HTML5 Canvas with Examples: A Guide
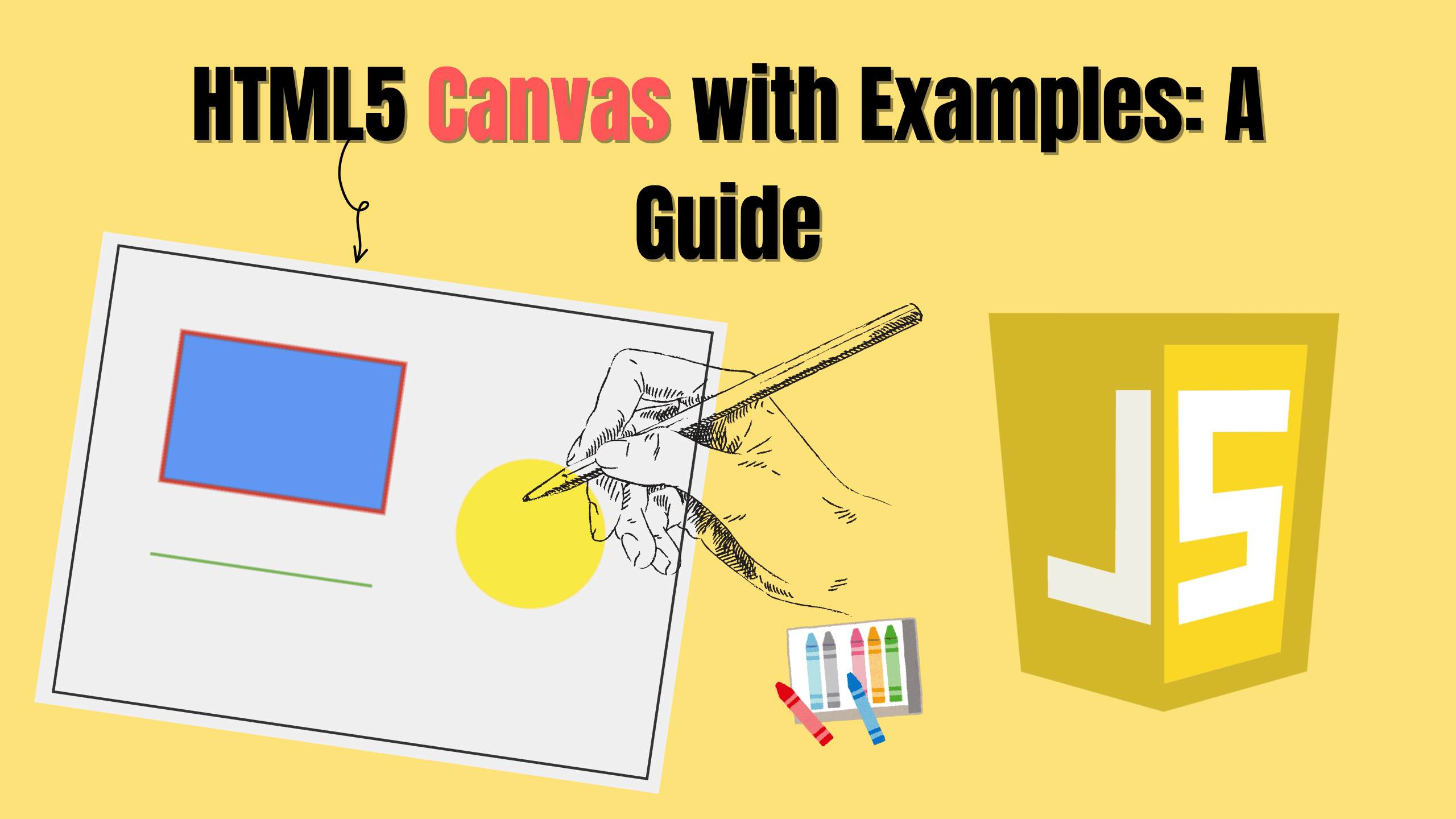
The HTML5 Canvas element is a powerful tool for rendering graphics, animations, and visual effects directly in the browser. It allows developers to create dynamic, interactive content without relying on external plugins like Flash. With Canvas, developers can create rich user experiences, including real-time visualizations, interactive infographics, and browser-based games. This blog will explore every aspect of the HTML5 Canvas, from basic usage to advanced techniques, with clear explanations and examples.
