8 Essential JavaScript Array Functions
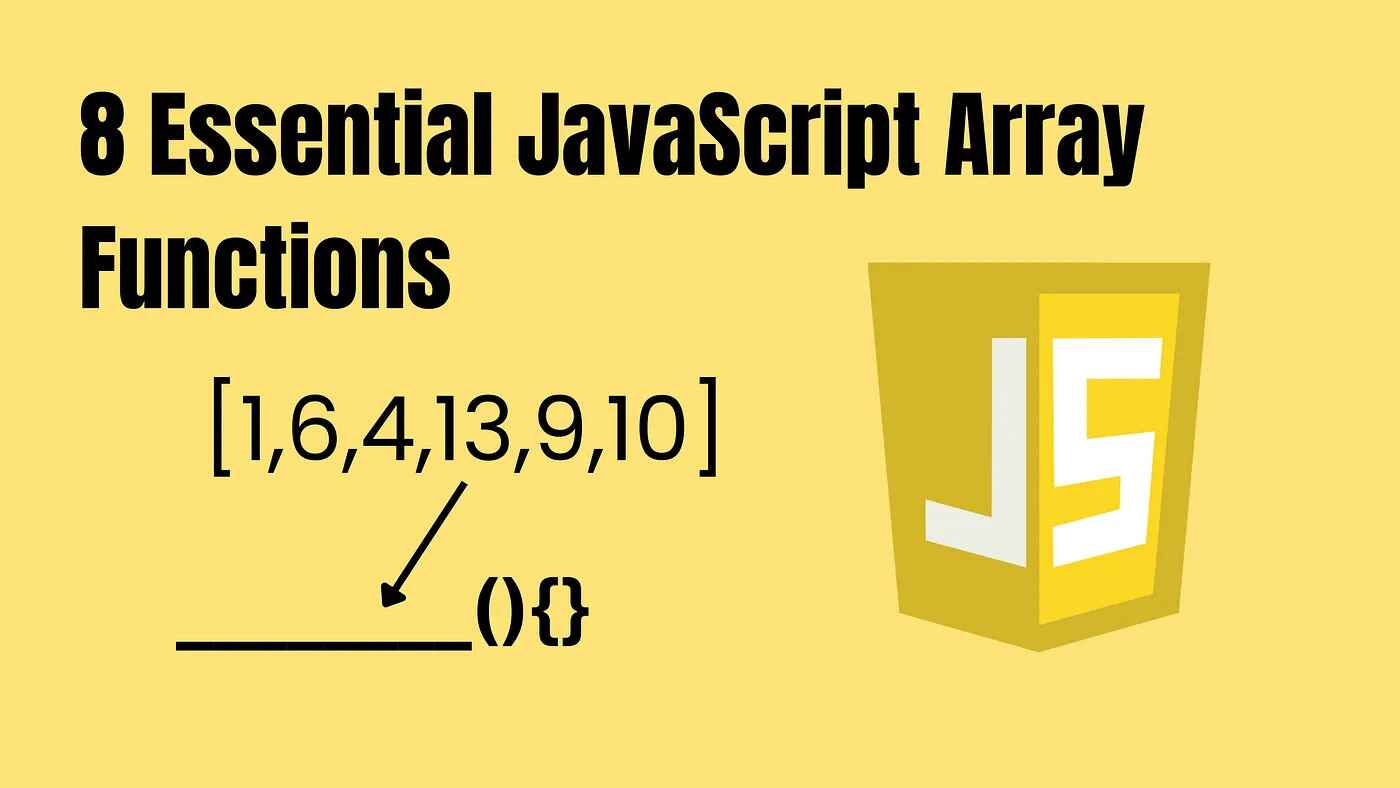
Arrays are the backbone of JavaScript. They’re powerful, flexible, and, with the right functions, can make your code much cleaner. Here are eight array functions every developer needs to understand.
1. map() – Transforming Data
map() is like a factory for arrays. It takes your original array and transforms each element, returning a new array. Perfect for when you need to apply a function to every item.
jsconst numbers = [1, 2, 3]; const doubled = numbers.map(num => num * 2); console.log(doubled); // [2, 4, 6]
2. filter() – Picking What Matters
Need to pick out specific items from your array? filter() is your go-to. It creates a new array with only the elements that pass a test you provide.
jsconst ages = [16, 21, 18, 14]; const adults = ages.filter(age => age >= 18); console.log(adults); // [21, 18]
3. reduce() – Boiling It Down
reduce() is all about reducing your array into a single value. Whether it’s summing numbers or merging objects, it’s super versatile.
jsconst numbers = [1, 2, 3, 4]; const sum = numbers.reduce((total, num) => total + num, 0); console.log(sum); // 10
4. forEach() – Doing It All
Need to do something with each item in an array but don’t need a new array? forEach() is like a loop that’s built right into your array.
jsconst colors = ['red', 'green', 'blue']; colors.forEach(color => console.log(color)); // Output: red green blue
5. find() – Finding the Needle
find() helps you search for an element in your array. It returns the first match it finds, or undefined if there’s no match.
jsconst users = [{name: 'John'}, {name: 'Jane'}, {name: 'Jack'}]; const user = users.find(user => user.name === 'Jane'); console.log(user); // {name: 'Jane'}
6. some() – Is It There?
some() checks if at least one item in your array passes the test. It’s like asking, “Does this array have anything like this?”
jsconst numbers = [1, 2, 3, 4]; const hasEven = numbers.some(num => num % 2 === 0); console.log(hasEven); // true
7. every() – All or Nothing
every() is the opposite of some(). It checks if all items pass the test. If even one fails, it returns false.
jsconst numbers = [2, 4, 6]; const allEven = numbers.every(num => num % 2 === 0); console.log(allEven); // true
8. sort() – Putting Things in Order
sort() reorders your array based on a function you provide. Be careful—by default, it sorts as if everything is a string.
jsconst letters = ['b', 'c', 'a']; letters.sort(); console.log(letters); // ['a', 'b', 'c']
Conclusion
These array functions are like your best tools in the toolbox. The more you use them, the more you’ll appreciate their power. Happy coding!!!
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!