Learn addEventListener and removeEventListener in JavaScript
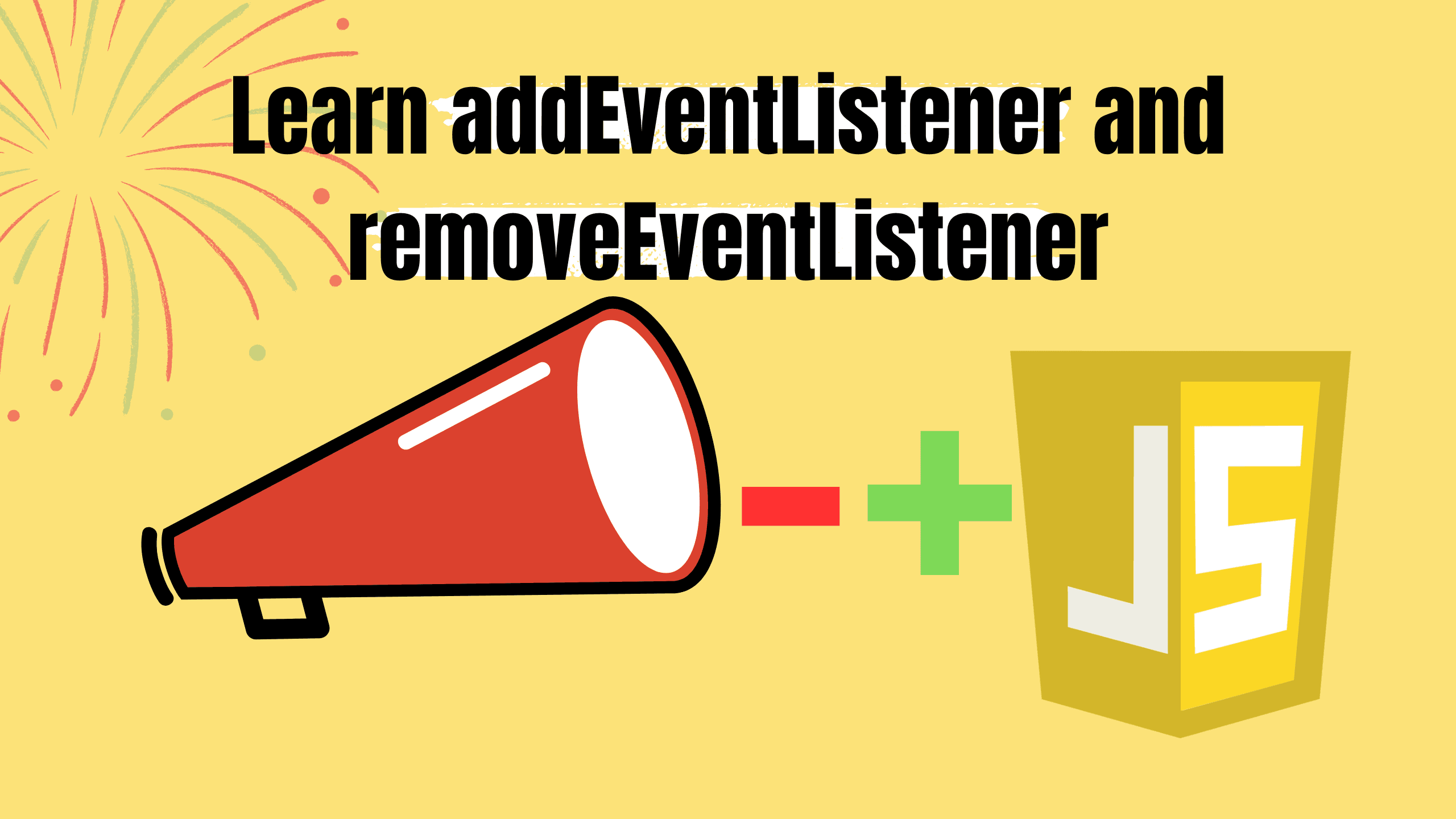
JavaScript is a versatile and dynamic programming language that allows to create interactive and responsive web applications. One of its core features is event handling, which enables to listen for and respond to user actions such as clicks, key presses, and mouse movements. Efficient event handling is crucial for ensuring a seamless user experience, reducing unnecessary computations, and maintaining performance across complex web applications.
Among the various event-handling techniques available in JavaScript, addEventListener and removeEventListener play a pivotal role in managing event-driven interactions. These methods provide a structured and flexible approach to adding and removing event listeners, allowing to create modular, maintainable, and efficient code. In this blog, we will dive deep into these essential methods, explore their syntax, understand their best use cases, and highlight potential pitfalls to avoid when working with event listeners.