What is HTML5 Canvas?
The canvas element in HTML5 provides a way to draw graphics via JavaScript. It acts as a drawing board that allows developers to render 2D shapes, images, text, and even real-time animations. Unlike static image formats such as PNG or JPEG, the canvas is highly dynamic, meaning that graphics can be updated and manipulated programmatically using JavaScript. This makes it perfect for building interactive web applications, games, and data visualization tools.
Basic Syntax
js<canvas id="myCanvas" width="500" height="300"></canvas>
To draw on the canvas, JavaScript is required. This involves selecting the canvas and obtaining its drawing context:
jslet canvas = document.getElementById("myCanvas"); let ctx = canvas.getContext("2d");
Here, getContext("2d") returns a 2D rendering context, which provides a range of drawing methods for shapes, text, and images. Without this context, you wouldn't be able to draw anything on the canvas.
1. Drawing Basic Shapes
The Canvas API provides a set of drawing functions that allow developers to create various geometric shapes. Understanding how to draw basic shapes like rectangles, lines, and circles is essential before moving on to more complex designs and animations.
i. Rectangles
The canvas provides three methods to draw rectangles:
- fillRect(x, y, width, height): Draws a filled rectangle with the specified dimensions.
- strokeRect(x, y, width, height): Draws only the outline of a rectangle.
- clearRect(x, y, width, height): Clears a specific rectangular area on the canvas, making it transparent.
jsctx.fillRect(50, 50, 150, 100); ctx.strokeRect(50, 50, 150, 100);
This code snippet creates a solid blue rectangle with a red border. By adjusting the values, you can control the size and position of the rectangle on the canvas.
ii. Lines
A line is drawn by defining a path between two or more points using moveTo() and lineTo(), and then rendering it with the stroke() method to display it on the canvas. The beginPath() method is used to start a new drawing path, ensuring that previous paths do not interfere with the new line. By adjusting stroke color and line width, developers can further customize the appearance of the line.
jsctx.beginPath(); ctx.moveTo(50, 50); ctx.lineTo(200, 50); ctx.stroke();
Here, moveTo() sets the starting position, and lineTo() defines the endpoint. This creates a straight line on the canvas.
iii. Circles and Arcs
The arc() method is used to draw circles and arcs by specifying a center point, radius, and angle. It takes parameters such as the starting angle and ending angle, allowing developers to create partial arcs or full circles. This method is widely used for drawing circular shapes, clock faces, pie charts, and other curved elements in graphical applications.
jsctx.beginPath(); ctx.arc(100, 100, 50, 0, Math.PI * 2); ctx.fill();
This will create a solid filled circle at coordinates (100, 100) with a radius of 50 pixels. The Math.PI * 2 parameter ensures that the arc completes a full circle.
2. Working with Colors and Styles
Color and style settings play a crucial role in making graphics more visually appealing and engaging. The Canvas API allows developers to control colors in multiple ways, such as setting fill colors for shapes, defining stroke colors for outlines, and using transparency effects to create layering and depth. Additionally, gradients and patterns can be applied to enhance the visual experience, providing smooth transitions between colors or using textures to mimic real-world materials.
i. Fill and Stroke Colors
Colors are an essential part of any graphic, and the Canvas API provides various methods to control them. The fillStyle property sets the interior color of shapes, while strokeStyle defines the outline color, both supporting HEX, RGB, and HSL values. Here are both:
- fillStyle: Sets the fill color of a shape.
