JavaScript Sleep Function - A Comprehensive Guide
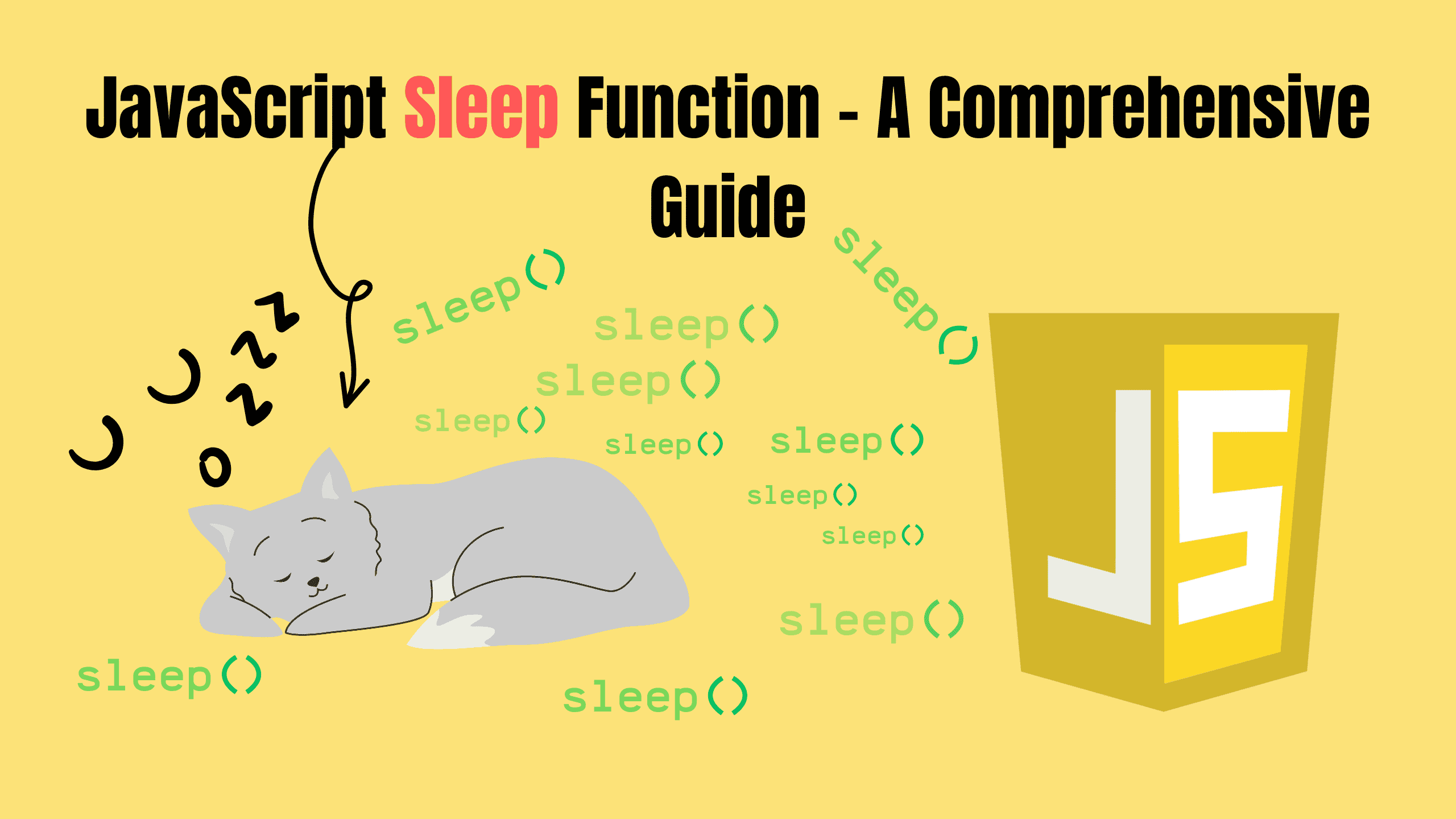
Imagine you’re working on a JavaScript project, and you realize you need a delay between certain operations. Maybe it’s for debugging, animations, or even simulating API latency. You eagerly type "JavaScript sleep" into your editor and hit run, only to find… nothing happens.
