Tween in Phaser JS to animate objects
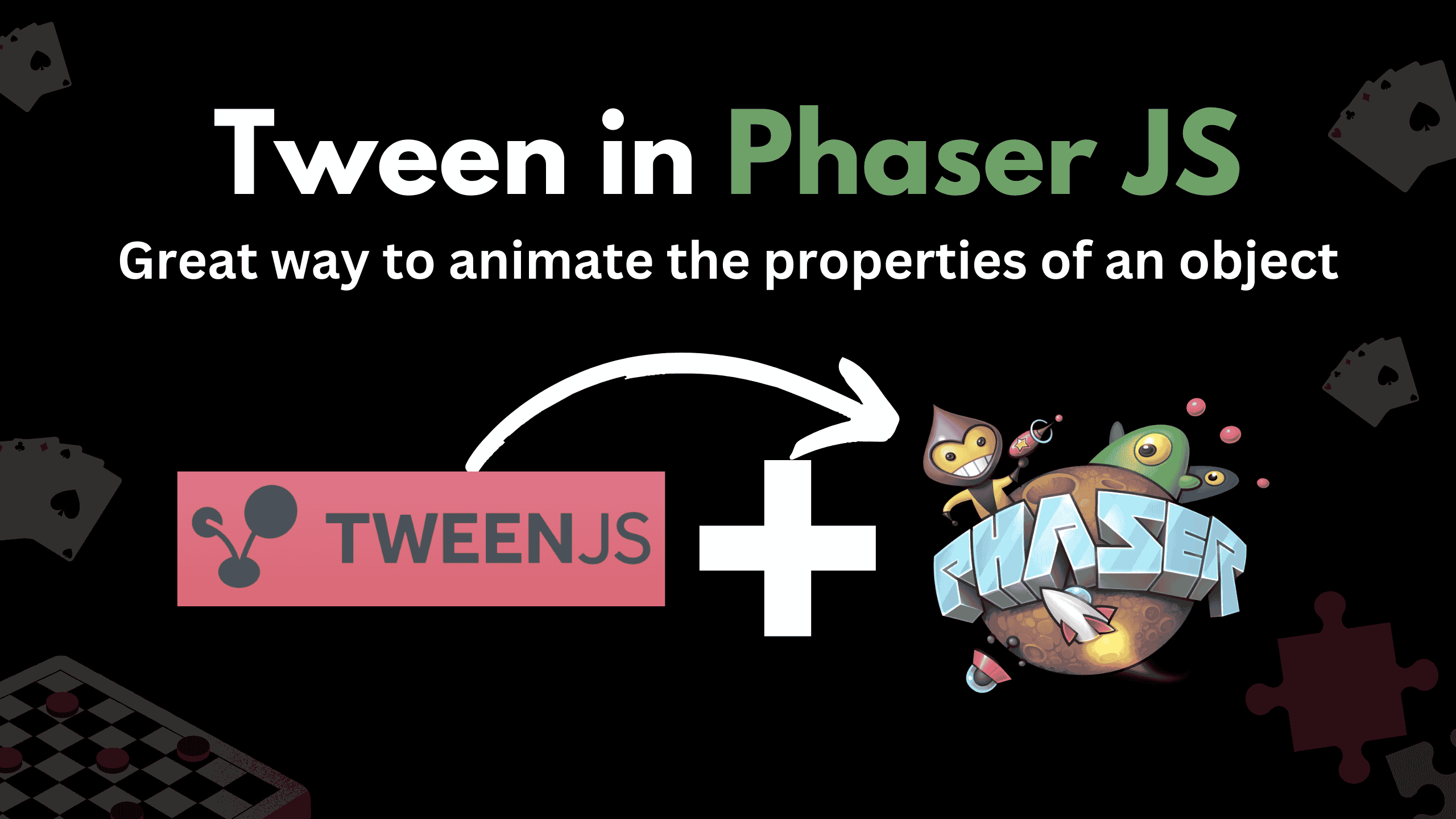
Tweens are used to create smooth animations by transitioning the values of properties over time. They are ideal for moving objects, scaling, fading, rotating, or creating any kind of visual effect that makes your game more dynamic and engaging.
In this blog, we will explore the concept of tweens in Phaser.js in detail, covering their setup, configuration, and practical use cases with code examples.