- strokeStyle: Sets the border color of a shape.
js// Fill Style ctx.fillStyle = "#ff5733"; ctx.fillRect(10, 10, 100, 100); // Stroke Style ctx.strokeStyle = "#000000"; ctx.lineWidth = 3; ctx.strokeRect(10, 10, 100, 100);
This fills a square with an orange-red color.
ii. Gradients
Canvas supports both linear and radial gradients, allowing smooth transitions between multiple colors. Linear gradients create color transitions along a straight line, while radial gradients form color shifts from a central point outward. These gradient effects can be used to enhance backgrounds, buttons, and dynamic visual elements for a more polished look.
js// Linear Gradient let linearGradient = ctx.createLinearGradient(0, 0, 200, 0); linearGradient.addColorStop(0, "red"); linearGradient.addColorStop(1, "blue"); ctx.fillStyle = linearGradient; ctx.fillRect(10, 10, 200, 100); // Radial Gradient let radialGradient = ctx.createRadialGradient(150, 150, 20, 150, 150, 100); radialGradient.addColorStop(0, "yellow"); radialGradient.addColorStop(1, "green"); ctx.fillStyle = radialGradient; ctx.fillRect(220, 10, 200, 200);
This creates a rectangle with a smooth transition from red to blue, giving it a visually appealing gradient effect.
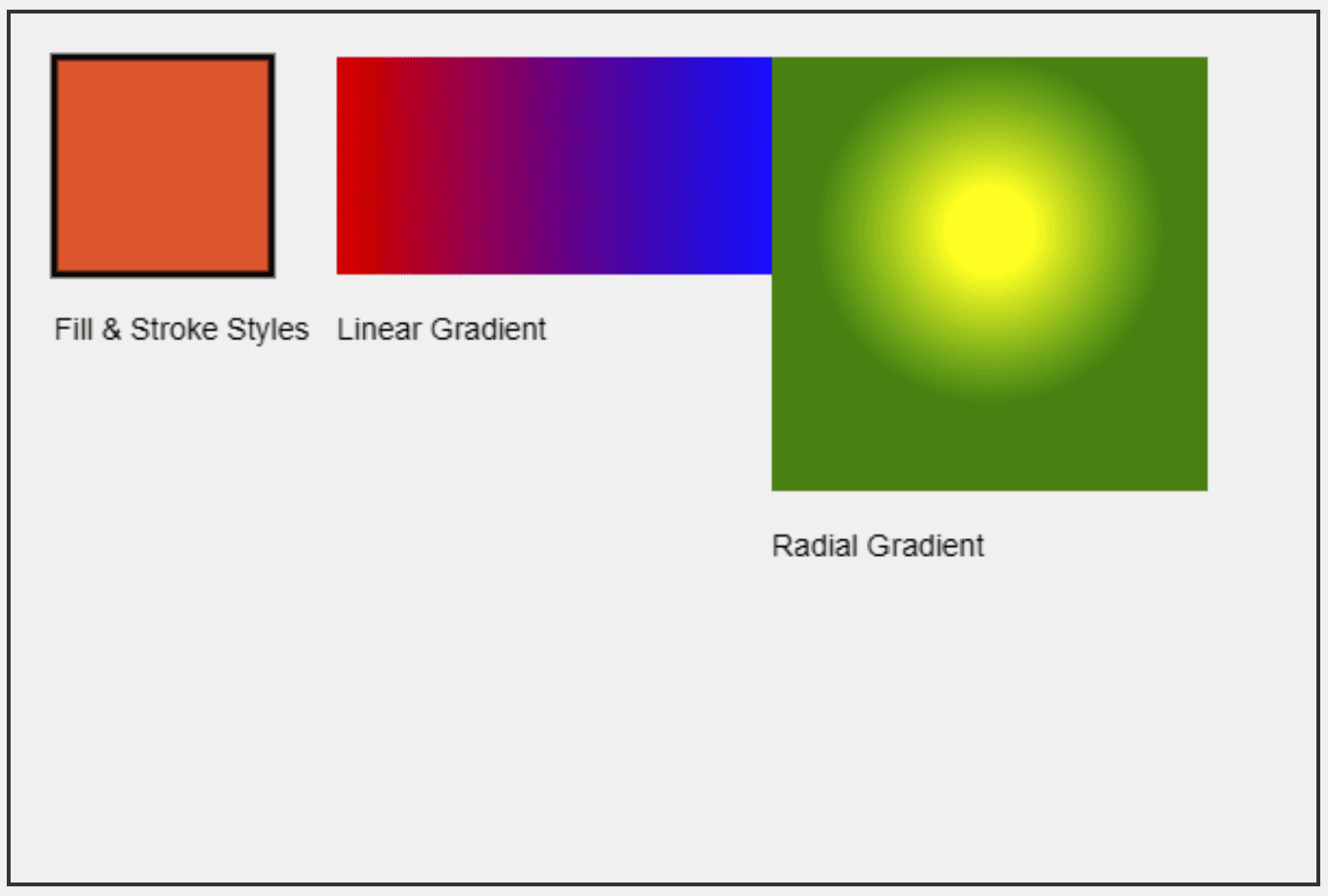
3. Adding Text to Canvas
Text can be drawn onto the canvas using the fillText() and strokeText() methods to create filled and outlined text. These methods support font customization, text alignment, and positioning for precise rendering.