What is addEventListener?
The addEventListener method enables to attach multiple event handlers to an element without overwriting existing ones. This approach provides greater flexibility and control over event management compared to inline event handlers like onclick, allowing for cleaner and more modular code.
Syntax of addEventListener:
The addEventListener method follows a specific structure that allows to define what event to listen for, which function should handle it, and optionally, whether the event should be captured in a specific phase.
jselement.addEventListener(event, function, useCapture);
- event: A string representing the event type (e.g., click, mouseover, keydown).
- function: The function that will be executed when the event occurs.
- useCapture (optional): A boolean value determining whether the event should be captured during the capture phase (true) or the bubbling phase (false, default).
Example of addEventListener:
Understanding how to properly use addEventListener is crucial for managing user interactions effectively. Look at example:
javascript// Select the button element const button = document.getElementById("myButton"); // Add a click event listener button.addEventListener("click", function() { alert("Button Clicked!"); });
Phases of Event Propagation
JavaScript events follow a propagation model that consists of 3 phases. This model determines how events travel through the DOM, allowing to control where and when an event handler executes.
- Capturing Phase: The event starts at the root of the document and moves down through the DOM hierarchy to the target element. During this phase, parent elements receive the event before it reaches the intended target.
- Target Phase: The event reaches the actual element that triggered it. At this stage, only the event listener attached directly to the target element executes.
- Bubbling Phase: After reaching the target element, the event travels back up the DOM tree toward the root. Any parent elements with an event listener for the same event type will execute their handlers, unless event propagation is stopped.
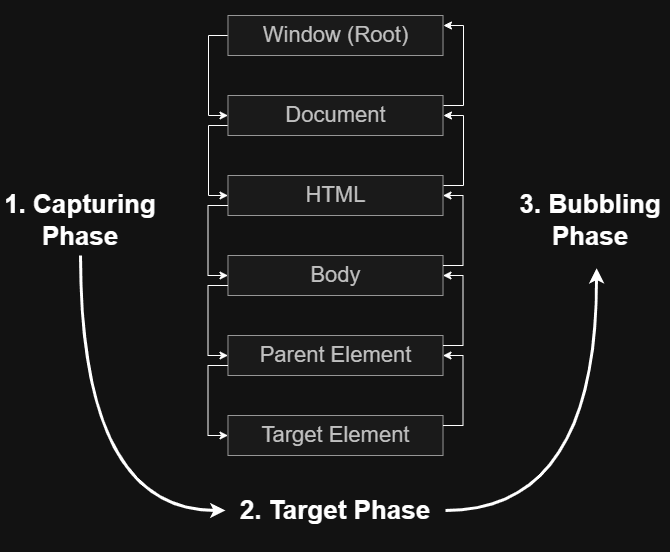
By default, addEventListener listens in the bubbling phase, meaning the event is handled after it reaches the target and starts traveling back up. However, by setting the third parameter of addEventListener to true, you can make it listen in the capturing phase instead, allowing parent elements to handle the event before it reaches the target. Understanding event propagation is essential for implementing event delegation, preventing unnecessary event handling, and optimizing performance in web applications.
Example of Capturing vs. Bubbling:
In JavaScript, event propagation follows two paths: capturing and bubbling. Capturing moves from the top of the DOM tree to the target element, while bubbling moves from the target back up. Choosing the right propagation method helps in better event handling.
javascriptdocument.getElementById("parent").addEventListener("click", () => { console.log("Parent clicked (bubbling phase)"); }, false); document.getElementById("child").addEventListener("click", () => { console.log("Child clicked"); }, false);
Advantages of addEventListener
- Allows multiple event listeners: Unlike onclick, which replaces the existing event handler, addEventListener enables multiple functions to run on the same event.
- Event delegation: Events can be handled at a parent level instead of individual child elements, improving performance and reducing redundant event bindings.
- Separation of concerns: Keeps JavaScript separate from HTML, making code cleaner, more structured, and maintainable.
- Works with third-party scripts: Ensures compatibility with other scripts modifying event listeners.
- Supports additional event options: With addEventListener, you can specify options such as once (ensures the handler runs only once) and passive (optimizes scrolling events).
javascript
button.addEventListener("click", function() { alert("This will run only once"); }, { once: true });
What is removeEventListener?
The removeEventListener method detaches an event listener that was previously added using addEventListener. This helps in managing event handlers effectively, preventing memory leaks, and enhancing application performance by ensuring that unused listeners do not persist unnecessarily.
