JavaScript Move Items to Cart with mousemove
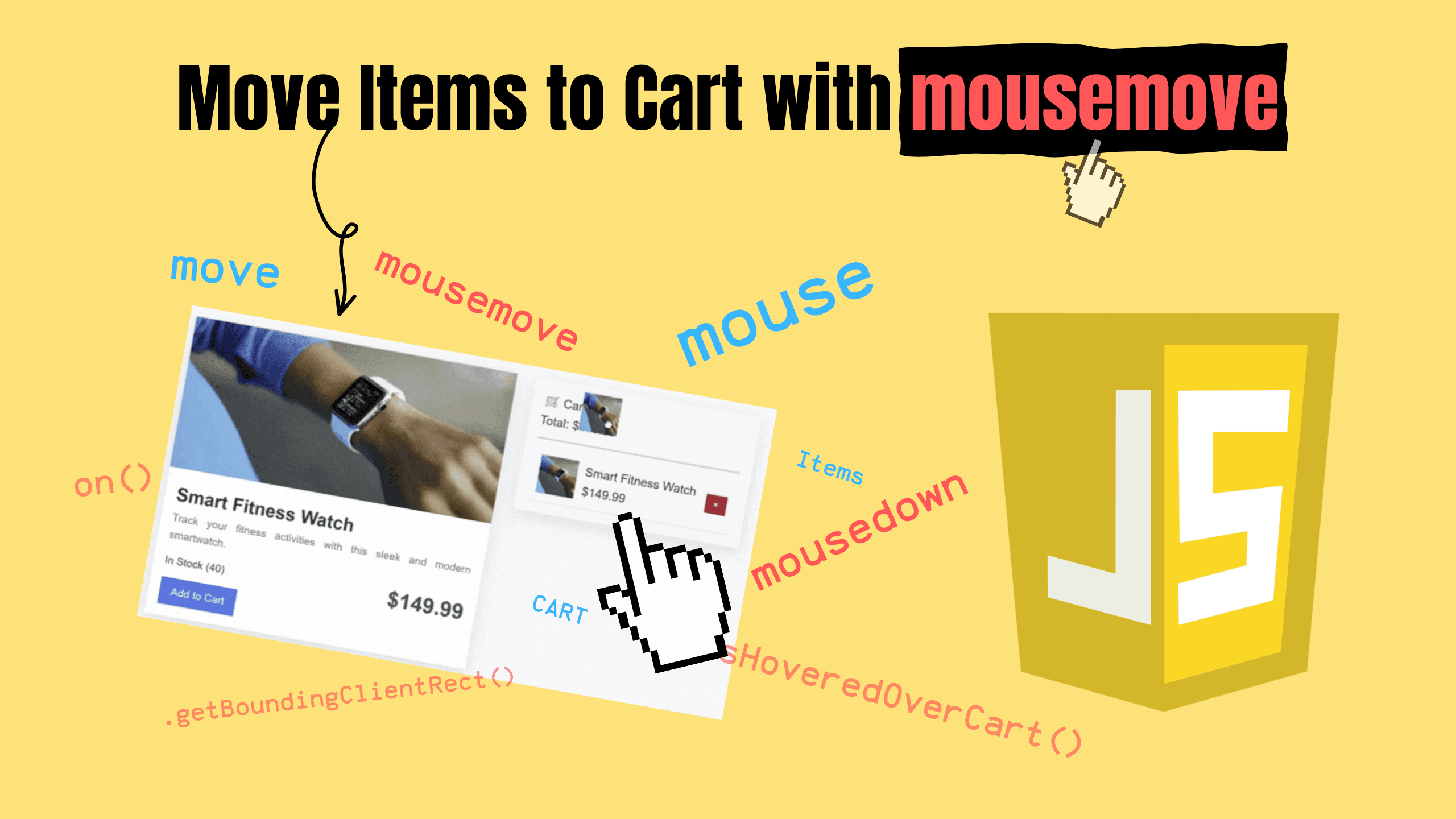
In modern web development, creating engaging user experiences is critical. One way to captivate your audience is by implementing dynamic interactions on your website. Imagine dragging a product image directly into the shopping cart—how cool and engaging does that sound?
In this tutorial, we’ll explore how to use JavaScript to visually move items to a shopping cart by tracking mouse movements (mousemove) and adding interactive drag-and-drop effects. We’ll break the project into manageable sections, walking through the code step by step.
