How to validate an Email with Regex in Javascript?
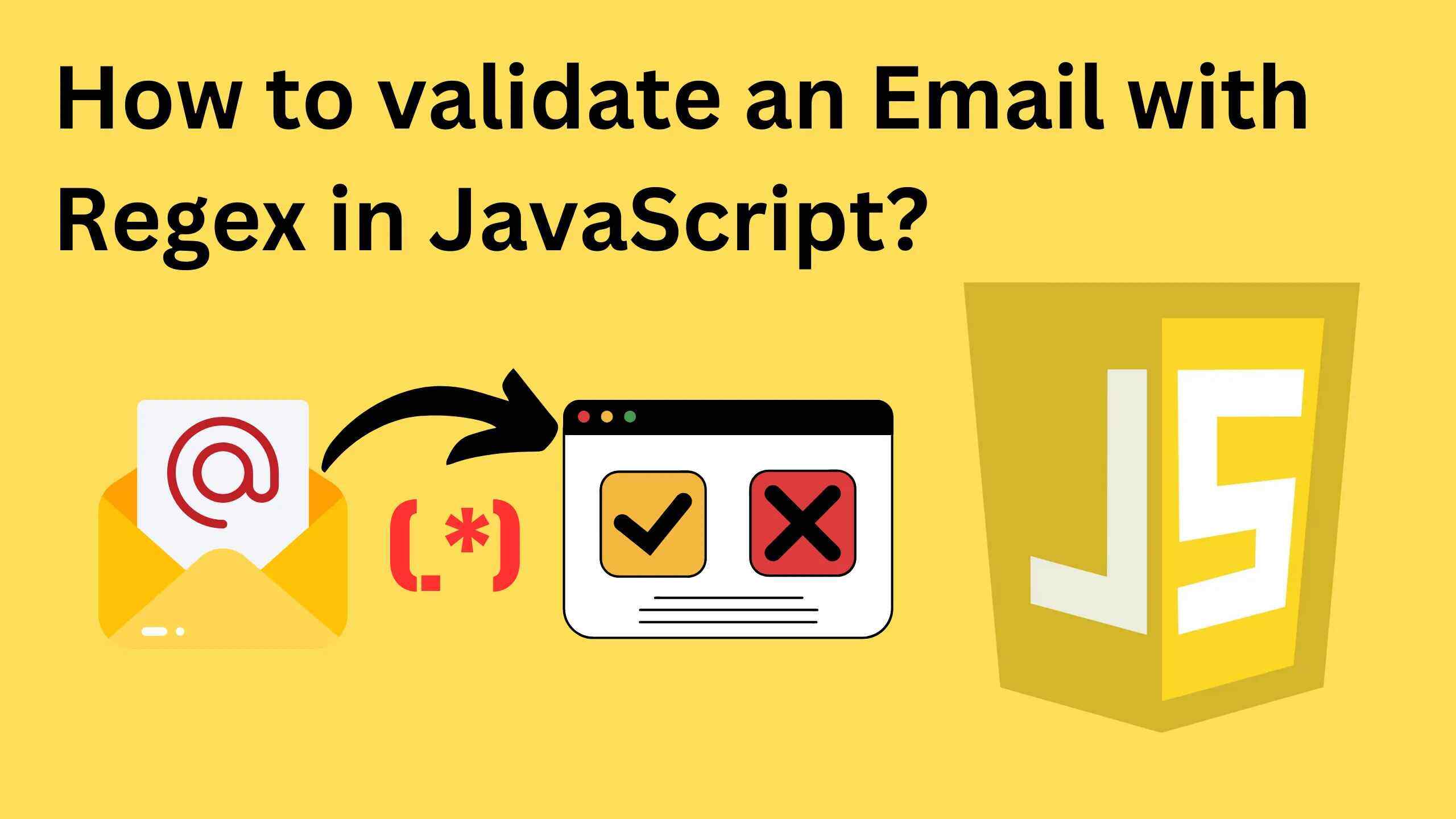
Validating an email with regex (regular expressions) in JavaScript is a common task for web developers. It ensures that email addresses entered by users conform to a standard format. Here’s a straightforward guide on how to validate an email with regex in JavaScript.
Why? Email validation helps prevent errors in email submissions, ensuring that users provide valid email addresses. This is crucial for effective communication and for reducing bounce rates in email campaigns.
1. Regular Expression for all Emails
A regular expression is a pattern used to match character combinations in strings. For email validation, the regex checks for the general structure of an email address, including the local part, the “@” symbol, and the domain part.
Here’s a regex pattern commonly used for email validation:
jsconst emailPattern = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
The regex pattern begins by asserting the start of the string with ^. It then matches one or more characters that are not spaces or "@" symbols with [^\s@]+, representing the local part of the email. Following this, it matches the "@" symbol with "@", and again matches one or more characters that are not spaces or "@" symbols using [^\s@]+, which represents the domain name. The ., which matches the literal dot, is followed by another [^\s@]+ to match one or more characters that are not spaces or "@" symbols, representing the top-level domain. Finally, the pattern asserts the end of the string with $.
2. Regular Expression for Specific Domains Emails
To validate email addresses that end with specific domains, you can use a regex pattern that includes those domains. Here’s a pattern to match emails that end with gmail.com or any other specified domains:
jsconst specificDomainPattern = /^[^\s@]+@(gmail\.com|example\.com|anotherdomain\.com)$/;
The regex pattern begins with ^, asserting the start of the string. It then matches one or more characters that are not spaces or "@" symbols with [^\s@]+, representing the local part of the email. The "@" symbol is matched next with "@". Following this, the pattern matches any of the specified domains— gmail.com, example.com, or anotherdomain.com — using (gmail.com|example.com|anotherdomain.com), where the pipe | symbol acts as a separator between the options. The pattern concludes by asserting the end of the string with $.
Implementing Email Validation in JavaScript
You can use the regex pattern to validate an email address by matching it against the pattern in JavaScript. Here’s a simple JavaScript function to check if an email address is valid:
jsfunction isValidEmail(email) { const emailPattern = /^[^\s@]+@[^\s@]+\.[^\s@]+$/; return emailPattern.test(email); } // Example usage const email = "example@example.com"; console.log(isValidEmail(email)); // true
Why Use This Regex?
This regex is a basic example and handles the most common cases. However, email validation is complex due to various valid email formats. Consider using built-in libraries or validators that follow the official standards for more advanced validation in JavaScript.
Conclusion
Validating an email with regex in JavaScript is a straightforward process that ensures user inputs are in the correct format. By using the regex pattern provided, you can quickly implement basic email validation in your applications. Happy Coding!!!
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!