Javascript Double Exclamation Mark (!!) - A Guide
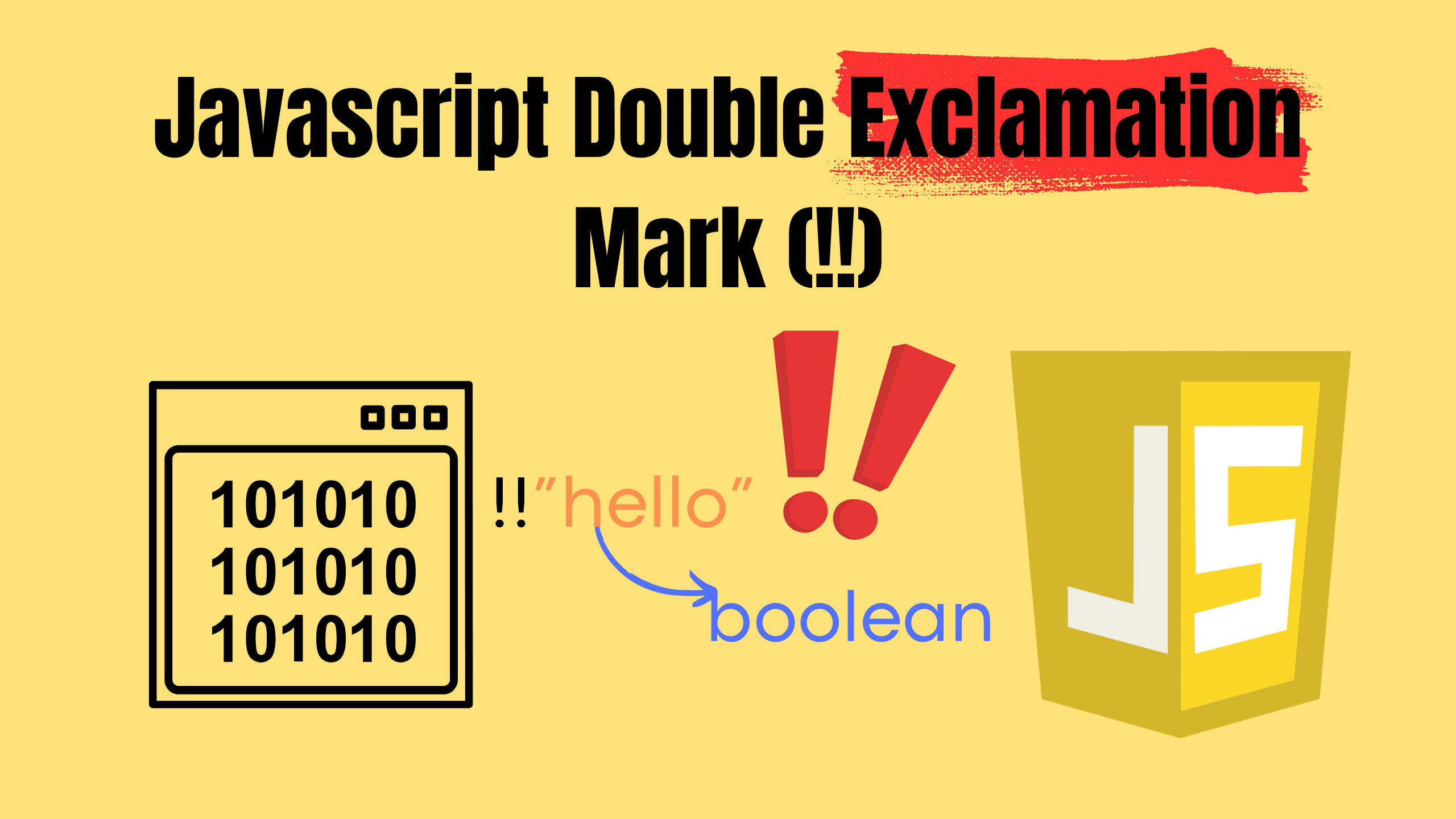
JavaScript developers often encounter the double exclamation mark (!!) in their code. Many pause to ask, "What does it really do?" Though it may seem cryptic at first, this operator—commonly known as the "double bang"—is far from trivial. When understood, it becomes a powerful tool for converting values to booleans and enhancing code clarity.
In this guide, we’ll break down the JavaScript double exclamation mark. We’ll explore what it is actually, uncover how it works under the hood, and reveal why it’s a valuable asset in toolkit. Let’s dive in and unlock the secrets of the double bang!
What is the Double Exclamation Mark (!!)?
