How to Check if a Key Exists in an Object in JavaScript
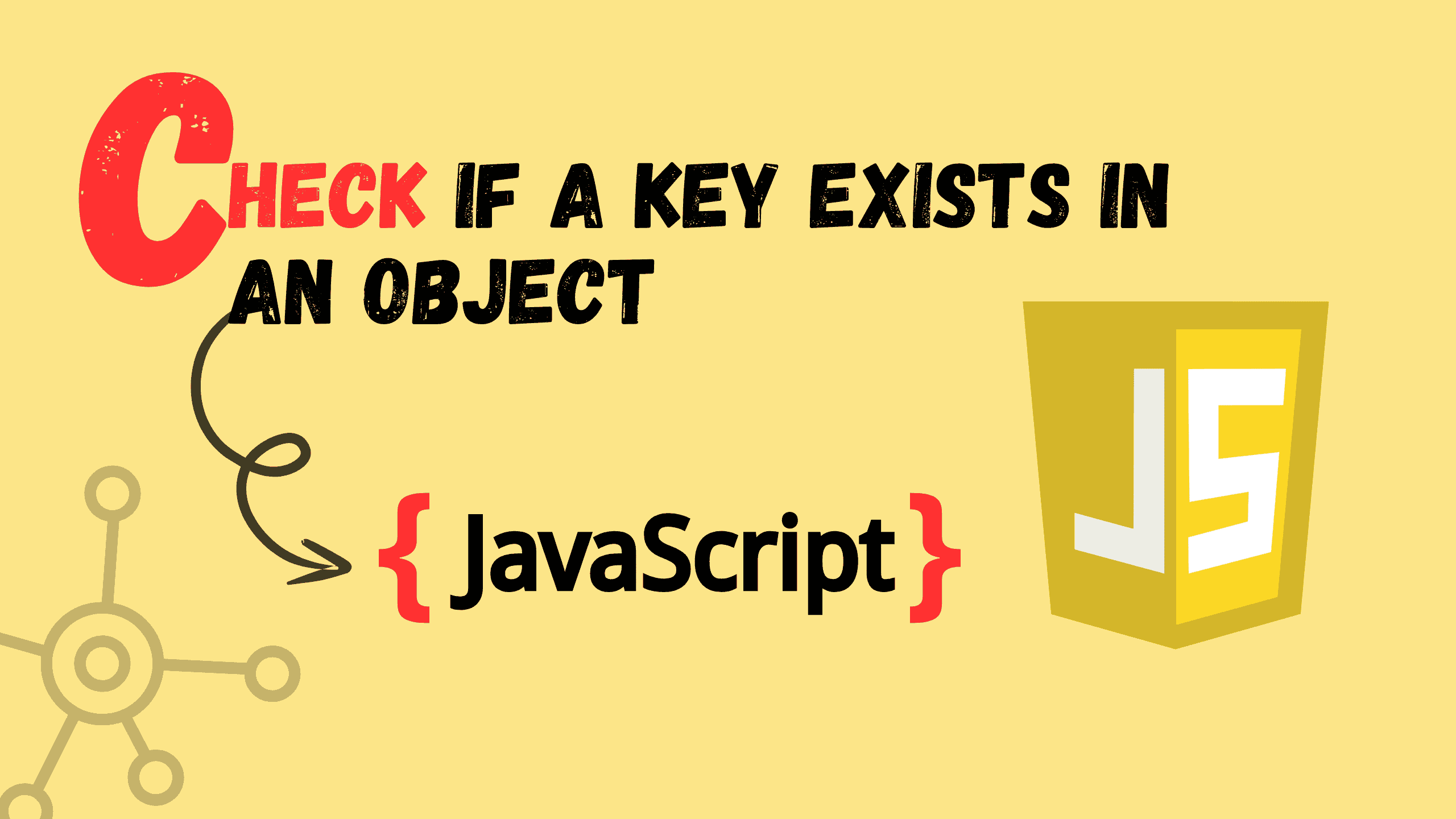
Have you ever been knee-deep in JavaScript code and wondered, “How do I check if a key exists in an object?” You’re not alone! It’s a common question that arises when working with JavaScript objects, and trust me, it’s easier than you might think.
But before we dive into the “how,” let’s take a step back and understand what an object is in JavaScript.
What is an Object?
In JavaScript, an object is a complex data type that allows you to store collections of key-value pairs. Objects are foundational to JavaScript programming because they represent real-world entities and data structures in a flexible and dynamic way.
Objects store data in the form of properties, where each property is a combination of a key (or name) and its associated value.
- Keys are usually strings or symbols.
- Values can be any valid JavaScript data type, including numbers, strings, arrays, functions, or even other objects.
jslet person = { name: "John", age: 30, isEmployed: true };
- name, age, and isEmployed are the keys.
- "John", 30, and true are the corresponding values.
Objects are everywhere in JavaScript, and checking if a key exists is a basic skill that can save you from many headaches.
Why Do You Need to Check If a Key Exists?
Imagine you’re building an app that relies on user input or API data. Sometimes, a key you’re looking for might not exist in the object you’re working with. If you try to access it without checking, you might end up with undefined or even an error.
Here’s how you can avoid those nasty surprises.
Different Ways to Check if a Key Exists
JavaScript offers multiple ways to check if a key exists in an object. Let’s explore them one by one.
1. Using the in Operator
The in operator is a straightforward way to check if a key exists in an object.
jsconst car = { brand: 'Toyota', model: 'Corolla', year: 2021, }; console.log('brand' in car); // true console.log('color' in car); // false
The in operator returns true if the key exists, even if its value is null or undefined.
Why use it? It’s quick and easy, and it works with inherited properties too (something to keep in mind!).
2. Using hasOwnProperty()
If you want to check only the keys that are directly on the object (not inherited), hasOwnProperty() is your go-to method.
jsconsole.log(car.hasOwnProperty('brand')); // true console.log(car.hasOwnProperty('toString')); // false
Pro Tip: Use hasOwnProperty() when you’re working with objects that may have inherited properties, like when extending prototypes.
3. Accessing the Key Directly (with a Twist)
You can also access the key directly and check if it’s undefined.
jsconsole.log(car.brand !== undefined); // true console.log(car.color !== undefined); // false
But wait — be cautious! Sometimes a key might exist with the value undefined. In such cases, this method won’t be reliable.
jsconst car = { brand: undefined }; console.log(car.brand !== undefined); // false (even though 'brand' exists)
4. Using Object.hasOwn() (Modern Approach)
If you’re using a modern JavaScript environment (ES2022+), there’s a shiny new method: Object.hasOwn().
This method works similarly to hasOwnProperty() but in a more concise way.
jsconsole.log(Object.hasOwn(car, 'brand')); // true console.log(Object.hasOwn(car, 'color')); // false
Why use it? It’s simpler and future-proof! If you’re working with the latest JavaScript, this is your best option.
Conclusion
Checking if a key exists in a JavaScript object isn’t rocket science, but picking the right method can make your code cleaner, faster, and easier to debug. Experiment with these approaches, and you’ll soon have a favorite! Do you have a go-to method for checking keys in objects? Happy Coding!!!
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!