Best JavaScript Guide: Add or Remove Style Dynamically
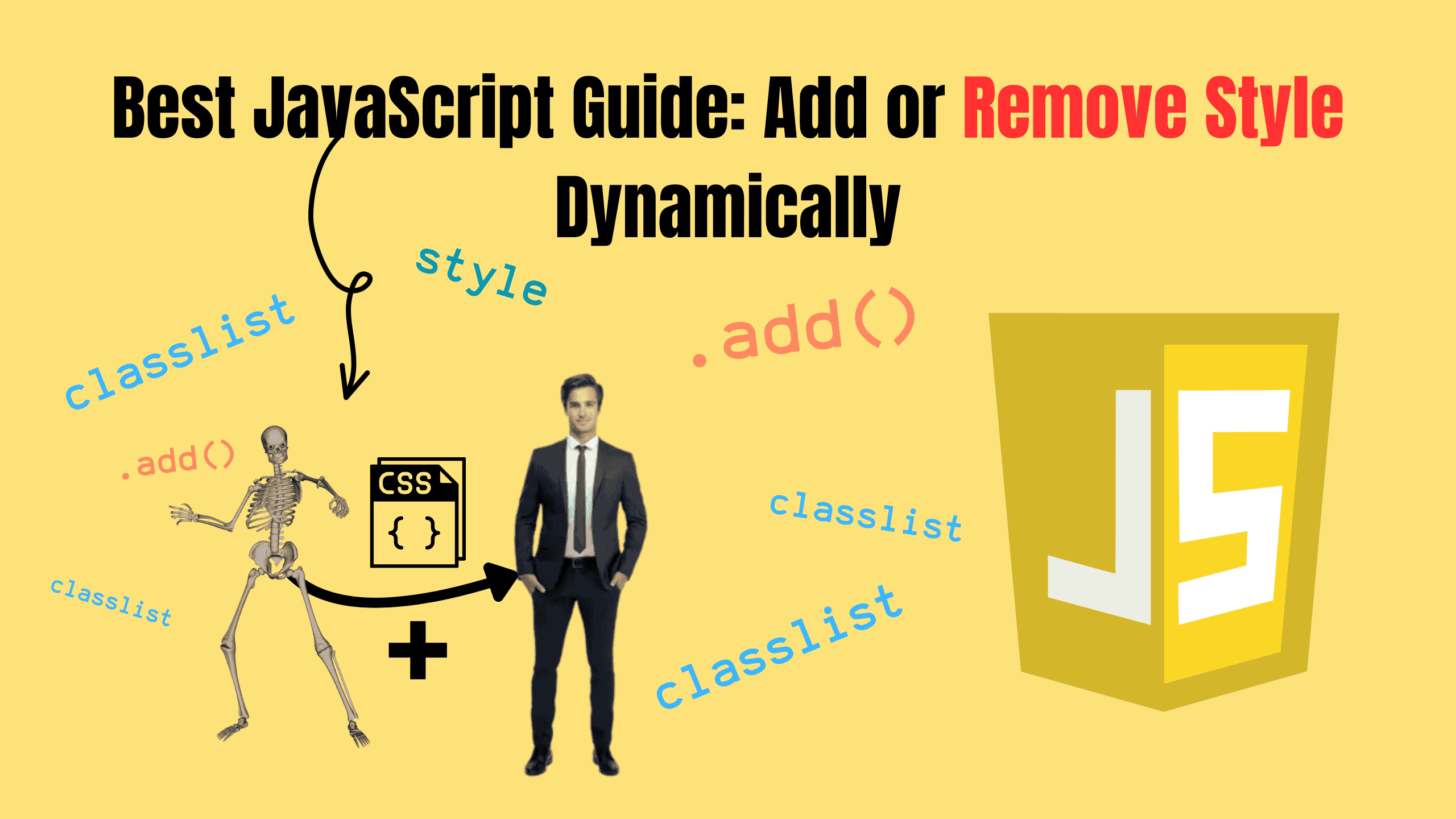
JavaScript is an incredibly versatile language, and one of its powerful features is its ability to manipulate the Document Object Model (DOM) to create dynamic and interactive web pages. Among the many tasks JavaScript can handle, adding or removing style dynamically is a fundamental yet essential technique that developers frequently use. In this blog, we’ll explore how to add or remove styles in JavaScript, with examples and best practices.
