HTML Parser In Python - A Comprehensive Guide
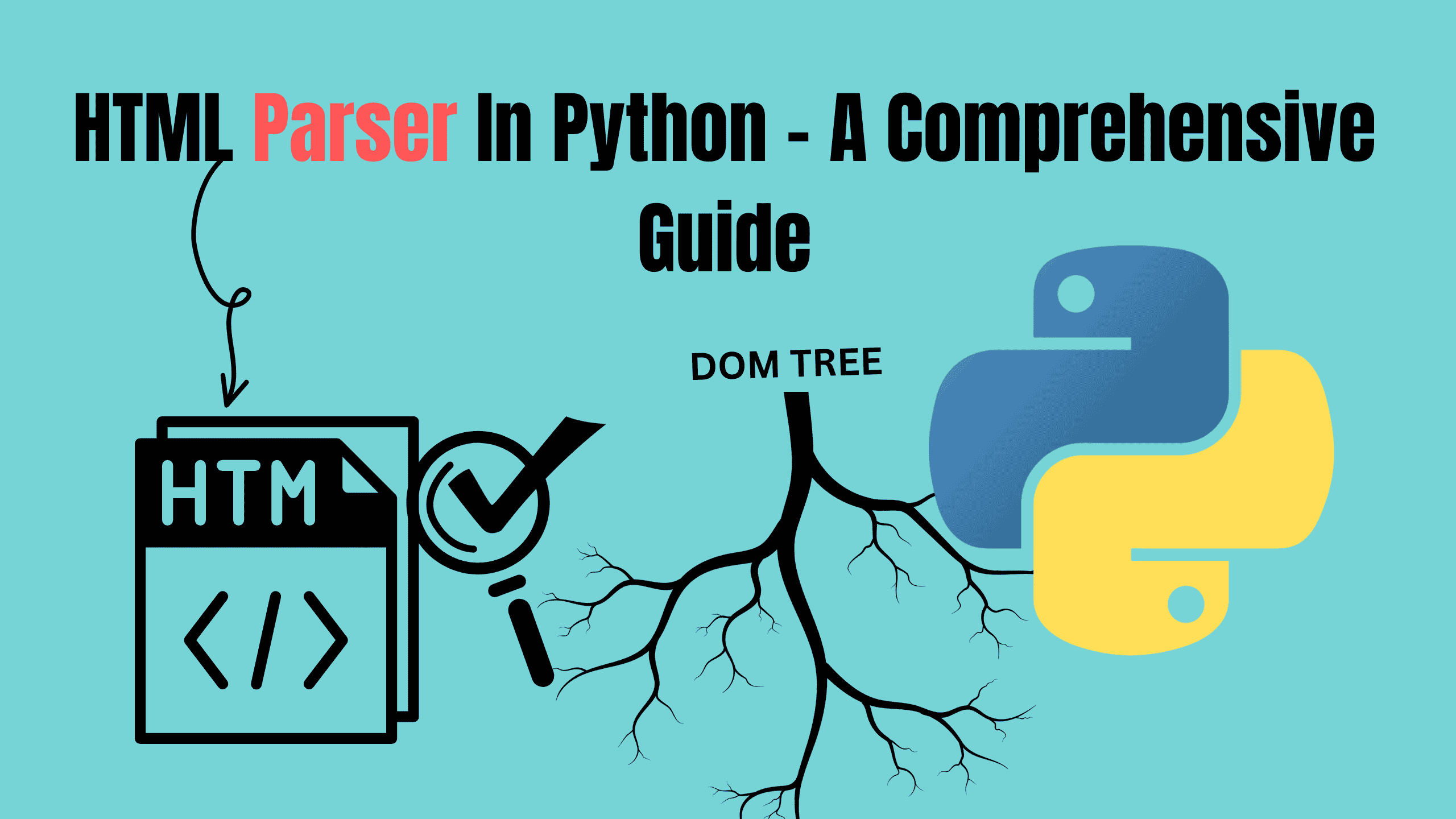
In the digital age, data is abundant, often encapsulated within the intricate structures of web pages. To harness this data effectively, understanding HTML parser in python is essential. In this guide, we'll delve into the fundamentals of HTML, demystify the concept of parsing, and explore three robust methods to parse HTML in Python: Beautiful Soup, lxml, and the standard library's html.parser.
What is HTML?
HTML (HyperText Markup Language) is the standard language for creating web pages. It structures content using elements denoted by tags, such as head, title, body, p, and a. These tags define the layout and organization of web content, enabling browsers to render pages appropriately.
What Does Parsing Mean?
Parsing involves analyzing a string of symbols (in this case, HTML) to understand its grammatical structure. In the context of web development, parsing HTML means programmatically dissecting the HTML content to extract or manipulate specific data elements.
Methods to Parse HTML in Python
Python offers several libraries for HTML parsing, each with unique features and advantages. Let's explore three prominent methods:
1. Beautiful Soup
BeautifulSoup is a widely-used Python library for parsing HTML and XML documents. It creates a parse tree from page source code, enabling easy data extraction.
Installation:
Before using Beautiful Soup, ensure it's installed:
bashpip install beautifulsoup4
Usage Example:
Suppose we have an HTML snippet and using Beautiful Soup to parse and extract data:
pyfrom bs4 import BeautifulSoup html_doc = """ <html> <head> <title>Sample Page</title> </head> <body> <p class="content">Welcome to web scraping with Beautiful Soup!</p> <a href="http://example.com/page1" class="link">Page 1</a> <a href="http://example.com/page2" class="link">Page 2</a> </body> </html> """ soup = BeautifulSoup(html_doc, 'html.parser') # Extracting the title title = soup.title.string print(f"Page Title: {title}") # Extracting the paragraph text content = soup.find('p', class_='content').text print(f"Content: {content}") # Extracting all links links = soup.find_all('a', class_='link') for link in links: print(f"Link Text: {link.text}, URL: {link['href']}")
Output using Beautiful Soup:
Page Title: Sample Page
Content: Welcome to web scraping with Beautiful Soup!