i. Basic Text Rendering
The canvas API offers methods like fillText() and strokeText() to render text. These allow you to draw text with both a fill color and an outline.
jsctx.font = "30px Arial"; ctx.fillText("Hello, Canvas!", 50, 50); ctx.strokeText("Hello, Canvas!", 50, 100);
This code renders the text "Hello, Canvas!" in 30-pixel Arial font. The fillText() method draws solid text, while strokeText() creates outlined text.
ii. Text Alignment
The alignment of text can be adjusted using the textAlign() and textBaseline properties which can be top, middle, or bottom of the text (or other defined points like "alphabetic") aligns, allowing for precise positioning within the canvas.
jsctx.textAlign = "center"; ctx.fillText("Centered Text", canvas.width / 2, 50);
4. Handling Images on Canvas
Images can be drawn onto the canvas to create rich visual content, making applications more engaging. You can manipulate images by resizing, cropping, or applying filters to enhance the presentation and achieve various effects.
i. Drawing Images
First load the image and onload() event ensures it fully loads before drawing, preventing incomplete rendering. The drawImage() method positions the image starting from the top-left corner, making placement on the canvas straightforward.
jslet img = new Image(); img.src = "image.png"; img.onload = function() { ctx.drawImage(img, 10, 10); };
This loads an image and draws it at the specified coordinates.