What Are Styles in Web Development?
Styles control the visual presentation of your web page. Typically, these styles are defined in a Cascading Style Sheets (CSS) file. However, there are scenarios where you may need to alter styles dynamically, such as: Highlighting a menu item on hover.
- Showing or hiding elements based on user interactions.
- Implementing dark mode toggles.
JavaScript enables developers to manipulate styles at runtime, offering immense flexibility in creating engaging user experiences.
Methods to Add or Remove Style in JavaScript
There are several ways to add or remove styles using JavaScript. Let’s break them down into categories for better understanding:
1. Using Inline Styles
Inline styles are directly added to an element using the style property. This approach is straightforward as it allows you to apply specific styles directly to an element without relying on external or internal stylesheets. It is especially useful for quick and isolated changes, such as temporarily highlighting a field in response to user interaction.
However, overusing inline styles can lead to cluttered and hard-to-maintain code, as they mix presentation with logic and do not leverage the reusability and organization benefits of CSS classes or stylesheets.
Adding a Style:
jsconst element = document.getElementById('myElement'); element.style.color = 'blue'; // Changes text color to blue
Removing a Style:
jsconst element = document.getElementById('myElement'); element.style.color = ''; // Resets to the default or inherited value
Inline styles are quick to implement and useful for single-use scenarios but can make the code harder to maintain if overused.
2. Using the classList Property
A more structured and reusable way to add or remove styles is by manipulating CSS classes using the classList property. This method promotes clean separation of concerns by keeping styles defined in CSS files, ensuring a clear distinction between styling and functionality. It also allows for easy reuse of styles across multiple elements, making your code more modular and maintainable.
By utilizing the classList property, you can add, remove, or toggle predefined classes dynamically, enabling responsive and interactive web designs with minimal effort. This approach is not only cleaner but also adheres to best practices in web development.
Adding a Class:
jsconst element = document.getElementById('myElement'); element.classList.add('highlight'); // Adds the class 'highlight'
Removing a Class:
jsconst element = document.getElementById('myElement'); element.classList.remove('highlight'); // Removes the class 'highlight'
Toggling a Class:
jsconst element = document.getElementById('myElement'); element.classList.toggle('highlight'); // Adds if not present, removes if present
Checking if a Class Exists:
jsif (element.classList.contains('highlight')) { console.log('Class exists!'); }
Using classList is ideal for managing styles systematically, keeping your code clean and your CSS reusable.
3. Using the setAttribute and removeAttribute Methods
This method manipulates the style attribute directly to add or remove styles. Using setAttribute, you can apply multiple styles at once, which is helpful for quick changes. However, it overwrites all existing inline styles, so it must be used carefully to avoid losing other styles.
Conversely, removeAttribute clears the style attribute entirely, resetting the element's appearance to inherited or stylesheet-defined styles. While simple, these methods are less flexible than classList, which keeps styles modular and easier to maintain. Use direct style manipulation sparingly for precise control when needed.
Adding Styles:
jsconst element = document.getElementById('myElement'); element.setAttribute('style', 'color: blue; background-color: yellow;');
Removing the Style Attribute:
jsconst element = document.getElementById('myElement'); element.removeAttribute('style');
This approach is helpful when applying multiple inline styles at once but should be used sparingly to avoid overriding existing styles.