In JavaScript, the double exclamation mark (!!) isn’t an independent operator like + or -. Instead, it’s simply the logical NOT operator (!) applied twice in succession. Essentially, !! converts any JavaScript value to its corresponding boolean value—true or false—by leveraging the language’s inherent concepts of truthiness and falsiness.
Before we delve deeper, it's crucial to have a solid grasp of two fundamental Javascript concepts that underpin its functionality: Type Coercion and the Logical NOT operator.
Type Coercion
Type coercion is the automatic or implicit conversion of values from one data type to another in Javascript. This often happens when Javascript operators or functions expect a certain data type, and the provided value is of a different type. Javascript, being a loosely typed language, frequently performs type coercion to attempt to execute operations without throwing errors. Let's look at common example:
javascriptlet num = 5; let str = " is a number"; let result = num + str; // Number 5 is coerced to a string console.log(result); // Output: 5 is a number console.log(typeof result); // Output: string
Here, the number 5 is coerced into a string so that it can be concatenated with the string " is a number".
Truthiness and Falsiness
Type coercion automatically converts values to booleans in conditional expressions and logical operations. This behavior is guided by two important concepts: truthiness and falsiness. Every JavaScript value is inherently either truthy or falsy, which determines how it behaves in boolean contexts.
Falsy Values
In JavaScript, there are exactly eight values that are considered falsy. When any of these values are evaluated in a boolean context, they convert to false. These values are:
- false (the literal false value)
- 0 (numeric zero)
- -0 (numeric negative zero)
- "" (an empty string)
- null
- undefined
- NaN (Not a Number)
- 0n (BigInt zero)
Truthy Values
Any value that is not one of the eight falsy values is considered truthy. This means that even values that might seem empty or insignificant will evaluate to true in a boolean context. Common examples include:
- true (the literal true value)
- "hello" (non empty string)
- 5 (any positive or negtive number)
- [] (an empty array)
- (an empty object)
- function() (functions are object)
Logical NOT Operator (!)
Logical NOT Operator (!) is a unary operator that converts any given value into its boolean equivalent and then inverts that value. This means it changes truthy values to false and falsy values to true, ensuring that the result is always a boolean. It performs the following actions:
- Evaluates the operand: It takes a single operand (the value to its right).
- Coerces the operand to a boolean: It internally converts the operand to its boolean equivalent based on truthiness or falsiness.
- Negates the boolean value: It then returns the logical negation of this boolean value. If the coerced boolean was true, it returns false, and if it was false, it returns true.
See here some examples:
javascriptconsole.log("! true:", !true); // Output: ! true: false console.log("! undefined:", !undefined); // Output: true console.log("! 10:", !10); // Output: false console.log("! []:", ![]); // Output: false console.log("! {}:", !{}); // Output: false
How Exclamation Mark (!!) works?
Regardless of the initial data type (string, number, null, undefined), applying !! consistently results in a boolean value (true or false)., when we apply !! (two exclamation marks), we are essentially applying the logical NOT operation twice. Let's break it down step-by-step:
- The first ! converts the value to its boolean equivalent and then negates it.
- The second ! operates on the result from the first step. It again converts the value (which is now a boolean) to its boolean equivalent (which remains the same as it's already a boolean) and then negates it again.
The crucial part is the double negation. By negating the boolean value twice, we effectively return the original boolean equivalent of the initial value, but now explicitly as a boolean primitive. Loot at simple examples:
javascriptlet message = "Hello"; let isEmptyString = ""; let number = 10; let zero = 0; let nullValue = null; let undefinedValue = undefined; console.log("Original Values & Types:"); console.log("message:", message, typeof message); // message: Hello string console.log("isEmptyString:", isEmptyString, typeof isEmptyString); // isEmptyString: string console.log("number:", number, typeof number); // number: 10 number console.log("zero:", zero, typeof zero); // zero: 0 number console.log("nullValue:", nullValue, typeof nullValue); // nullValue: null object console.log("undefinedValue:", undefinedValue, typeof undefinedValue); // undefinedValue: undefined undefined console.log("\nUsing Double Exclamation Mark (!!):"); console.log("!!message:", !!message, typeof !!message); // !!message: true boolean console.log("!!isEmptyString:", !!isEmptyString, typeof !!isEmptyString); // !!isEmptyString: false boolean console.log("!!number:", !!number, typeof !!number); // !!number: true boolean console.log("!!zero:", !!zero, typeof !!zero); // !!zero: false boolean console.log("!!nullValue:", !!nullValue, typeof !!nullValue); // !!nullValue: false boolean console.log("!!undefinedValue:", !!undefinedValue, typeof !!undefinedValue); // !!undefinedValue: false boolean
As you can see, regardless of the initial data type (string, number, null, undefined), applying !! consistently results in a boolean value (true or false).
