Removing the Last Character from Strings in JavaScript
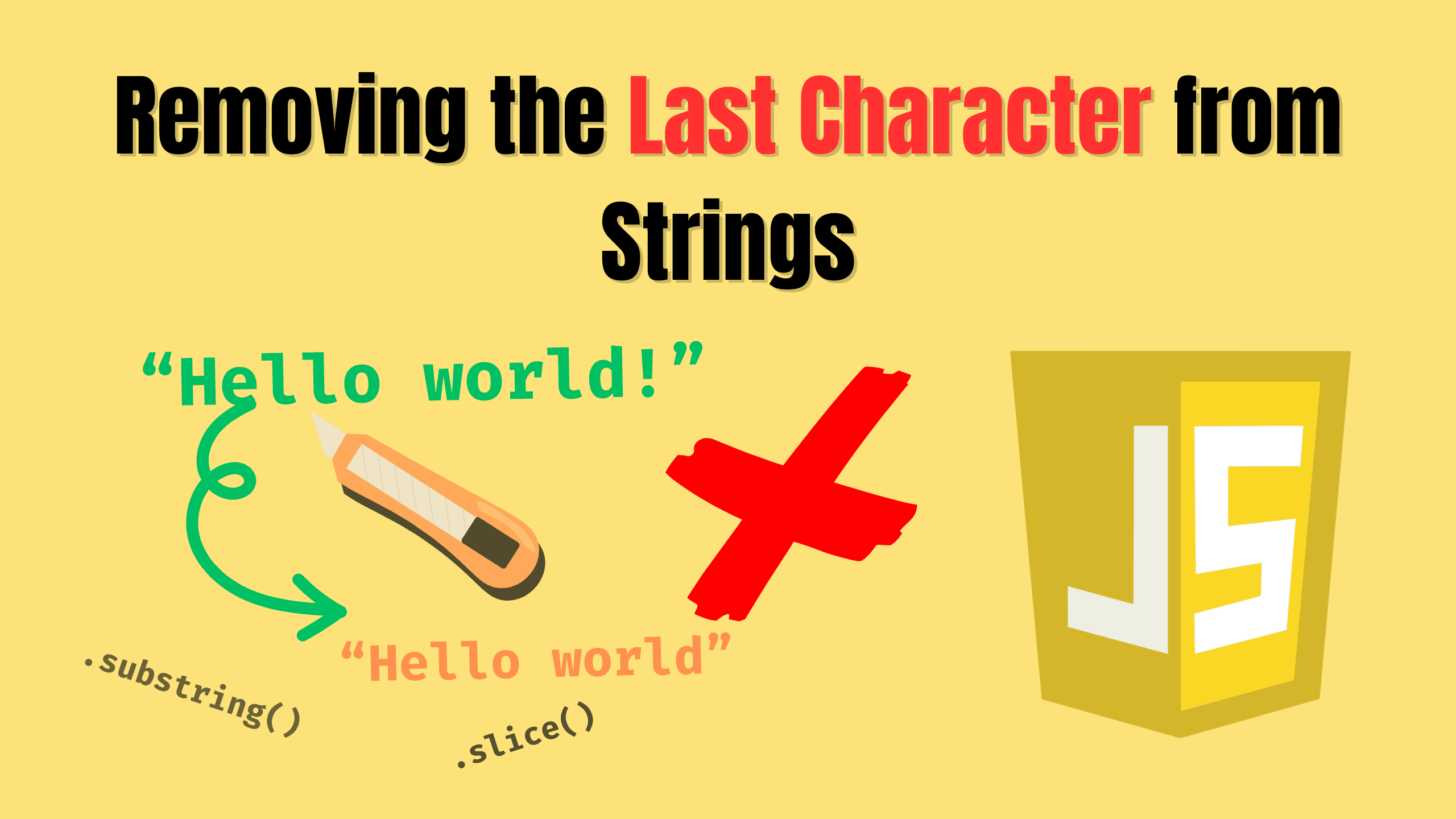
JavaScript developers, we've all been there. You've got a string, and it's almost perfect... except for that one pesky character hanging out at the very end. Maybe it's a trailing comma, an extra space, or just something you need to chop off. Whatever the reason, removing the last character from a string is a surprisingly common task.
But how do you do it in JavaScript without getting tangled up in complicated code? Don't worry, it's easier than you might think! In this guide, we'll explore simple, straightforward ways to trim the end of your strings. Let's dive in and learn how to give those strings a clean finish!
Common Reasons for Removing the Last Character
Before we jump into how, let's quickly chat about why you might want to do this. Understanding the "why" often makes the "how" much more meaningful. Here are a few real-world scenarios where removing the last character can be super handy:
- Cleaning up user input: Users are wonderfully unpredictable. Sometimes they might accidentally add extra spaces or characters at the end of text fields. Removing the last character can be a simple way to tidy up this input.
- Formatting data: When you're building strings programmatically, especially when looping through data, you might end up with a trailing delimiter – like a comma at the very end of a list. Chopping it off makes your output look much cleaner.
