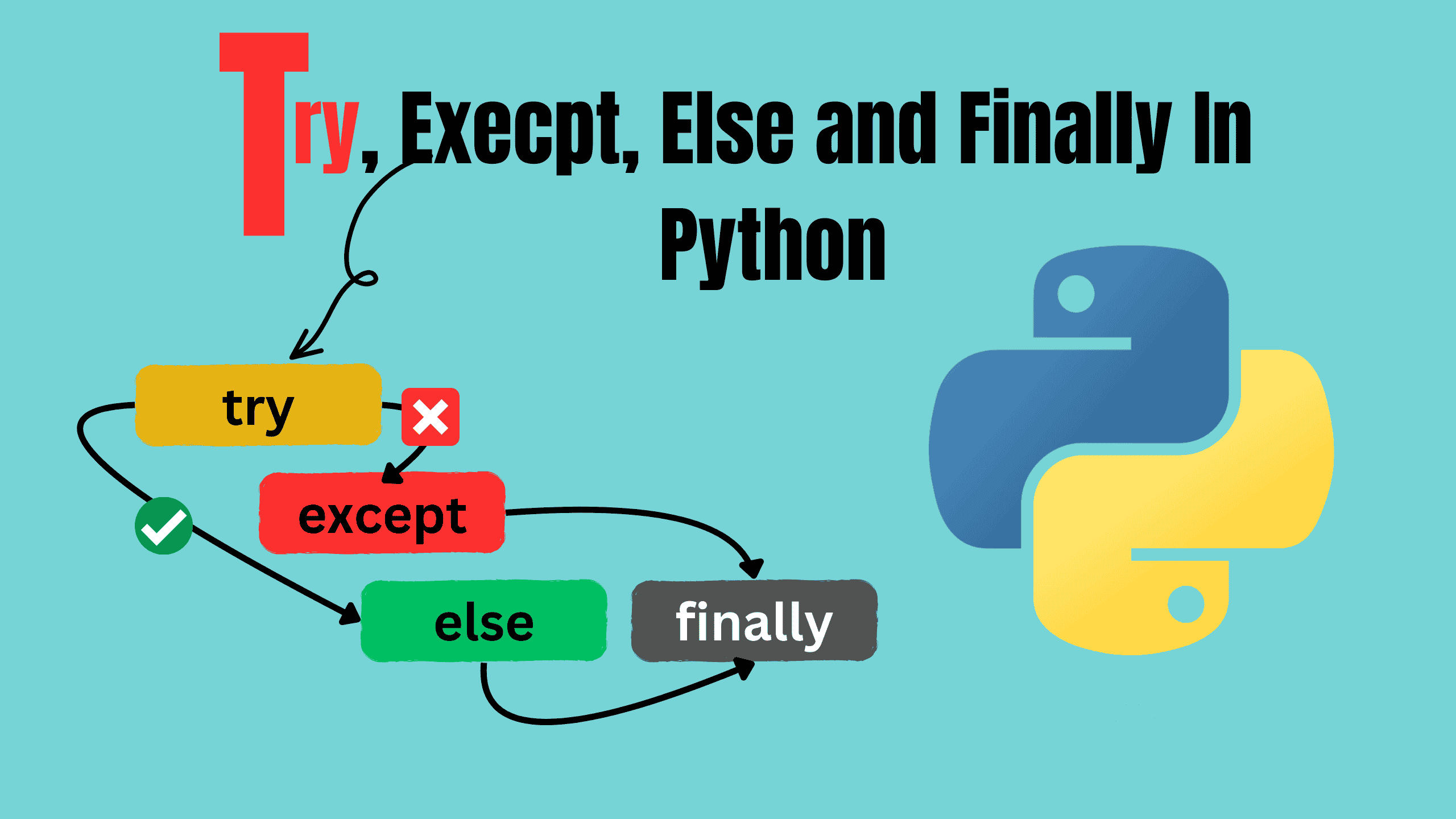
Hey there, Python explorers!
Today, we’re diving into one of Python’s most powerful and user-friendly features: try and except. Whether you’re a beginner or a seasoned coder, handling errors gracefully is an essential skill, and Python makes it as simple as a safety net that catches you when you fall. Ready to explore?
Why Do Errors Matter?
Errors happen. It’s an inevitable part of coding. You might think your program is bulletproof, but unexpected user inputs, missing files, or even unforeseen edge cases can derail your code in seconds.
Without error handling, your program may crash, frustrating users and potentially causing data loss. But what if you could catch these issues and handle them gracefully? That’s where try and except shine. They don’t just prevent crashes; they give you the tools to respond to errors in a way that’s helpful, intuitive, and professional.
What Are Try and Except?
In simple terms, try is where you put the code that might throw an error. If an error does occur, Python skips over the rest of the try block and executes the corresponding except block. It’s like saying, “Try this code, but if something goes wrong, here’s what to do instead.”
Here’s a tiny example:
pytry: number = int(input("Enter a number: ")) print(f"Your number is {number}.") except ValueError: print("Oops! That’s not a valid number.")
The code inside the try block runs first.
- If there’s no error, everything moves along smoothly.