Syntax of removeEventListener:
The removeEventListener method follows a similar structure to addEventListener, requiring the exact event type, function reference, and optional useCapture parameter to remove an event listener successfully.
jselement.removeEventListener(event, function, useCapture);
- event: A string representing the event type (e.g., click, mouseover, keydown).
- function: The function that will be executed when the event occurs.
- useCapture (optional): A boolean value determining whether the event should be captured during the capture phase (true) or the bubbling phase (false, default).
Example of removeEventListener:
Removing event listeners helps in preventing memory leaks and improving performance. Below is an example demonstrating how to properly remove an event listener.
javascriptfunction handleClick() { alert("Button Clicked!"); } const button = document.getElementById("myButton"); button.addEventListener("click", handleClick); // Remove the event listener after 5 seconds setTimeout(() => { button.removeEventListener("click", handleClick); }, 5000);
Key Considerations When Using removeEventListener
- Function reference must match: If an anonymous function was used in addEventListener, it cannot be removed because removeEventListener requires the exact function reference.
- Matching useCapture value: If true was used in addEventListener, the same must be used in removeEventListener.
- Memory leaks: Always remove event listeners for dynamically created elements when they are no longer needed to avoid memory leaks and improve performance.
- Avoid unnecessary removals: Ensure that you remove event listeners only when required, as removing event listeners too aggressively can impact application behavior.
Event Delegation Using addEventListener
Event delegation is a powerful pattern in JavaScript where a parent element listens for events on its child elements instead of attaching individual event listeners to each child. This is particularly useful when working with dynamically created elements, as it ensures that even newly added elements can respond to events without requiring additional event bindings.
The way event delegation works is by leveraging event bubbling. When an event occurs on a child element, it propagates up through the DOM hierarchy, allowing an event listener attached to a common ancestor to detect and handle the event. This technique reduces the number of event listeners needed, optimizing memory usage and improving performance.
Using event delegation is advantageous in situations where elements are frequently added or removed from the DOM, such as lists, tables, and interactive components. It simplifies event management, avoids redundant event handlers, and ensures that events are handled efficiently without unnecessary memory consumption.
javascriptdocument.getElementById("list").addEventListener("click", function(event) { if (event.target.tagName === "LI") { alert("You clicked on " + event.target.textContent); } });
Common Mistakes
Making mistakes while using event listeners can lead to performance issues, memory leaks, and unintended behavior in your applications. Here are some common mistakes and how to avoid them.
1: Not Using Named Functions for Removal
One common mistake is using anonymous functions when adding and removing event listeners. Since removeEventListener requires an exact reference to the function, an anonymous function cannot be removed because it is treated as a new function each time.
javascriptbutton.addEventListener("click", function() { console.log("Clicked!"); }); button.removeEventListener("click", function() { console.log("Clicked!"); }); // Will not work
Using named functions ensures that event listeners can be added and removed properly, preventing unexpected behavior and memory leaks.
javascriptfunction handleClick() { console.log("Clicked!"); } button.addEventListener("click", handleClick); button.removeEventListener("click", handleClick);
2: Forgetting to Remove Listeners in Single-Use Scenarios
If an event listener is only needed once, failing to remove it can lead to unnecessary memory usage. Instead of manually removing it, using once: true ensures that the event handler is automatically removed after execution.
javascriptbutton.addEventListener("click", () => console.log("Clicked!"), { once: true });
3: Using Too Many Event Listeners
Adding event listeners to multiple child elements can be inefficient, leading to performance issues. Instead, use event delegation by attaching a single listener to a parent element and handling events for child elements dynamically.
javascriptdocument.getElementById("parent").addEventListener("click", function(event) { if (event.target.matches(".child")) { console.log("Child clicked"); } });
Conclusion
Understanding addEventListener and removeEventListener is crucial for effective event management in JavaScript. These methods provide flexibility, efficiency, and better maintainability for modern web applications. Always remember that addEventListener allows for multiple event handlers on the same element without interference, making it a versatile and essential tool for event handling.
Additionally, always ensure that unnecessary event listeners are removed using removeEventListener to prevent memory leaks and optimize performance. Using event delegation is an effective strategy for handling dynamically generated elements, reducing redundant event bindings, and improving overall application efficiency. Furthermore, when removing event listeners, always provide the exact function reference to ensure proper detachment and avoid unexpected behaviors.
By mastering these concepts, you can write cleaner, more efficient, and scalable JavaScript applications, ensuring a smooth user experience and improved performance across complex web applications.
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!