Fibonacci Series in JavaScript
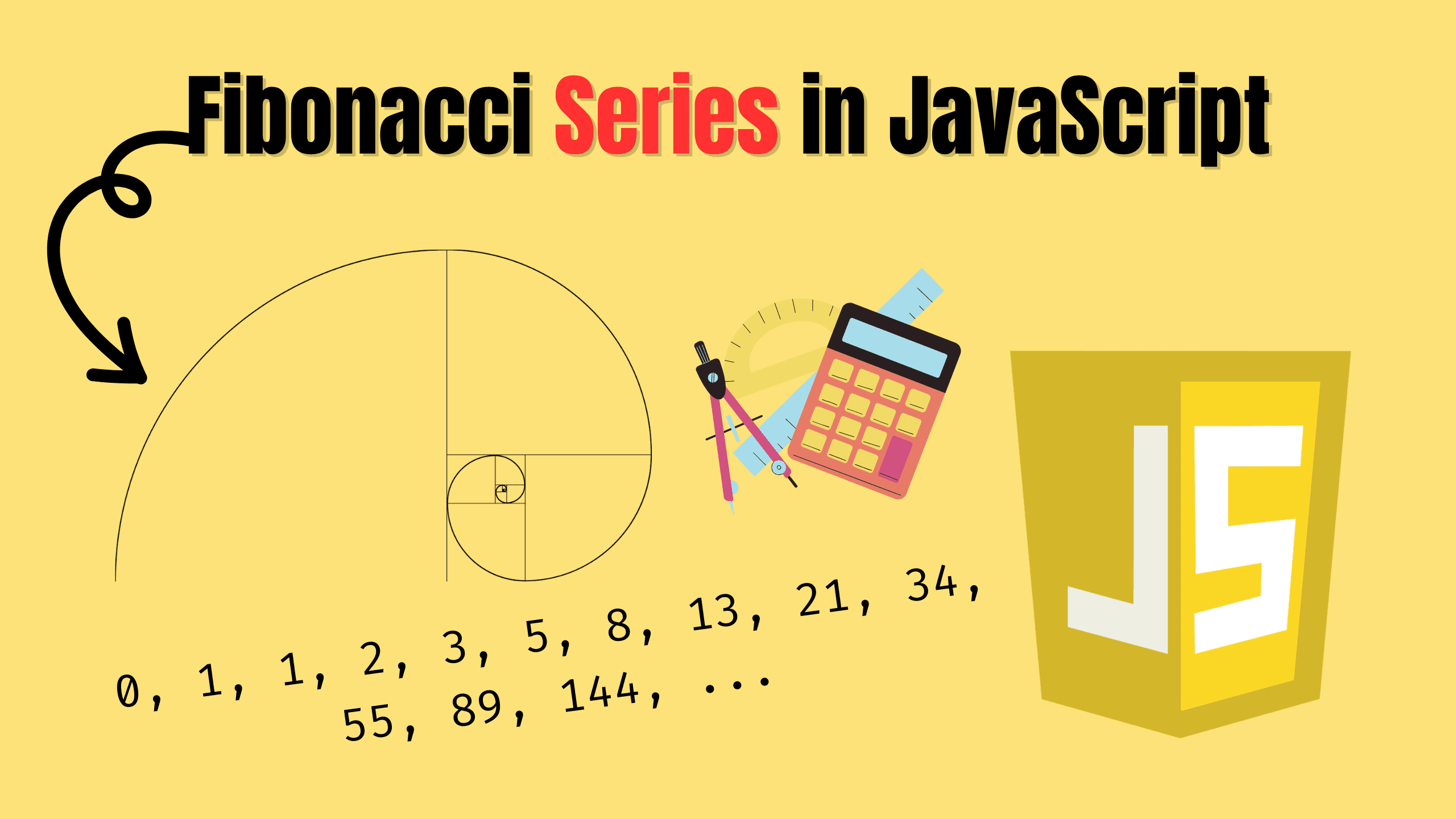
In mathematics, the Fibonacci sequence is a sequence in which each element is the sum of the two elements that precede it. Numbers that are part of the Fibonacci sequence are known as Fibonacci numbers. Many writers begin the sequence with 0 and 1, although some authors start it from 1 and 1 and some (as did Fibonacci) from 1 and 2. Starting from 0 and 1, the sequence begins
js0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, ... (sequence A000045 in the OEIS)
The Fibonacci numbers were first described in Indian mathematics as early as 200 BC in work by Pingala on enumerating possible patterns of Sanskrit poetry formed from syllables of two lengths. They are named after the Italian mathematician Leonardo of Pisa, also known as Fibonacci, who introduced the sequence to Western European mathematics in his 1202 book Liber Abaci.
Fibonacci numbers are not just theoretical; they play a key role in:
- Dynamic programming and algorithms
- Data structures
- Financial modeling
- Artificial intelligence
Moreover, these numbers are evident in nature, from the arrangement of leaves on a plant to the spiral pattern of a seashell.
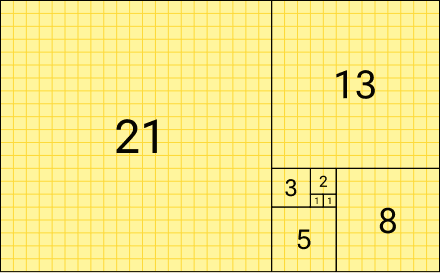
In this blog, we will explore different ways to generate the Fibonacci series using JavaScript, comparing their efficiency and practicality. We will also discuss real-world applications of Fibonacci numbers and their importance in problem-solving.