- If an error does occur (like entering letters instead of numbers), Python jumps to the except block. The program doesn’t crash. Instead, you get a friendly message like, “Oops!” Pretty neat, right?
Why Should You Care?
Here are some key reasons why error handling is essential in programming:
- Improves User Experience: No one likes cryptic error messages or programs that abruptly stop working. Catching errors lets you provide meaningful feedback to users.
- Keeps Your Program Running: Instead of halting execution, error handling ensures your program can continue functioning (or at least exit gracefully).
- Debugging Made Easier: By identifying and catching specific errors, you can pinpoint where things went wrong without wading through unnecessary crash reports.
Exploring try, except, else, and finally
Python gives you more than just try and except. Let’s take a closer look at how you can handle different scenarios.
1. Basic try and except
Here’s a simple example to catch input errors:
pytry: user_age = int(input("Enter your age: ")) print(f"You are {user_age} years old!") except ValueError: print("Please enter a valid number.")
If the user enters something like "twenty" instead of a number, the program will handle the error without crashing.
2. Using Multiple except Blocks
Sometimes, you need to handle different types of errors differently. Python lets you stack multiple except blocks:
pytry: number = int(input("Enter a number: ")) result = 10 / number print(f"The result is {result}.") except ZeroDivisionError: print("You can’t divide by zero. Try again!") except ValueError: print("That’s not a valid number. Please enter a numeric value.")
What’s Happening Here?
- If the user enters 0, the ZeroDivisionError block is triggered.
- If the user enters something non-numeric, the ValueError block handles it. This makes your code more adaptable and responsive to different situations.
3. Adding else and finally
else:
The else block runs if no errors occur in the try block. It’s a great way to separate error-free logic from error-handling logic.
pytry: number = int(input("Enter a number: ")) except ValueError: print("That’s not a valid number.") else: print(f"You entered {number}, and everything worked perfectly!")
finally:
The finally block runs no matter what — whether an error occurred or not. It’s often used for cleanup tasks like closing files or releasing resources.
pytry: file = open("example.txt", "r") content = file.read() print(content) except FileNotFoundError: print("The file doesn’t exist.") finally: print("Closing the file.") file.close()
Here's how try, except, else and finally looks:
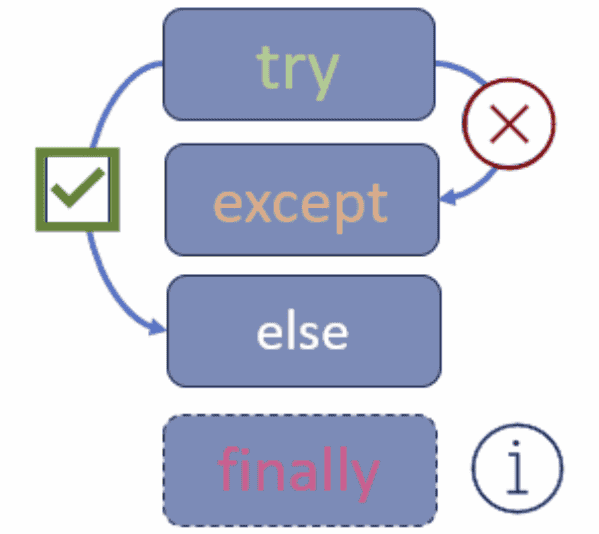
Common Errors and How to Handle Them
Here’s a quick guide to some common Python errors you’ll encounter and how to handle them with try and except:
1. ValueError
Occurs when you try to convert an invalid value.
pytry: number = int("text") except ValueError: print("Invalid value! Please enter a number.")
2. ZeroDivisionError
Triggered when dividing by zero.
pytry: result = 5 / 0 except ZeroDivisionError: print("Division by zero is not allowed!")
3. FileNotFoundError
Occurs when a file you’re trying to open doesn’t exist.
pytry: with open("nonexistent_file.txt", "r") as file: content = file.read() except FileNotFoundError: print("Oops! File not found.")
4. TypeError
Raised when an operation involves incompatible types.
pytry: result = "hello" + 5 except TypeError: print("Type mismatch: You can’t add a string and a number.")
5. KeyError
Happens when trying to access a non-existent dictionary key.
pytry: my_dict = {"name": "Python"} print(my_dict["age"]) except KeyError: print("The key doesn’t exist in the dictionary.")
Pro Tips for Error Handling in Python
- Be Specific with Errors: Always catch specific exceptions when possible. Avoid using a generic except: unless absolutely necessary.
- Log Errors: Instead of just printing error messages, log them for future debugging. Python’s logging module is great for this.
- Don’t Ignore Errors: Silent failures can make debugging a nightmare. Always handle errors in a meaningful way.
- Keep Code Readable: Overusing try and except can clutter your code. Use them judiciously to maintain readability.
Conclusion
Errors don’t have to ruin your day (or your code). With try and except, you can handle problems like a pro and keep your program running smoothly. It’s Python’s way of saying, “I’ve got your back.”
Now you know how to handle everything from invalid inputs to missing files with confidence. Go ahead, give it a try (pun intended), and make your code error-proof. Happy coding!
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!