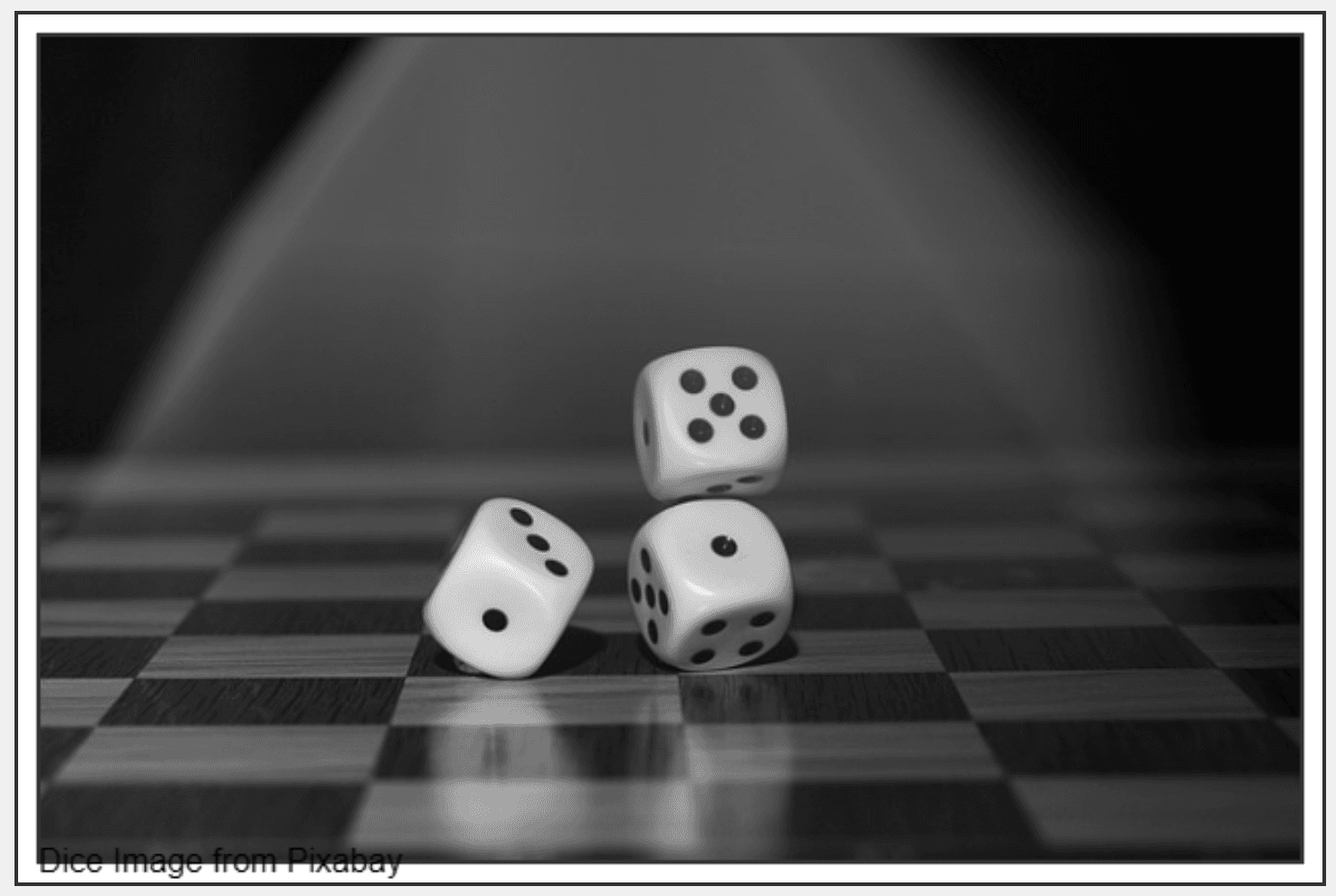
ii. Cropping Images
To display only a portion of an image, you can use the drawImage() method with specific coordinates and dimensions. This allows you to crop a selected part of the image and render it at a desired position on the canvas.
jsctx.drawImage(img, sx, sy, sWidth, sHeight, dx, dy, dWidth, dHeight);
This method lets you crop and scale images dynamically.
iii. Rotating Images
Image rotation on the canvas can be achieved using the rotate() method in combination with transformations. The rotation occurs around the canvas origin (0,0), so you may need to adjust the position of the image before and after rotation.
jsctx.save(); ctx.translate(x + width / 2, y + height / 2); ctx.rotate(angle * Math.PI / 180); ctx.drawImage(img, -width / 2, -height / 2, width, height); ctx.restore();
This ensures that the image rotates around its center while maintaining its position on the canvas.
