Animating 3D Objects in Three.js (Beginner Guide 03)
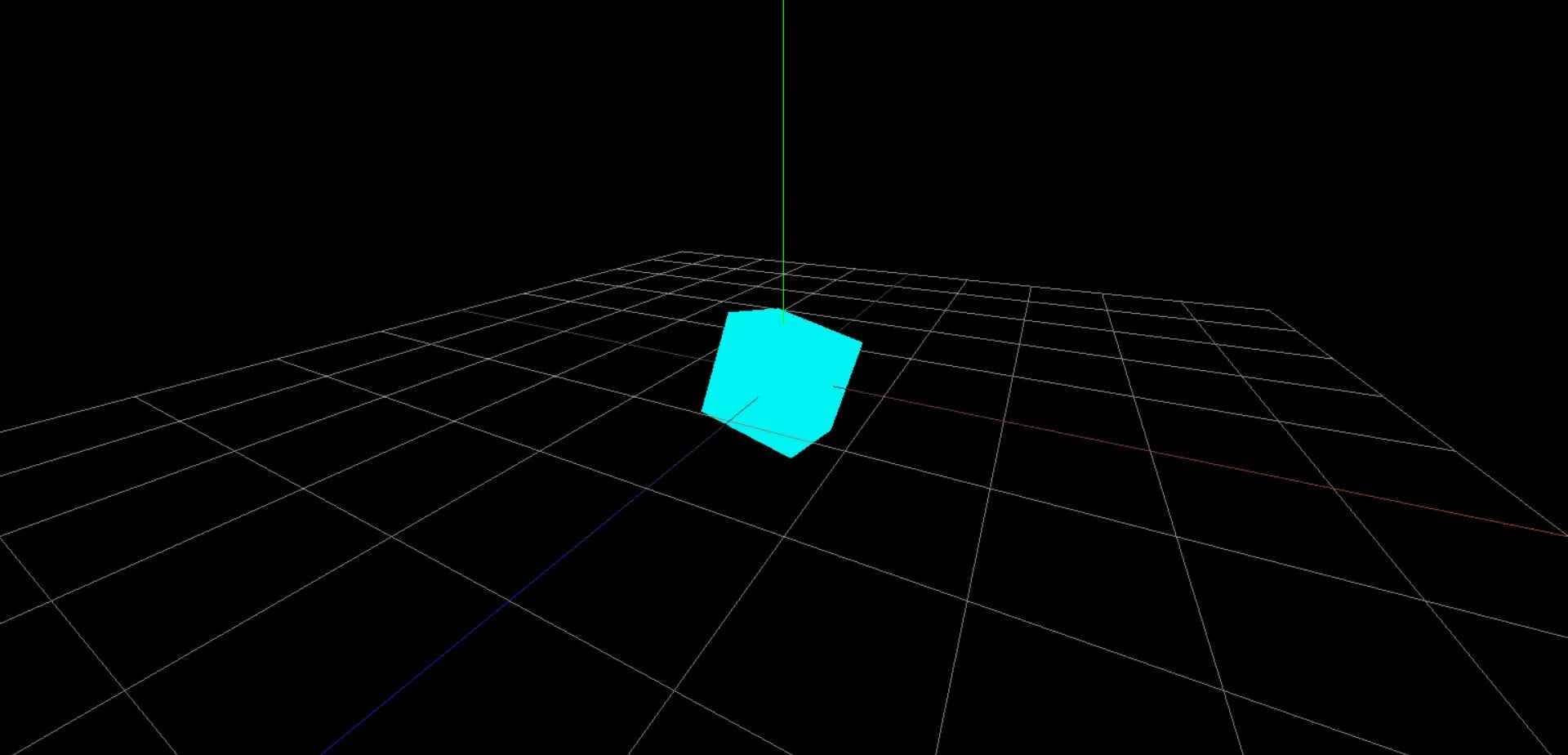
Animations can bring your Three.js scenes to life. In this blog, we'll explore how to animate 3D objects in a Three.js scene.
Creating an Animation Loop
In Three.js, the most common way to create animations is through an animation loop. An animation loop is a function that updates the scene and then re-renders it. This function is called repeatedly, creating the illusion of movement.
js// Create an animation loop function animate() { requestAnimationFrame(animate); // Rotate the cube cube.rotation.x += 0.01; cube.rotation.x += 0.01; // Render the scene renderer.render(scene, camera); } animate();
This code will create a cube that rotates about the x and y axes.
Full Code
Check below how the full code will look like:
jsimport './style.css' import * as THREE from 'three'; // Initialize Three.js const scene = new THREE.Scene(); const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000); camera.position.set(2, 2, 4); camera.lookAt(0, 0, 0); const renderer = new THREE.WebGLRenderer(); renderer.setSize(window.innerWidth, window.innerHeight); document.body.appendChild(renderer.domElement); // Create a geometry let geometry = new THREE.BoxGeometry(1, 1, 1); // Create a material let material = new THREE.MeshBasicMaterial({ color: 0xf2f2 }); // Create a mesh let cube = new THREE.Mesh(geometry, material); // Add the cube to the scene scene.add(cube); // Create an ambient light let ambientLight = new THREE.AmbientLight(0xfffff, 5); // Add the light to the scene scene.add(ambientLight); // Create a grid helper with size 10 and divisions 10 let gridHelper = new THREE.GridHelper(10, 10); // Add the grid helper to the scene scene.add(gridHelper); // Create an axes helper with size 5 let axesHelper = new THREE.AxesHelper(5); // Add the axes helper to the scene scene.add(axesHelper); // Create an animation loop function animate() { requestAnimationFrame(animate); // Rotate the cube cube.rotation.x += 0.01; cube.rotation.x += 0.01; // Render the scene renderer.render(scene, camera); } animate();
Conclusion
In this blog, we learned how to animate 3D objects in a Three.js scene. Animation is a powerful tool that can greatly enhance your 3D scenes. If you have any questions, don't hesitate to reach out.
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!