Phaser JS:
Phaser JS is a fast and robust open-source game framework used to develop HTML5 games. Phaser JS is particularly great for developing 2D games and supports both Canvas and WebGL rendering.
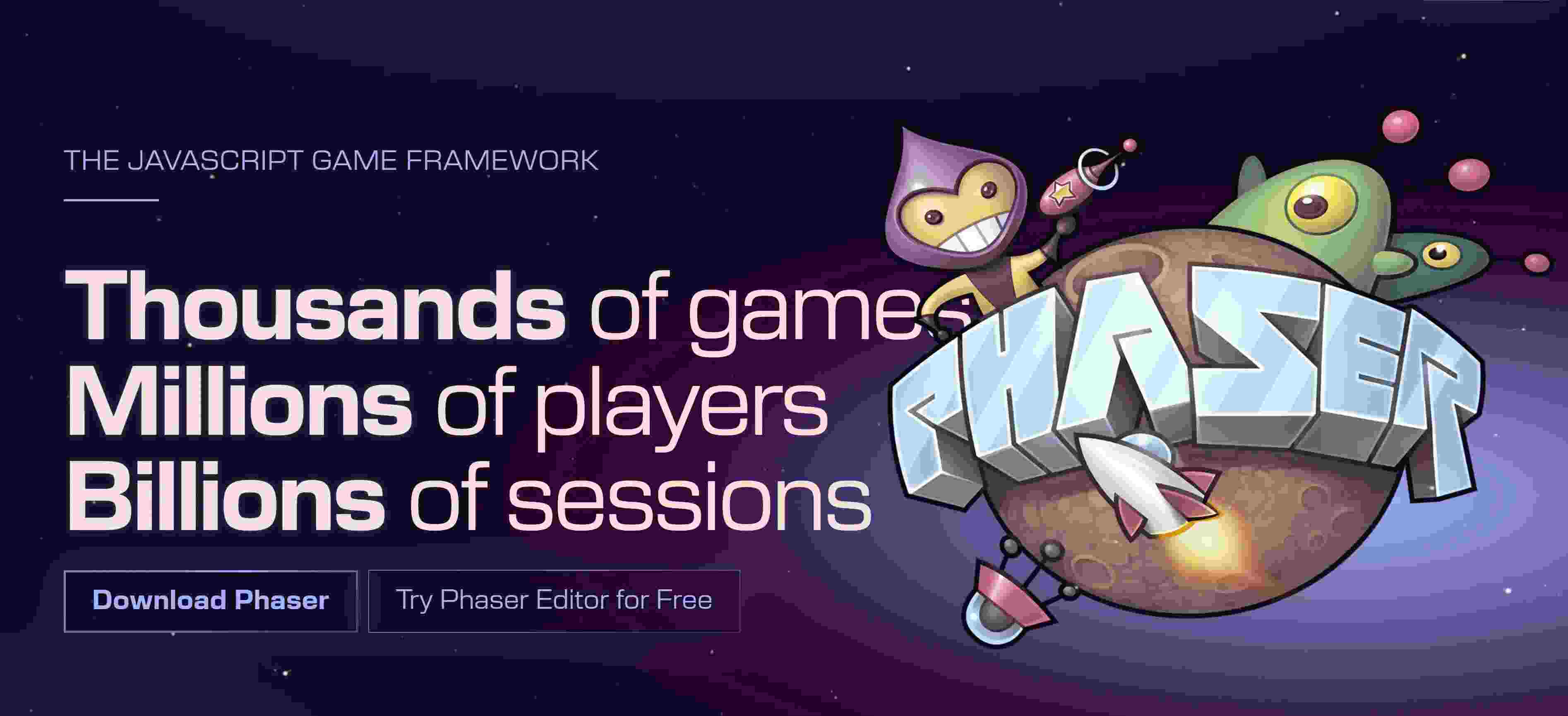
What is a Tween?
A tween is a mechanism for animating the properties of an object, such as its position, scale, or alpha (transparency), over a specified duration. Instead of updating values manually in each frame, tweens automatically interpolate between starting and ending values, saving time and ensuring smoother animations.
e.g: Can move a character from point A to point B, fade out an image, or create a bouncing ball effect using tweens.
Let me explain this,
How Tweens Work?