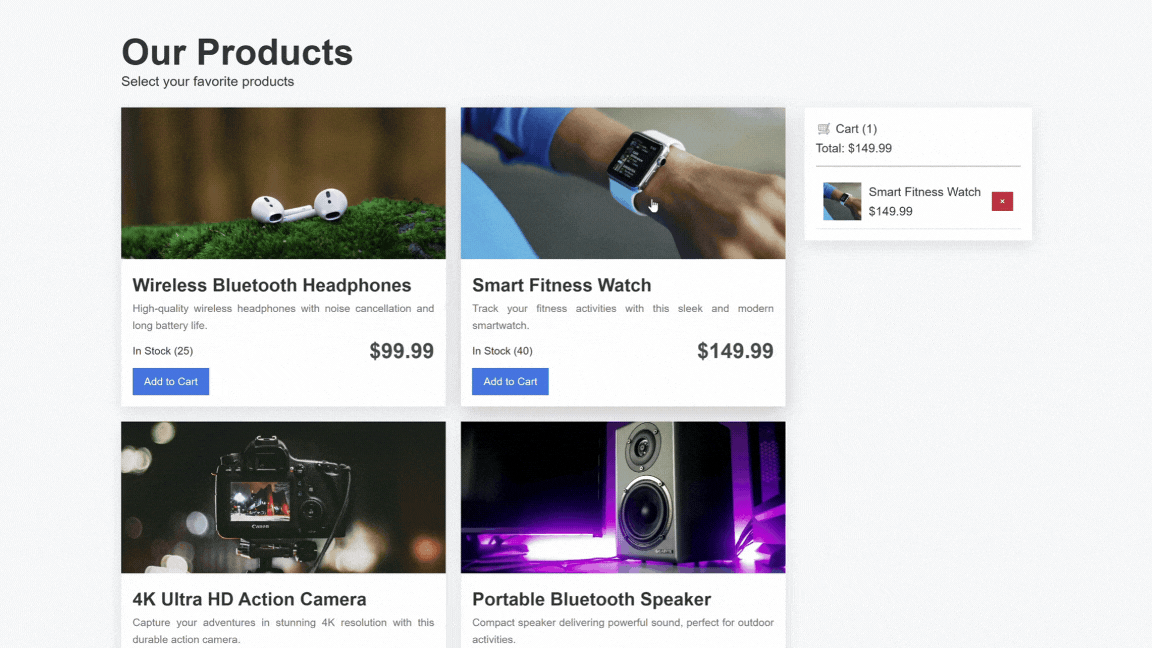
By the end, you’ll not only understand how the code works but also gain insights into how these techniques can enhance user engagement on your e-commerce websites.
Table of Contents
- Why Use Visual Interactions in E-Commerce?
- Tools and Technologies
- Overview of the Code Structure
- FAQs
- Conclusion
Why Use Visual Interactions in E-Commerce?
Online shoppers often abandon their carts due to lackluster user experiences. Adding visual interactions, like dragging a product into a cart, can boost engagement and reduce cart abandonment rates. It makes your website feel more interactive, professional, and even enjoyable to use.
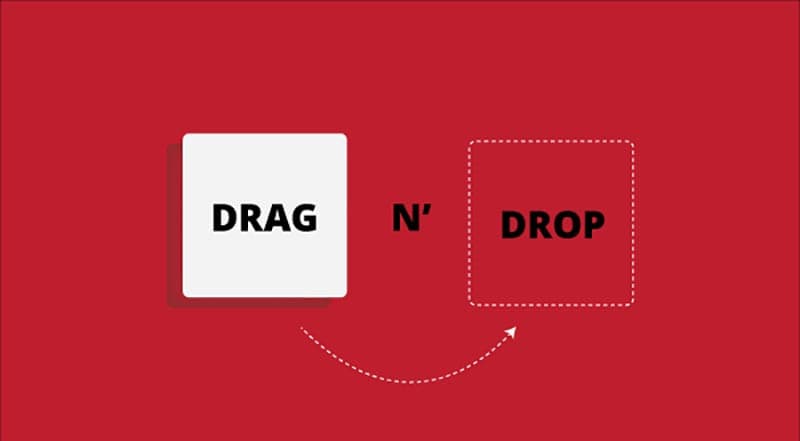
Psychological studies show tactile interactions increase user engagement by 40%. By allowing customers to "grab" products and see them move toward the cart, we tap into their spatial reasoning – making digital shopping feel more tangible.