That’s because JavaScript doesn’t have a built-in sleep function.
But don’t worry! In this guide, we’ll explore how to implement sleep functionality in JavaScript, delve into advanced patterns, and look at when (and when not) to use it. By the end, you’ll know all about using sleep in JavaScript, and more importantly, when to reach for it.
Why Doesn’t JavaScript Have a Native Sleep Function?
The absence of a sleep function isn’t an oversight—it’s by design. JavaScript operates in a single-threaded environment. In plain terms, this means only one piece of code runs at a time.
If JavaScript had a blocking sleep() function, it could pause the thread entirely. No animations. No user interactions. No network calls. Nothing. Your entire application would grind to a halt during that time. That’s bad news for user experience.
Instead, JavaScript emphasizes non-blocking code, encouraging the use of asynchronous programming with tools like promises, async/await, and callbacks. This design ensures that even if one task is waiting, the rest of your app keeps working.
How to Implement Sleep in JavaScript
Now that we know why JavaScript doesn’t have a built-in sleep function, let’s explore how to simulate it.
1. The Asynchronous Way: Using setTimeout
The simplest way to achieve a delay in JavaScript is by combining the setTimeout function with Promises. This method is non-blocking and works perfectly for most use cases.
Here’s how to create a reusable sleep function in JavaScript:
jsfunction sleep(ms) { return new Promise(resolve => setTimeout(resolve, ms)); } async function demoSleep() { console.log('Starting...'); await sleep(2000); // Sleep for 2 seconds console.log('2 seconds later...'); } demoSleep();
This approach is clean and leverages the power of promises and async/await. The await keyword pauses the execution of the demoSleep function until the sleep promise resolves.
Why This Works
- Non-blocking: The rest of your application remains responsive.
- Modern and readable: Using async/await makes your code more intuitive compared to callback-heavy alternatives.
2. The Synchronous Way: Busy-Wait Loop
If you need a true blocking sleep function in JavaScript (for example, in a Node.js script), you can use a busy-wait loop.
jsfunction sleepSync(ms) { const end = Date.now() + ms; while (Date.now() < end) { // Loop until the time has passed } } console.log('Starting...'); sleepSync(2000); // Sleep for 2 seconds console.log('2 seconds later...');
Warning: This method freezes the thread entirely. Avoid using it in browser environments, as it blocks everything from animations to user input.
Advanced Patterns with JavaScript Sleep
Now that you understand the basics of implementing sleep in JavaScript, it’s time to explore some more advanced use cases where sleep functions can truly shine. While the simple use of sleep() in standalone operations can be useful, there are many scenarios where adding delays within complex logic or asynchronous workflows becomes essential. These advanced patterns allow you to handle intricate timing issues, improve the user experience, or simulate real-world delays for testing and debugging purposes.
1. Delaying Inside Loops
Have you ever needed to pause execution inside a loop? Here’s how to achieve it with an asynchronous sleep function:
jsasync function delayedLoop() { for (let i = 1; i <= 5; i++) { console.log(`Step ${i}`); await sleep(1000); // Sleep for 1 second } console.log('Loop complete!'); } delayedLoop();
Each iteration of the loop pauses for 1 second before proceeding, ensuring a sequential delay. This is perfect for timed operations or animations.
2. Simulating API Latency
When testing applications, you might need to simulate slow API responses. Sleep functions in JavaScript are great for this purpose.
jsasync function fakeApiCall() { console.log('Fetching data...'); await sleep(1500); // Simulate a 1.5-second delay console.log('Data received!'); } fakeApiCall();
This is a common trick for debugging or testing timeout scenarios in applications.
