7 Single-Line Helpful Functions in JavaScript
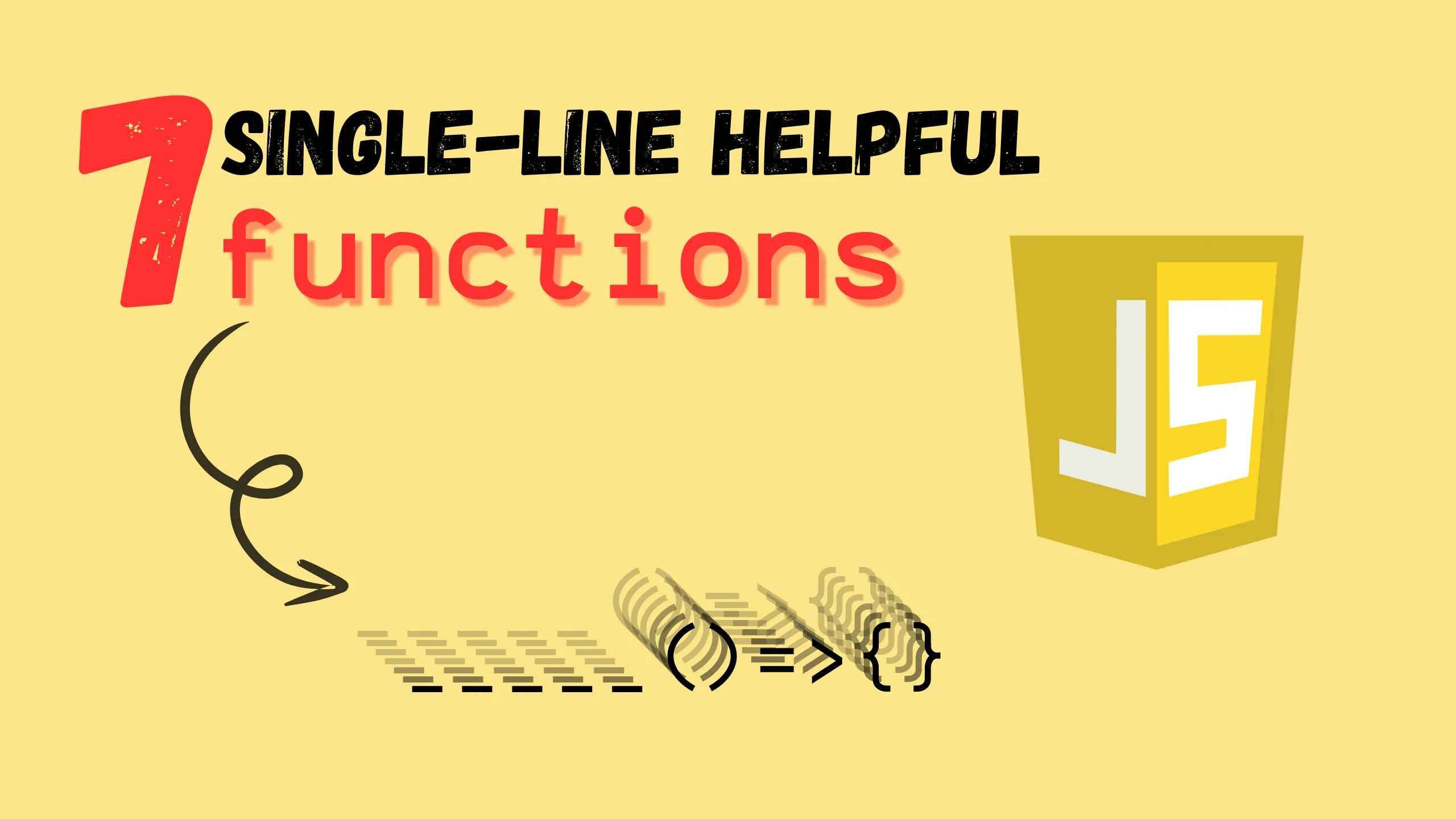
Ahh, Only 7 single-line functions will boost your development. JavaScript often requires more lines of code than necessary for common tasks. Sometimes, a single line is all you need to do the job efficiently.
Here are seven single-line functions that simplify your code and simplify your life.
1. Array Deduplication
Remove duplicates from an array using the Set object, which only stores unique values.
jsconst uniqueArray = arr => [...new Set(arr)];
Example: This function converts the array into a Set to remove duplicates and then back into an array.
jsconst numbers = [1, 2, 2, 3, 4, 4]; console.log(uniqueArray(numbers)); // [1, 2, 3, 4]
2. Check for Empty Object
Quickly check if an object is empty (i.e., has no own properties).
jsconst isEmptyObject = obj => Object.keys(obj).length === 0;
Example: This function uses Object.keys() to get an array of the object's own enumerable properties and check if its length is zero.
jsconst obj = {}; console.log(isEmptyObject(obj)); // true
3. Get a Random Element from the Array
Retrieve a random element from an array with a simple one-liner.
jsconst getRandomElement = arr => arr[Math.floor(Math.random() * arr.length)];
Example: This function calculates a random index and returns the element at that index in the array.
jsconst colors = ['red', 'green', 'blue']; console.log(getRandomElement(colors)); // e.g., 'green'
4. Check if the Array Contains Value
Determine if an array contains a specific value using includes.
jsconst arrayContains = (arr, value) => arr.includes(value);
Example: This function checks if the value exists in the array and returns a boolean result.
jsconst fruits = ['apple', 'banana', 'cherry']; console.log(arrayContains(fruits, 'banana')); // true
5. Convert String to Title Case
Convert a string to a title case where each word is capitalized.
jsconst toTitleCase = str => str.replace(/\b\w/g, char => char.toUpperCase());
Example: This function capitalizes the first letter of each word in the string using a regular expression.
jsconst title = 'hello world'; console.log(toTitleCase(title)); // 'Hello World'
6. Flatten Nested Arrays
Flatten a deeply nested array into a single-level array.
jsconst flattenArray = arr => arr.flat(Infinity);
Example: This function uses the flat() method with Infinity as the depth argument to handle any level of nesting.
jsconst nestedArray = [1, [2, [3, [4, [5]]]]]; console.log(flattenArray(nestedArray)); // [1, 2, 3, 4, 5]
7. Deep Merge Two Objects
Merge two objects deeply, combining properties recursively.
jsconst deepMerge = (obj1, obj2) => Object.assign({}, obj1, Object.keys(obj2).reduce((acc, key) => ({ ...acc, [key]: (typeof obj2[key] === 'object' && obj2[key] !== null && obj1[key] && typeof obj1[key] === 'object') ? deepMerge(obj1[key], obj2[key]) : obj2[key] }), {}));
Example: This function recursively merges two objects, combining properties from both objects while handling nested objects properly. It uses Object.assign() and reduce() to achieve a deep merge.
jsconst obj1 = { a: 1, b: { c: 2, d: 3 } }; const obj2 = { b: { d: 4, e: 5 }, f: 6 }; console.log(deepMerge(obj1, obj2)); // { a: 1, b: { c: 2, d: 4, e: 5 }, f: 6 }
Conclusion
Single-line functions can simplify and streamline your JavaScript code, making it both elegant and efficient. By leveraging concise patterns for common tasks, you enhance readability and maintainability, proving that less can indeed be more in coding.
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!