This tutorial provides a reusable pattern that you can incorporate into e-commerce websites to elevate your user experience.
Tools and Technologies
Before diving into the code, here’s what we’re working with:
- HTML: Hypertext Markup Language (HTML) is the standard markup language for documents designed to be displayed in a web browser.
- CSS: Cascading Style Sheets (CSS) is a style sheet language used for specifying the presentation and styling of a document written in a markup language such as HTML or XML (including XML dialects such as SVG, MathML or XHTML).
- JavaScript: JavaScript (/ˈdʒɑːvəskrɪpt/), often abbreviated as JS, is a programming language and core technology of the Web, alongside HTML and CSS.
All components are built without any external libraries, ensuring maximum flexibility.
Overview of the Code Structure
This project has three key files:
- index.html: The main structure of the webpage, containing placeholders for products and the cart.
- styles.css: Defines the look and feel of the webpage.
- main.js: Implements interactivity, including the drag-and-drop effect.
Let’s take a closer look at each file.
1. HTML File (index.html)
The HTML file creates a clean, semantic foundation for the e-commerce interface. It features a responsive layout with a product grid (div id="products") dynamically populated via JavaScript and a sticky cart sidebar (aside id="cart") that remains visible during scrolling. The mobile-first approach shines through a hidden desktop cart and a fixed bottom mobile cart (div id="mobile-cart" ), ensuring usability across devices. Semantic tags like header, main, and footer improve accessibility, while empty containers like products-container and cart-items serve as injection points for JavaScript-generated content.
html<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <meta http-equiv="X-UA-Compatible" content="ie=edge" /> <title>Our Products</title> <link rel="stylesheet" href="styles.css" /> </head> <body> <header> <h1>Our Products</h1> <p class="subheader">Select your favorite products</p> <div id="mobile-cart" class="mobile-cart"> 🛒 <p>Cart (<span id="mobile-cart-count">0</span>)</p> </div> </header> <main class="main-container"> <!-- Products Section --> <div id="products" class="products-container"></div> <!-- Cart Section --> <aside id="cart" class="cart"> <div class="cart-header"> 🛒 Cart (<span id="cart-count">0</span>) <p>Total: <span id="cart-total">$0.00</span></p> </div> <div id="cart-items" class="cart-items"></div> </aside> </main> <footer> <p>© 2025 Our Products</p> </footer> <script src="./main.js"></script> </body> </html>
2. CSS File (styles.css)
The CSS stylesheet implements a modern aesthetic with card-based product displays, smooth hover animations, and a responsive grid that adapts column counts based on viewport width.
- Grid Layouts: A responsive grid for products.
- Hover Effects: Smooth hover transitions for product cards.
- Cart Styling: Fixed placement and clean UI for the shopping cart.
css/* General Reset */ * { margin: 0; padding: 0; box-sizing: border-box; } body { font-family: "Arial", sans-serif; background-color: #f7f8fa; color: #333; line-height: 1.6; padding: 10px; } header { max-width: 1200px; margin: 0 auto; margin-top: 20px; } h1 { margin: 0px; font-size: 48px; font-weight: bold; } a { text-decoration: none; color: #0073e6; } .subheader { font-size: 18px; color: #333; margin-top: -14px; } footer { text-align: center; padding: 10px; background-color: #505050; font-size: 14px; color: #d8d8d8; margin-top: 40px; } /* Main Layout */ .main-container { display: flex; flex-direction: row; justify-content: space-between; max-width: 1200px; gap: 25px; margin: 0 auto; margin-top: 20px; } /* Products Grid */ .products-container { display: grid; grid-template-columns: repeat(auto-fit, minmax(300px, 1fr)); gap: 20px; width: 100%; } /* Product Card */ .card { background-color: white; box-shadow: 0px 6px 20px rgba(0, 0, 0, 0.1); overflow: hidden; transition: transform 0.3s ease, box-shadow 0.3s ease; cursor: pointer; } .card:hover { box-shadow: 0px 10px 30px rgba(0, 0, 0, 0.2); } .card-image-container { height: 200px; overflow: hidden; } .card-image { width: 100%; height: 100%; object-fit: cover; transition: transform 0.3s ease; } .card:hover .card-image { transform: scale(1.1); } .card-content { padding: 15px; } .card-title { font-size: 24px; font-weight: bold; color: #333; } .card-description { font-size: 14px; color: #727272; text-align: justify; } .card-details { display: flex; justify-content: space-between; align-items: center; font-size: 14px; } .card-price { color: #484848; font-size: 28px; font-weight: bold; } .add-to-cart-btn { background-color: #0073e6; outline: none; color: white; border: none; padding: 10px 15px; font-size: 14px; cursor: pointer; transition: background 0.3s ease; } .add-to-cart-btn:hover { background-color: #005bb5; } /* Cart Section */ .cart { width: 100%; max-width: 300px; background: white; box-shadow: 0px 6px 20px rgba(0, 0, 0, 0.1); padding: 15px; display: flex; flex-direction: column; position: sticky; top: 20px; height: fit-content; } .cart-header { border-bottom: 2px solid #cdcdcd; padding-bottom: 10px; margin-bottom: 10px; } .cart-items { display: flex; flex-direction: column; gap: 10px; } .cart-item { display: flex; align-items: center; gap: 10px; padding: 10px; border-bottom: 1px solid #dcdcdc; } .cart-item-image { width: 50px; height: 50px; object-fit: cover; } .cart-item-details { flex-grow: 1; } .remove-cart-item { background: #dc3545; color: white; border: none; padding: 5px 10px; cursor: pointer; } .remove-cart-item:hover { background: #c82333; } /* Mobile Cart */ .mobile-cart { display: none; } .drag-image { position: absolute; z-index: 1000; width: 50px; height: 50px; } /* Responsive Design */ @media (max-width: 768px) { body { padding-bottom: 50px; } .main-container { flex-direction: column; } .cart { display: none; } .mobile-cart { display: block; position: fixed; bottom: 0; left: 0; right: 0; color: white; background: rgb(0, 0, 0); box-shadow: 0px -6px 20px rgba(0, 0, 0, 0.1); padding: 10px; display: flex; z-index: 100; justify-content: space-between; align-items: center; } header { display: flex; flex-direction: column; } header h1 { margin-bottom: 10px; } }
3. JavaScript File (main.js)
The JavaScript file drives all interactivity through three core systems:
- Product Rendering: Generates HTML cards from a product data array, complete with stock indicators and prices
- Drag Mechanics: Uses mousedown, mousemove, and mouseup events to create a "ghost image" drag effect, complete with cart boundary detection
- Cart Management: Maintains cart state, updates totals, and handles item removal via event delegation. Real-time UI synchronization ensures stock levels and cart counts always reflect current state, while the isHoveredOverCart() function uses getBoundingClientRect() for precise drag-and-drop targeting.
jsconst products = [ { name: "Wireless Bluetooth Headphones", description: "High-quality wireless headphones with noise cancellation and long battery life.", price: 99.99, stock: 25, image: "https://images.pexels.com/photos/1646704/pexels-photo-1646704.jpeg", }, { name: "Smart Fitness Watch", description: "Track your fitness activities with this sleek and modern smartwatch.", price: 149.99, stock: 40, image: "https://images.pexels.com/photos/267394/pexels-photo-267394.jpeg", }, { name: "4K Ultra HD Action Camera", description: "Capture your adventures in stunning 4K resolution with this durable action camera.", price: 199.99, stock: 15, image: "https://images.pexels.com/photos/243757/pexels-photo-243757.jpeg", }, { name: "Portable Bluetooth Speaker", description: "Compact speaker delivering powerful sound, perfect for outdoor activities.", price: 59.99, stock: 30, image: "https://images.pexels.com/photos/2651794/pexels-photo-2651794.jpeg", }, { name: "Ergonomic Office Chair", description: "Comfortable office chair with adjustable settings to support long working hours.", price: 249.99, stock: 10, image: "https://images.pexels.com/photos/245032/pexels-photo-245032.jpeg", }, { name: "Electric Toothbrush", description: "Advanced electric toothbrush with multiple cleaning modes and a long-lasting battery.", price: 79.99, stock: 50, image: "https://images.pexels.com/photos/298611/pexels-photo-298611.jpeg", }, { name: "Stainless Steel Water Bottle", description: "Insulated water bottle that keeps your drinks hot or cold for hours.", price: 29.99, stock: 100, image: "https://images.pexels.com/photos/416528/pexels-photo-416528.jpeg", }, { name: "Noise-Cancelling Earbuds", description: "Compact earbuds with active noise cancellation and superior sound quality.", price: 129.99, stock: 35, image: "https://images.pexels.com/photos/373945/pexels-photo-373945.jpeg", }, { name: "Smart Home Security Camera", description: "Monitor your home remotely with this high-definition smart security camera.", price: 89.99, stock: 20, image: "https://images.pexels.com/photos/21264/pexels-photo.jpg", }, { name: "Digital Kitchen Scale", description: "Precise kitchen scale for accurate