Link Text: Page 1, URL: http://example.com/page1
Link Text: Page 2, URL: http://example.com/page2
In this example, we:
- Initialized Beautiful Soup with the HTML content.
- Extracted the page title using soup.title.string.
- Retrieved the paragraph text with soup.find('p', class_='content').text.
- Collected all anchor tags with the class 'link' and printed their text and URLs.
2. lxml
lxml is a powerful and high-performance library for processing XML and HTML in Python. It provides safe and convenient access to these documents, offering both ease of use and speed.
Installation:
Install lxml using pip:
bashpip install lxml
Usage Example:
Suppose we have the same HTML snippet and using lxml:
pyfrom lxml import html html_doc = """ <html> <head> <title>Sample Page</title> </head> <body> <p class="content">Welcome to web scraping with lxml!</p> <a href="http://example.com/page1" class="link">Page 1</a> <a href="http://example.com/page2" class="link">Page 2</a> </body> </html> """ tree = html.fromstring(html_doc) # Extracting the title title = tree.findtext('.//title') print(f"Page Title: {title}") # Extracting the paragraph text content = tree.xpath('//p[@class="content"]/text()')[0] print(f"Content: {content}") # Extracting all links links = tree.xpath('//a[@class="link"]') for link in links: link_text = link.text_content() link_url = link.get('href') print(f"Link Text: {link_text}, URL: {link_url}")
Output using lxml:
Page Title: Sample Page
Content: Welcome to web scraping with lxml!
Link Text: Page 1, URL: http://example.com/page1
Link Text: Page 2, URL: http://example.com/page2
In this example, we:
- Parsed the HTML content using html.fromstring().
- Extracted the title with tree.findtext().
- Retrieved the paragraph text using XPath: tree.xpath('//p[@class="content"]/text()')[0].
- Collected all anchor tags with the class 'link' and printed their text and URLs using XPath.
3. html.parser (Standard Library)
Python's built-in html.parser module is a lightweight and straightforward option for parsing HTML. While it may lack some of the advanced features and performance of third-party libraries like Beautiful Soup or lxml, it’s an excellent choice for basic parsing tasks, especially when you want to avoid external dependencies.
Usage Example:
Suppose we have the same HTML snippet and using the html.parser module:
pyfrom html.parser import HTMLParser class MyHTMLParser(HTMLParser): def __init__(self): super().__init__() self.title = None self.in_title = False self.links = [] self.in_paragraph = False self.paragraph_content = "" def handle_starttag(self, tag, attrs): if tag == "title": self.in_title = True if tag == "p": self.in_paragraph = True if tag == "a": href = dict(attrs).get("href", None) if href: self.links.append((self.get_starttag_text(), href)) def handle_endtag(self, tag): if tag == "title": self.in_title = False if tag == "p": self.in_paragraph = False def handle_data(self, data): if self.in_title: self.title = data if self.in_paragraph: self.paragraph_content += data html_doc = """ <html> <head> <title>Sample Page</title> </head> <body> <p class="content">Welcome to web scraping with html.parser!</p> <a href="http://example.com/page1" class="link">Page 1</a> <a href="http://example.com/page2" class="link">Page 2</a> </body> </html> """ parser = MyHTMLParser() parser.feed(html_doc) # Extracting the title print(f"Page Title: {parser.title}") # Extracting the paragraph content print(f"Content: {parser.paragraph_content}") # Extracting links for link_text, link_url in parser.links: print(f"Link Text: {link_text}, URL: {link_url}")
Output using html.parser:
Page Title: Sample Page
Content: Welcome to web scraping with html.parser!
Link Text: <a href="http://example.com/page1" class="link">Page 1</a>, URL: http://example.com/page1
Link Text: <a href="http://example.com/page2" class="link">Page 2</a>, URL: http://example.com/page2
In this example, we:
- Extended the HTMLParser class to define custom behavior for start tags, end tags, and data.
- Captured a title tag and its content in the parser.
- Fetched the data of p tag using the handle_data method.
- Extracted the all a tags with class 'link' and printed their text and URLs.
This method offers fine-grained control over the parsing process but requires more manual effort compared to the other methods.
Comparing the Methods
Python offers three excellent HTML parsing methods, each suited for different needs:
Feature | Ease of Use | Performance Good | Handling Malformed HTML | Dependencies | Best Use Case |
---|---|---|---|---|---|
Beautiful Soup | Very High | Excellent | Excellent | Third-Party Library | Flexible and user-friendly parsing for general tasks. |
lxml | Moderate | Good | Good | Third-Party Library | High-performance parsing, particularly for XML-heavy tasks. |
html.parser (Standard Library) | Moderate | Good | Basic | Third-Party Library | Lightweight parsing with no external dependencies. |
Conclusion
HTML parsing is a vital skill for developers working with web data. Python offers versatile tools to achieve this, ranging from Beautiful Soup's user-friendly interface, lxml's high performance, to the standard library's lightweight html.parser. Each has its strengths, and the choice depends on your project requirements. Armed with this knowledge, you’re well-equipped to dive into the world of web scraping and data extraction.
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!