4. Using External or Internal Stylesheets Dynamically
In some cases, you may need to dynamically load or modify stylesheets using JavaScript to enhance user experiences or implement theming functionality. Dynamically adding a new stylesheet allows you to apply a fresh set of styles without refreshing the page. This is particularly useful for enabling features like dark mode or applying user-specific preferences.
You can also modify existing stylesheets by adding or editing rules programmatically, giving you precise control over the design. For example, inserting a rule dynamically can immediately impact all matching elements, which is an efficient way to implement global style changes.
Adding a New Stylesheet:
jsconst link = document.createElement('link'); link.rel = 'stylesheet'; link.href = 'styles.css'; document.head.appendChild(link);
Modifying Existing Rules:
jsconst stylesheet = document.styleSheets[0]; stylesheet.insertRule('body { background-color: lightgray; }', stylesheet.cssRules.length);
This method allows global style changes and is ideal for advanced theming requirements or dynamic styling.
Using Local Storage to Persist Styles (Bonus)
To enhance user experience further, you can use localStorage to remember style preferences, such as dark mode or custom themes. By saving these preferences locally, you ensure they persist across page reloads or even future visits to the site.
For instance, storing a "dark" or "light" theme in localStorage allows users to return to their preferred setting effortlessly. This creates a smoother and more personalized browsing experience, fostering user satisfaction and engagement.
Saving a Style Preference:
jslocalStorage.setItem('theme', 'dark');
Applying the Saved Preference:
jsconst savedTheme = localStorage.getItem('theme'); if (savedTheme) { document.body.classList.add(savedTheme); // Apply the saved theme }
Clearing the Preference:
jslocalStorage.removeItem('theme');
Using localStorage in combination with classList allows for a seamless and persistent styling experience for users.
Best Practices for Adding or Removing Styles
- Prefer classList Over Inline Styles: Using classList helps separate concerns by keeping CSS in dedicated stylesheets, making the code more maintainable and readable.
- Minimize Inline Styles: Use inline styles sparingly as they can lead to code that is harder to debug and maintain.
- Use CSS Variables: For theming or dynamic style changes, consider using CSS variables along with JavaScript to toggle themes efficiently.
jsdocument.documentElement.style.setProperty('--main-color', 'blue');
- Optimize Performance: Avoid excessive DOM manipulation as it can degrade performance, especially for complex or frequently updated elements.
- Use Event Delegation: When adding or removing styles in response to events, use event delegation to improve efficiency:
jsdocument.addEventListener('click', (event) => { if (event.target.matches('.clickable')) { event.target.classList.toggle('active'); } });
Frequently Asked Questions (FAQs)
- What is the difference between classList and style? classList is used to add, remove, or toggle CSS classes, which are pre-defined in a stylesheet. The style property is used to apply inline styles directly to an element.
- When should I use inline styles? Inline styles should be used for quick, one-off changes or when dynamically applying styles that are not pre-defined in CSS. However, it’s generally better to use CSS classes for maintainability.
- Can I add multiple classes at once using classList? Yes, you can add multiple classes by passing them as separate arguments to the add method:
jselement.classList.add('class1', 'class2');
- How can I dynamically change the CSS file of a webpage? You can dynamically load a new CSS file by creating a link element and appending it to the head of the document:
jsconst link = document.createElement('link'); link.rel = 'stylesheet'; link.href = 'new-styles.css'; document.head.appendChild(link);
- How do I check if an element has a specific style? To check if an element has a specific style applied, you can use the getComputedStyle method:
jsconst style = window.getComputedStyle(element); console.log(style.color); // Outputs the computed color value
Conclusion
Manipulating styles dynamically with JavaScript is an indispensable skill for web developers. Whether you’re adding styles inline, toggling CSS classes, or modifying stylesheets, JavaScript provides a variety of methods to achieve the desired results. Adhering to best practices and leveraging the right approach for your use case can help you create responsive, dynamic, and visually appealing web applications. Experiment with the methods discussed in this guide to unlock JavaScript's potential to enhance your web designs.
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!