measurements, essential for every home cook.", price: 24.99, stock: 60, image: "https://images.pexels.com/photos/102104/pexels-photo-102104.jpeg", }, ]; const productsDom = document.getElementById("products"); const cartDiv = document.getElementById("cart"); const cartDom = document.getElementById("cart-items"); const cartCount = document.getElementById("cart-count"); const cartTotal = document.getElementById("cart-total"); let cart = []; let isMouseDown = false; let dragImage = null; let selectedProduct = null; // Render products productsDom.innerHTML = ""; products.forEach((product) => { const card = document.createElement("div"); card.className = "card"; card.setAttribute("id", product.name); card.innerHTML = ` <div class="card-image-container"> <img src="${product.image}" alt="${product.name}" class="card-image" /> </div> <div class="card-content"> <h2 class="card-title">${product.name}</h2> <p class="card-description">${product.description}</p> <div class="card-details"> <span class="card-stock">${ product.stock > 0 ? `In Stock (${product.stock})` : "Out of Stock" }</span> <span class="card-price">$${product.price.toFixed(2)}</span> </div> <button class="add-to-cart-btn">Add to Cart</button> </div> `; // Add to cart button click card .querySelector(".add-to-cart-btn") .addEventListener("click", () => addToCart(product)); // Attach mouse events for dragging the image const image = card.querySelector(".card-image"); // Mouse down event to start dragging image.addEventListener("mousedown", (e) => { e.preventDefault(); isMouseDown = true; selectedProduct = product; if (dragImage) { document.body.removeChild(dragImage); } dragImage = document.createElement("img"); dragImage.className = "drag-image"; dragImage.src = product.image; dragImage.style.left = `${e.clientX + window.scrollX - 25}px`; dragImage.style.top = `${e.clientY + window.scrollY - 25}px`; document.body.appendChild(dragImage); document.body.style.cursor = "grabbing"; }); productsDom.append(card); }); // Mouse move event to update position of the image document.addEventListener("mousemove", (e) => { if (isMouseDown && dragImage) { dragImage.style.left = `${e.clientX + window.scrollX - 25}px`; dragImage.style.top = `${e.clientY + window.scrollY - 25}px`; if (isHoveredOverCart(e)) { cartDiv.style.backgroundColor = "#f7f7f7"; } else { cartDiv.style.backgroundColor = "white"; } } }); // Mouse up event to end dragging document.addEventListener("mouseup", (e) => { if (isMouseDown && dragImage) { isMouseDown = false; document.body.removeChild(dragImage); dragImage = null; if (isHoveredOverCart(e)) { addToCart(selectedProduct); selectedProduct.stock--; // Decrease stock when added to the cart } cartDiv.style.backgroundColor = "white"; document.body.style.cursor = "auto"; } }); // Check if mouse is over cart function isHoveredOverCart(e) { const cartRect = cartDiv.getBoundingClientRect(); return ( cartRect.left < e.clientX && e.clientX < cartRect.right && cartRect.top < e.clientY && e.clientY < cartRect.bottom ); } // Add to cart function addToCart(product) { if (product.stock > 0) { cart.push(product); updateCartUI(); } } // Update cart UI function updateCartUI() { cartDom.innerHTML = ""; let total = 0; cart.forEach((item, index) => { total += item.price; const cartItem = document.createElement("div"); cartItem.className = "cart-item"; cartItem.innerHTML = ` <img src="${item.image}" alt="${item.name}" class="cart-item-image" /> <div class="cart-item-details"> <p class="cart-item-name">${item.name}</p> <p class="cart-item-price">$${item.price.toFixed(2)}</p> </div> <button class="remove-cart-item" data-index="${index}">×</button> `; cartItem .querySelector(".remove-cart-item") .addEventListener("click", () => { cart.splice(index, 1); updateCartUI(); }); cartDom.append(cartItem); }); cartCount.textContent = cart.length; cartTotal.textContent = `$${total.toFixed(2)}`; }
Step-by-Step Code Breakdown
1. Rendering Products Dynamically
Products are stored as an array of objects. Each object includes properties like name, price, stock, and image. Using forEach, each product is converted into a card and added to the DOM:
javascriptproducts.forEach((product) => { const card = document.createElement("div"); card.className = "card"; card.innerHTML = ` <div class="card-image-container"> <img src="${product.image}" class="card-image" /> </div> <h2>${product.name}</h2> <p>${product.description}</p> <span>$${product.price.toFixed(2)}</span> <button>Add to Cart</button>`; productsDom.append(card); });
This approach ensures scalability and avoids hardcoding products.