5. Animations in Canvas
Creating smooth animations with HTML5 Canvas involves updating and redrawing the canvas content rapidly. By leveraging JavaScript’s requestAnimationFrame method, we can create efficient animations that sync with the browser’s refresh rate. Key techniques include clearing the canvas each frame, updating object positions, and applying transformations for dynamic effects. Below are essential animation concepts to bring your graphics to life.
i. Basic Animation Loop
The requestAnimationFrame method is the backbone of canvas animations. It recursively calls a function to redraw the canvas, ensuring smooth motion. Always clear the canvas with clearRect() before redrawing to avoid trails.
jslet x = 0; function animate() { ctx.clearRect(0, 0, canvas.width, canvas.height); ctx.fillRect(x, 50, 50, 50); x += 2; requestAnimationFrame(animate); } animate();
This code moves a square horizontally across the canvas at 60 frames per second (FPS).
ii. Transforming Objects
Use translate(), rotate(), and scale() to animate complex transformations. Always wrap transformations in save() and restore() to reset the canvas state.
jsctx.save(); ctx.translate(100, 100); ctx.rotate(angle * Math.PI / 180); ctx.fillRect(-25, -25, 50, 50); // Rotates around center ctx.restore();
This rotates a square around its center point.
iii. Controlling Frames Per Second (FPS)
By default, requestAnimationFrame syncs with the browser’s refresh rate (typically 60 FPS), which is ideal for smooth animations. However, you might want to limit FPS to:
- Reduce CPU/GPU usage for performance optimization.
- Create slower animations for artistic effects.
- Synchronize with external processes (e.g., game logic or physics calculations).
Instead of updating the canvas on every frame, you use timestamps to track elapsed time and only update when a specific interval (e.g., 30 FPS) has passed.
jslet prevTime = 0; function animate(timestamp) { if (timestamp - prevTime > 1000 / 30) { // 30 FPS updatePosition(); prevTime = timestamp; } requestAnimationFrame(animate); }
6. Advanced Canvas Techniques
Beyond basic drawing and animations, the Canvas API offers advanced features for complex visual effects and optimizations. Techniques like clipping paths restrict drawing to specific areas, while composite operations enable blending layers. Integrating WebGL unlocks 3D rendering, and Offscreen Canvas improves performance by moving tasks to a separate thread.
i. Clipping Paths
Use clip() to restrict drawing to a custom shape. Define a path and call clip() to create a mask.
jsctx.beginPath(); ctx.arc(100, 100, 50, 0, Math.PI * 2); ctx.clip(); ctx.fillRect(0, 0, 200, 200); // Only visible inside the circle
ii. Composite Operations
The globalCompositeOperation property controls how new drawings blend with existing content. Use values like 'multiply' or 'screen' for creative effects.
jsctx.globalCompositeOperation = 'xor'; ctx.fillRect(50, 50, 100, 100);
FAQS
- How to clear canvas HTML5? Use the clearRect() method to clear a specific area or the entire canvas:
jsctx.clearRect(0, 0, canvas.width, canvas.height);
- What is the HTML5 canvas? HTML5 canvas is a rectangular area on a web page where you can draw graphics, animations, and images dynamically using JavaScript.
- How to draw an arrow in HTML5 canvas? To draw an arrow, use the moveTo() and lineTo() methods to create the shaft and the lineTo() again to form the arrowhead. Here's a basic example:
jsctx.moveTo(50, 50); ctx.lineTo(150, 50); ctx.lineTo(130, 30); ctx.moveTo(150, 50); ctx.lineTo(130, 70);
- How to add a button in canvas HTML5? HTML5 canvas itself does not have buttons, but you can create a button using HTML and position it over the canvas using CSS.
- Can video animation be made with HTML5 canvas? Yes, you can create video-like animations on the canvas by drawing frames of a video or using JavaScript to update the canvas continuously for animations.
Conclusion
The HTML5 Canvas API is a versatile and powerful tool for creating dynamic graphics. Whether you are building interactive visualizations, games, or animations, mastering the canvas can take your web development skills to the next level. Start experimenting with the various features covered in this guide and bring your creative ideas to life!
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!