- Dealing with APIs: Occasionally, APIs might return data with unexpected trailing characters that you need to remove to properly process the information.
- Just good ol' string manipulation: Sometimes, you just need to modify strings for specific display or processing needs, and removing the last character is exactly what the doctor ordered!
Different Methods to Remove the Last Character
Okay, now we know why we might need to do this, let's get to the how. JavaScript provides us with some handy string methods that make this task a breeze. We'll look at a couple of the most common and easy-to-understand approaches:
1. slice() - The Gentle String Slicer
The slice() method is like a friendly knife for strings. It lets you extract a section of a string without changing the original string itself. To remove the last character, we can use slice() to grab everything except the very last bit.
Here's how it works:
javascriptlet myString = "Hello World!"; console.log("Original string:", myString); // Output: Original string: Hello World! let stringWithoutLastCharacter = myString.slice(0, myString.length - 1); console.log("String after slice:", stringWithoutLastCharacter); // Output: String after slice: Hello World console.log("Original string remains:", myString); // Output: Original string remains: Hello World!
Let's break it down like we're chatting over coffee:
- myString.slice(0, myString.length - 1): This is the magic line!
- slice(0, ...): We're telling slice() to start from the very beginning of the string (index 0).
- slice(..., myString.length - 1): And here's the clever part. myString.length gives us the total number of characters. We subtract 1 to get the index of the character before the last one. So, we're telling slice() to go from the start up to, but not including, the last character.
slice() creates a new string. It doesn't change the original myString. This is often a good thing, as it keeps your original data safe!
2. substring() - Another String Snipping Superstar
substring() is very similar to slice(), and it can achieve the same result. It also extracts a portion of a string. Let's see it in action:
javascriptlet anotherString = "Coding Fun?"; console.log("Original string:", anotherString); // Output: Original string: Coding Fun? let stringSub = anotherString.substring(0, anotherString.length - 1); console.log("String after substring:", stringSub); // Output: String after substring: Coding Fun console.log("Original string remains:", anotherString); // Output: Original string remains: Coding Fun?
The explanation is almost identical to slice():
- anotherString.substring(0, anotherString.length - 1):
- substring(0, ...): Start from the beginning (index 0).
- substring(..., anotherString.length - 1): Go up to, but not including, the index one less than the total length.
Just like slice(): substring() also returns a new string, leaving the original untouched.
So, slice() vs. substring()? For this particular task, they are pretty much interchangeable! Developers often choose slice() slightly more often in modern JavaScript, but substring() works perfectly well too. Pick whichever one you find more readable or easier to remember!
3. A More "Manual" Method (For Deeper Understanding)
While slice() and substring() are the most common and recommended ways, it's helpful to understand how you could also do this more "manually" using the string's length property and indexing.
javascriptlet manualString = "Last Try."; console.log("Original string:", manualString); // Output: Original string: Last Try. let stringManual = ""; // Start with an empty string for (let i = 0; i < manualString.length - 1; i++) { stringManual += manualString[i]; // Add each character *except* the last one } console.log("String after manual method:", stringManual); // Output: String after manual method: Last Try console.log("Original string remains:", manualString); // Output: Original string remains: Last Try.
Let's walk through this one step by step:
- let stringManual = "";: We create an empty string called stringManual. This is where we'll build our new string character by character.
- for (let i = 0; i < manualString.length - 1; i++) : We use a for loop to go through each character of the original string.
- i < manualString.length - 1: The loop continues as long as i is less than the length of the string minus one. This is crucial! It means the loop stops before it gets to the last character.
- stringManual += manualString[i];: Inside the loop, for each index i, we get the character using manualString[i] and add it (concatenate it +=) to our stringManual.
We build up stringManual by adding all characters from the original string except the very last one.
Why show this "manual" method? While slice() or substring() are more efficient and concise, understanding this manual approach can be really helpful for grasping how strings and loops work in JavaScript. It reinforces the idea of string indexing and iteration. For everyday coding, stick with slice() or substring(), but for learning, this manual method can be insightful.
Choosing the Right Method: Simplicity and Readability Win
For removing the last character from a string in JavaScript, both slice() and substring() are excellent and highly recommended choices. They are:
- Easy to read: Code using slice() or substring() is generally very clear and understandable.
- Efficient: These methods are built-in string operations and are optimized for performance.
- Concise: They achieve the result in a single, elegant line of code.
The "manual" method is more verbose and less efficient for this specific task. It's valuable for learning but not the best choice for everyday code where clarity and efficiency are key.
My recommendation? Go with slice() or substring(). They are the friendly, efficient, and readable tools for the job!
Conclusion
Alright, to wrap things up, removing the last character from a string in JavaScript is a common little task that's thankfully super easy to handle. With handy tools like slice() and substring() in your JavaScript toolkit, you can confidently trim those trailing characters and get your strings exactly how you need them. These methods are not only straightforward to use but also keep your code clean and efficient. So, go ahead and confidently snip away whenever you need to – JavaScript has got your back when you need to say goodbye to the end of a string! Happy coding, and may your strings always be perfectly trimmed!
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!