2. Handling Drag-and-Drop Interactions
The mousedown event initiates the dragging behavior when the user clicks on a product image. It sets isMouseDown to true, identifies the selected product, and creates a new dragImage element that visually follows the cursor. This event also styles the cursor as "grabbing" to enhance interactivity.
javascriptimage.addEventListener("mousedown", (e) => { e.preventDefault(); isMouseDown = true; selectedProduct = product; if (dragImage) { document.body.removeChild(dragImage); } dragImage = document.createElement("img"); dragImage.className = "drag-image"; dragImage.src = product.image; dragImage.style.left = `${e.clientX + window.scrollX - 25}px`; dragImage.style.top = `${e.clientY + window.scrollY - 25}px`; document.body.appendChild(dragImage); document.body.style.cursor = "grabbing"; });
The mousemove event is triggered whenever the mouse pointer moves. In this implementation, it updates the position of the dragImage dynamically as the user drags a product image across the screen. The event ensures smooth and precise movement by setting the left and top styles of the dragImage based on the cursor's current position (e.clientX and e.clientY).
javascriptdocument.addEventListener("mousemove", (e) => { if (isMouseDown && dragImage) { dragImage.style.left = `${e.clientX + window.scrollX - 25}px`; dragImage.style.top = `${e.clientY + window.scrollY - 25}px`; if (isHoveredOverCart(e)) { cartDiv.style.backgroundColor = "#f7f7f7"; } else { cartDiv.style.backgroundColor = "white"; } } });
Dropping into the Cart on mouseup, if the item is hovered over the cart, it is added to the cart array, and the UI is updated.
3. Updating the Cart in Real-Time
The cart maintains a global cart array. Adding an item involves:
- Pushing the product into the array.
- Rendering the updated list:
javascriptcart.forEach((item) => { const cartItem = document.createElement("div"); cartItem.innerHTML = ` <p>${item.name}</p> <span>$${item.price}</span>`; cartDom.append(cartItem); });
This real-time update makes the experience seamless.
FAQs
- Why use mousemove instead of the HTML5 Drag-and-Drop API? The HTML5 Drag-and-Drop API has limited customization for visual effects (e.g., ghost images, animations). Using mousemove with JavaScript grants full control over the dragged element’s appearance, trajectory, and cart interaction logic. This approach allows smoother animations and custom hover feedback, as demonstrated in the code’s dynamic image positioning.
- How does the code handle mobile responsiveness? The CSS media queries hide the desktop cart on screens ≤768px and display a fixed bottom mobile cart. However, the current implementation uses mouse events (mousedown, mousemove), which don’t work on touch devices. To fix this, you’d need to add touchstart, touchmove, and touchend event listeners alongside mouse events for cross-device compatibility.
- Why does the product stock not update visually after adding to the cart? The code decrements selectedProduct.stock during the mouseup event (see selectedProduct.stock--), but the UI isn’t automatically refreshed. To update stock displays, add logic in the addToCart function to re-render affected product cards or modify the DOM directly using
jsdocument.getElementById(product.name).querySelector('.card-stock').textContent.
- How can I add animations when items are removed from the cart? The current code removes items instantly via cart.splice(index, 1). To animate removals, apply CSS transitions to the .cart-item class (e.g., transition: opacity 0.3s) and use JavaScript to toggle a fading class before splicing the array:
jscartItem.classList.add('fade-out'); setTimeout(() => cart.splice(index, 1), 300);
- Is this implementation accessible for screen readers? As written, the drag interaction lacks ARIA labels and keyboard support. To improve accessibility:
- Add role="button" and aria-label="Add to cart" to draggable elements.
- Implement keyboard event listeners (e.g., keydown on Enter/Spacebar) to trigger cart additions.
- Use aria-live regions to announce cart updates dynamically.
Conclusion
With just HTML, CSS, and JavaScript, you can create an interactive e-commerce experience. This tutorial not only teaches technical implementation but also inspires you to think creatively about user engagement.
Take this concept further by integrating it with a backend to handle inventory or user authentication!
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!