Use Cases for Double Exclamation Mark (!!)
Now that we understand how !! works, let's explore some common and practical use cases where it can be beneficial in your Javascript code.
1. Explicit Boolean Conversion
This is the most primary and frequent use case for !!. When you need to ensure that a variable or expression is definitively a boolean value (true or false), !! is a concise and effective way to achieve this.
javascriptfunction processData(isActive) { // Ensure isActive is treated as a boolean const isProcessActive = !!isActive; if (isProcessActive) { console.log("Processing data..."); // ... perform data processing logic } else { console.log("Process is inactive."); } } processData(1); // Output: Processing data... (1 is truthy, !!1 is true) processData("yes"); // Output: Processing data... ("yes" is truthy, !!"yes" is true) processData(0); // Output: Process is inactive. (0 is falsy, !!0 is false) processData(null); // Output: Process is inactive. (null is falsy, !!null is false) processData(true); // Output: Processing data... (true is truthy, !!true is true)
In this example, even if processData is called with a truthy value like 1 or "yes", !!isActive guarantees that isProcessActive will always be a boolean (true in these cases), making the conditional logic predictable and robust.
2. Working with APIs and Data
When fetching data from APIs or processing external data sources, you often encounter values that are not strictly boolean but represent boolean-like states (e.g., "true", "false", 1, 0, or presence/absence of a value). !! can be handy for normalizing these values to booleans for consistent logic in your application.
javascriptasync function fetchUserData() { const response = await fetch('/api/user-data'); const data = await response.json(); const isUserActive = !!data.isActive; // Assume API might return "true", "false", 1, 0, null, etc. if (isUserActive) { console.log("User is active."); // ... perform actions for active users } else { console.log("User is inactive."); // ... perform actions for inactive users } } // Assume API returns: { isActive: "true", userName: "John" } or { isActive: 0, userName: "Jane" } fetchUserData();
In this API interaction scenario, !!data.isActive ensures that regardless of whether the API returns "true", 1, true, or even null or 0 for the isActive property, your code reliably interprets it as a boolean true or false.
FAQs
- Is !!value the same as Boolean(value)? Yes, functionally, !!value is equivalent to Boolean(value). Both achieve explicit conversion of value to its boolean equivalent. However, !!value is a more concise idiom, while Boolean(value) might be slightly more verbose but arguably more readable for developers completely unfamiliar with !!.
- Is there a performance difference between !!value and Boolean(value)? In practice, the performance difference between !!value and Boolean(value) is extremely minimal and usually negligible. Both are very efficient operations. Micro-benchmarks might show a tiny variance, but in real-world applications, it's unlikely to be a factor. Choose based on code style and readability preferences rather than performance concerns.
- Can !! be used with any Javascript data type? Yes, !! can be used with any Javascript value, regardless of its data type (string, number, object, array, null, undefined, etc.). Javascript's type coercion will handle the initial conversion to a boolean before the negation operations are applied.
- Is !! a standard Javascript operator? No, !! is not a standalone operator. It is simply the logical NOT operator ! applied twice in succession. It's an idiom that leverages the behavior of the ! operator and type coercion in Javascript.
- Is using !! considered good or bad practice? Using !! is generally considered good practice when you need explicit boolean conversion and conciseness is valued. It's a widely recognized and accepted idiom in the Javascript community. However, like any language feature, it should be used judiciously. Prioritize readability and clarity, and consider your audience's familiarity with the idiom. For beginners or in situations where clarity is paramount, Boolean(value) might be a slightly more explicit alternative.
Conclusion
The double exclamation mark (!!) is a succinct and powerful way to convert any JavaScript value into its boolean equivalent. By applying the logical NOT operator twice, you effortlessly tap into JavaScript’s inherent type coercion mechanism. This technique not only clarifies your intentions in code but also streamlines conditional checks, ensuring that values are evaluated precisely as intended.
Whether you’re working with unpredictable user input or data returned from APIs, using !! offers a clean and concise solution for normalizing values. While it performs the same function as Boolean(), its brevity often makes your code more elegant and readable—provided you balance conciseness with clarity. This makes it an essential idiom in the modern JavaScript developer’s toolkit.
Ultimately, mastering the double exclamation mark goes beyond just a neat trick—it reinforces your understanding of JavaScript’s dynamic nature and deepens your ability to write robust, maintainable code. Embrace this concept as you continue to explore and build more reliable applications. Happy coding!
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!