Understanding the Fibonacci Sequence
The Fibonacci sequence is defined mathematically as:
jsF(n) = F(n-1) + F(n-2), where n > 1 F(0) = 0, F(1) = 1
This recurrence relation means that each term is derived from the sum of the previous two terms. This simple yet powerful property makes the Fibonacci sequence an essential concept in various fields of science and engineering.
Now, let's dive into different JavaScript implementations of Fibonacci numbers.
1. Fibonacci Series Using Iteration
The iterative method is one of the most efficient ways to build the Fibonacci series. By using a simple loop, we avoid the pitfalls of recursion—such as stack overflows and redundant calculations.
jsfunction fibonacciIterative(n) { if (n <= 0) return []; if (n === 1) return [0]; let fibSeries = [0, 1]; for (let i = 2; i < n; i++) { fibSeries.push(fibSeries[i - 1] + fibSeries[i - 2]); } return fibSeries; } console.log(fibonacciIterative(10));
If n is 0 or 1, we handle those cases separately by returning either an empty array or an array containing only 0. For larger values of n, we start with an initial array [0, 1], which represents the first two Fibonacci numbers. We then use a loop that iterates from index 2 to n-1, calculating each subsequent Fibonacci number by summing the two preceding numbers in the array. These calculated numbers are appended to the array to construct the sequence iteratively. Finally, the function returns the complete Fibonacci sequence up to n elements, ensuring efficiency and avoiding the drawbacks of recursion.
2. Fibonacci Series Using Recursion
Recursion provides a clear and mathematical way to define the Fibonacci sequence. However, the naive recursive approach suffers from exponential time complexity due to overlapping subproblems.
javascriptfunction fibonacciRecursive(n) { if (n <= 1) { return n; } return fibonacciRecursive(n - 1) + fibonacciRecursive(n - 2); } let fibSequence = []; for (let i = 0; i < 10; i++) { fibSequence.push(fibonacciRecursive(i)); } console.log(fibSequence);
The function uses recursion to compute Fibonacci numbers by repeatedly calling itself with smaller values of n. When n is either 0 or 1, the function directly returns n, serving as the base case to terminate the recursion. For larger values of n, the function makes two recursive calls, calculating fibonacciRecursive(n - 1) and fibonacciRecursive(n - 2), then summing the results to determine the Fibonacci number at n. This process continues until all recursive calls reach the base cases, at which point the results are aggregated and returned.
3. Optimized Fibonacci Using Memoization
Memoization optimizes the recursive method by caching previously computed values. This transforms the exponential time complexity into a linear one, making the recursive approach practical for larger sequences.
javascriptfunction fibonacciMemoized() { let cache = {}; return function fib(n) { if (n in cache) { return cache[n]; } if (n <= 1) { return n; } cache[n] = fib(n - 1) + fib(n - 2); return cache[n]; }; } const fibonacci = fibonacciMemoized(); let memoizedFibSequence = []; for (let i = 0; i < 10; i++) { memoizedFibSequence.push(fibonacci(i)); } console.log(memoizedFibSequence);
To optimize the recursive approach, we use memoization, which involves storing previously computed Fibonacci numbers in an object called cache. Whenever the function is called with a particular n, it first checks if the value is already present in the cache. If it is, the function returns the cached value immediately, avoiding redundant calculations. If not, it computes the Fibonacci number recursively and stores the result in the cache before returning it. This drastically improves performance by eliminating repeated function calls and reducing the exponential time complexity of the naive recursive approach to a much more efficient linear complexity.
4. Fibonacci Using a Generator Function
Generator functions in JavaScript provide a memory-efficient way to generate Fibonacci numbers on demand. Instead of calculating the entire sequence upfront, generators yield one number at a time.
jsfunction* fibonacciGenerator() { let a = 0, b = 1; while (true) { yield a; [a, b] = [b, a + b]; } } const gen = fibonacciGenerator(); console.log([...Array(10)].map(() => gen.next().value));
The function* syntax is used to create a generator function, which allows for pausing and resuming execution. Within the function, the yield statement is used to produce values one at a time, meaning each time the generator function is called, it returns the next value in the sequence without computing all values upfront. This approach is particularly useful for generating large or infinite sequences efficiently, as it maintains internal state between calls and only computes values when needed. As a result, generator functions offer a memory-efficient solution compared to storing the entire sequence in an array, making them ideal for handling Fibonacci numbers in scenarios requiring dynamic value generation.
Performance Comparison
Each method has its advantages and use cases. Below is a comparison:
Method | Time Complexity | Space Complexity | Notes |
---|---|---|---|
Iterative | O(n) | O(n) | Efficient and simple |
Recursive | O(2^n) | O(n) | Slow for large n |
Memoization | O(n) | O(n) | Optimized recursion |
Generator | O(n) | O(1) | Generates values on demand |
Conclusion
The Fibonacci sequence is a fundamental concept in programming with various implementation strategies in JavaScript. If performance is a concern:
- Use the iterative approach for efficiency and simplicity.
- Use memoization to optimize recursion.
- Use generators when working with large or infinite sequences.
Each method has its place depending on the problem context. I hope this guide helps you understand and implement Fibonacci sequences effectively in JavaScript. Which approach do you prefer? Let me know in the comments!
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!