3. Retry Logic with Delays
Retrying failed operations is a common pattern in applications. Adding a delay between retries can prevent overwhelming the server or user experience.
jsasync function retryOperation(maxRetries, delay) { for (let attempt = 1; attempt <= maxRetries; attempt++) { try { console.log(`Attempt ${attempt}`); if (Math.random() < 0.7) throw new Error('Failed'); console.log('Success!'); return; } catch (err) { console.error(err.message); if (attempt < maxRetries) { console.log(`Retrying in ${delay}ms...`); await sleep(delay); } else { console.log('All attempts failed.'); } } } } retryOperation(3, 2000);
4. Animations with Delays
Creating timed animations is another practical use case for sleep functions in JavaScript.
jsasync function animateSequence() { const messages = ['Step 1', 'Step 2', 'Step 3']; for (const msg of messages) { console.log(msg); await sleep(1000); } console.log('Animation complete!'); } animateSequence();
Frequently Asked Questions
1. How to Sleep in JavaScript for 5 Seconds?
To implement a sleep function that delays execution for 5 seconds, you can use the sleep() function in combination with async/await. Here's how you do it:
jsawait sleep(5000); // Sleep for 5000 milliseconds, which equals 5 seconds
This will pause the execution of your function for 5 seconds before moving on to the next line of code. Using the await keyword ensures that the rest of the program doesn't execute until the sleep function has completed.
2. Can You Sleep in JavaScript Without async/await?
Yes, while async/await is the most readable and modern way to implement delays, you can achieve a similar effect using callbacks with setTimeout. Here’s an example:
jsfunction sleepCallback(ms, callback) { setTimeout(callback, ms); } sleepCallback(2000, () => console.log('2 seconds later...')); // Wait for 2 seconds
In this case, the code doesn’t block execution but instead sets a timer for the callback to be executed after 2 seconds. It’s a simple approach but less intuitive than async/await.
3. How Can I Implement Sleep in a Loop?
One common use case is implementing a sleep function inside a loop. This is useful when you want to add delays between iterations of the loop. Here’s an example using the await keyword:
jsasync function delayedLoop() { for (let i = 1; i <= 5; i++) { console.log(`Step ${i}`); await sleep(1000); // Sleep for 1 second between steps } console.log('Loop complete!'); } delayedLoop();
This ensures that each step of the loop pauses for 1 second, making it perfect for scenarios like timed animations or sequential tasks.
4. Can I Use sleep() in Browser-Side JavaScript?
Yes, you can use sleep() in browser-side JavaScript, but you need to be mindful that using sleep too frequently or for long periods can block UI updates and affect the user experience. It's better to use asynchronous solutions like await sleep() to ensure the browser doesn’t freeze while waiting.
jsasync function simulateDelay() { console.log('Waiting...'); await sleep(3000); // Sleep for 3 seconds console.log('3 seconds passed!'); } simulateDelay();
This works just as well as in Node.js because modern browsers fully support the async/await syntax.
5. How Do I Create a Sleep Function with Different Time Intervals?
You can easily customize the sleep function to sleep for different time intervals by passing the desired duration (in milliseconds) as a parameter. For instance, to sleep for 3 seconds, you would call await sleep(3000).
jsasync function sleep(ms) { return new Promise(resolve => setTimeout(resolve, ms)); } async function sleepDifferentTimes() { console.log('Wait for 1 second'); await sleep(1000); // Sleep for 1 second console.log('Wait for 3 seconds'); await sleep(3000); // Sleep for 3 seconds console.log('Wait for 5 seconds'); await sleep(5000); // Sleep for 5 seconds } sleepDifferentTimes();
This is a flexible and simple way to control the timing in your JavaScript applications.
Things to Watch Out For
- Misusing Synchronous Sleep: Avoid using busy-wait loops unless absolutely necessary, as they block the entire thread.
- Excessive Delays: Adding unnecessary delays can make your application feel slow and unresponsive.
- Debugging Sleep Issues: Always document why you’ve added a sleep function, so future developers (or even future you) understand its purpose.
Conclusion
While JavaScript lacks a native sleep() function, the techniques we’ve covered offer plenty of ways to achieve the same effect. From non-blocking delays using setTimeout to advanced retry logic, you now have all the tools you need to use sleep in JavaScript effectively.
So, the next time you need to pause execution, remember these approaches.
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!