In Phaser, tweens are managed by the Tween Manager. You define tweens by specifying the properties you want to animate, the duration of the animation, and optional easing functions to control the motion. Phaser handles the interpolation and updates the object's properties frame by frame.
javascriptthis.tweens.add({ targets: object, // The object whose properties will be animated property: value, // The property and target value duration: time, // Duration of the tween in milliseconds ease: 'easeFunction', // Easing function (optional) repeat: times, // How many times the tween repeats (optional) yoyo: true/false, // Reverse animation on repeat (optional) });
Creating a Simple Tween
To understand tweens, let's start with a simple example of moving a sprite across the screen.
javascriptthis.tweens.add({ targets: logo, x: 700, // Move to x = 700 duration: 2000, // 2 seconds ease: 'Linear', // Linear easing repeat: 0, // No repetition yoyo: false // No reverse });
How it works? Let me explain it,
- targets: Specifies the object to animate (logo in this case).
- x: 700: Moves the sprite to an x coordinate of 700.
- duration: 2000: Takes 2000 milliseconds (2 seconds) to complete the tween.
- ease: 'Linear': Animates with a constant speed.
Here is fully setup phaser js project with this basic example. Enjoy it
Advanced Tween Features
Phaser's tween system is highly customizable. Here are some advanced features and how to use them:
1. Easing Functions
Easing functions determine the rate of change during the animation. Phaser supports a variety of easing types, such as Linear, Bounce, Elastic, and more.
javascriptthis.tweens.add({ targets: logo, x: 700, duration: 2000, ease: 'Bounce', // Bounce effect });
Available easing functions include:
- Phaser.Math.Easing.Linear
- Phaser.Math.Easing.Quadratic.In
- Phaser.Math.Easing.Quadratic.Out
- Phaser.Math.Easing.Elastic.InOut
2. Repeating Tweens
You can make a tween repeat multiple times or infinitely using the repeat property.
javascriptthis.tweens.add({ targets: logo, x: 700, duration: 2000, repeat: -1, // -1 for infinite repetition });
3. YoYo Effect
The yoyo property makes the tween reverse after completing.
javascriptthis.tweens.add({ targets: logo, x: 700, duration: 2000, repeat: -1, yoyo: true, // Moves back to the starting position });
4. Delays
Use delay to wait before starting the tween or between repeats.
javascriptthis.tweens.add({ targets: logo, x: 700, duration: 2000, delay: 500, // Waits 500ms before starting });
Chaining Multiple Tweens
Phaser supports chaining tweens for creating sequential animations. This can be done using the onComplete callback or by using a timeline.
1. Using onComplete
javascriptthis.tweens.add({ targets: logo, x: 700, duration: 2000, onComplete: () => { this.tweens.add({ targets: logo, y: 100, duration: 1000, }); } });
2. Using Timelines
Timelines allow you to sequence multiple tweens easily.
javascriptconst timeline = this.tweens.createTimeline(); timeline.add({ targets: logo, x: 700, duration: 2000, }); timeline.add({ targets: logo, y: 100, duration: 1000, }); timeline.play();
Tweening Multiple Objects
You can animate multiple objects simultaneously by passing an array to the targets property.
javascriptconst sprites = [sprite1, sprite2, sprite3]; this.tweens.add({ targets: sprites, alpha: 0, // Fade out all sprites duration: 1000, ease: 'Linear', });
Tween Callbacks
Phaser tweens include several callbacks for handling different stages of the tween lifecycle:
- onStart: Triggered when the tween starts.
- onUpdate: Called during every frame of the tween.
- onComplete: Called when the tween finishes.
- onRepeat: Fired when the tween repeats.
javascriptthis.tweens.add({ targets: logo, x: 700, duration: 2000, onStart: () => console.log("Tween started!"), onComplete: () => console.log("Tween complete!"), });
Debugging Tweens
Phaser provides tools like tweens.pause(), tweens.resume(), and tweens.remove() to control active tweens during development, making debugging easier.
Conclusion
Tweens in Phaser.js are a powerful way to add dynamic motion and polish to your games. From moving objects and fading effects to complex animations and chained sequences, tweens help create engaging and visually appealing experiences. By mastering the tweening system, you can bring your game scenes to life and delight players with smooth, fluid animations. Start experimenting with tweens today to unlock their full potential!
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!