How to reverse a string in JavaScript
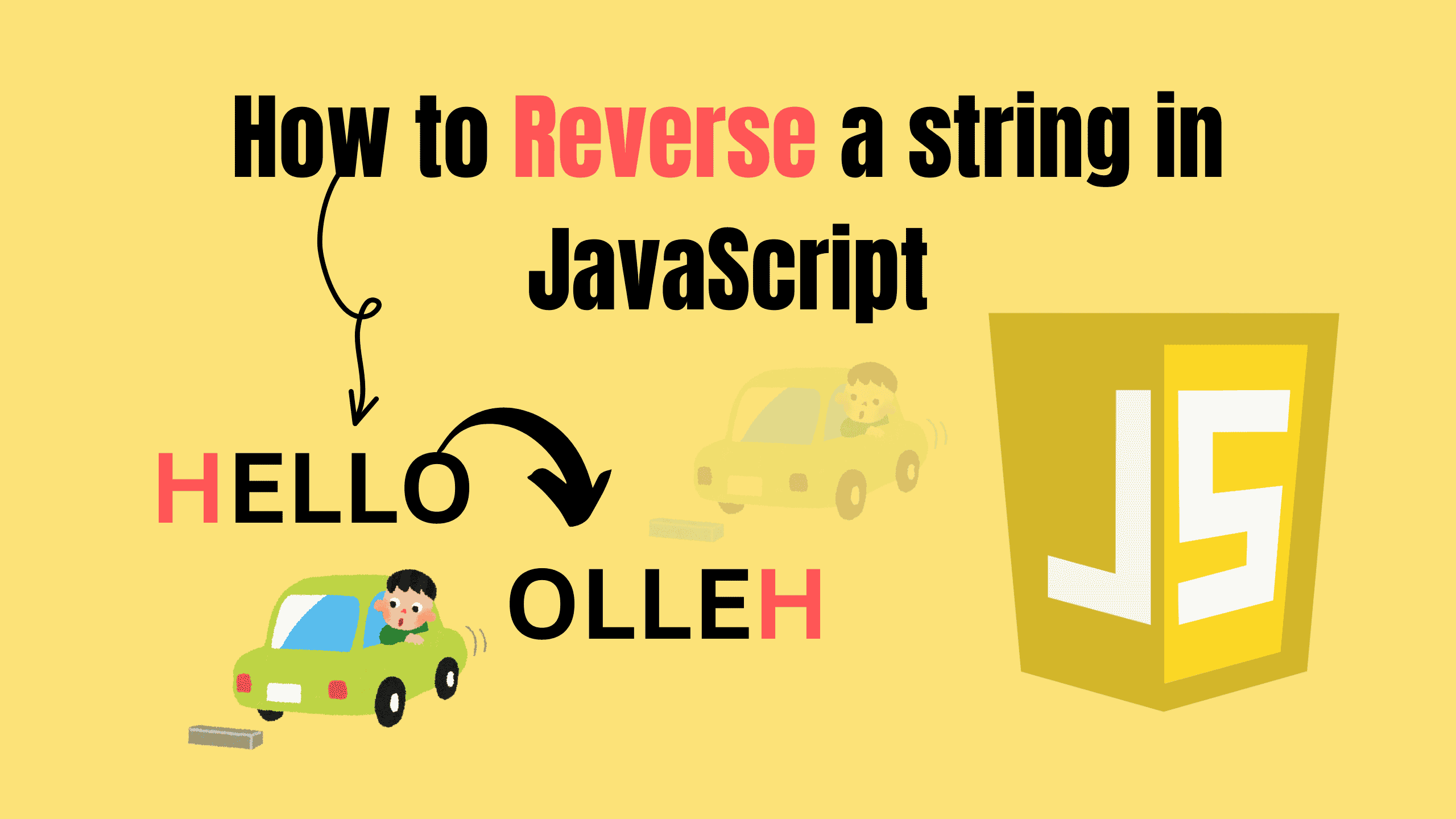
Reversing a string is one of the most common coding tasks that programmers encounter, especially when learning new programming languages. In JavaScript, this problem can be tackled in several ways. Whether you are a beginner trying to understand basic concepts or an experienced developer looking for optimized methods, this blog will provide all the information you need to reverse a string in JavaScript.
In this blog, we will cover:
- Why Reverse a String?
- Understanding of Strings in JavaScript
- Different Methods to Reverse a String
- Performance Considerations
- FAQs
- Conclusion
By the end of this blog, you’ll not only know how to reverse a string but also understand which method to use and why. Let's focuses on popular methods to reverse like inbuilt .reverse() function or for loop or recursion.
Why Reverse a String?
Before diving into the methods, let’s consider why you might need to reverse a string:
- Algorithmic Challenges: String reversal is a popular problem in coding interviews and competitive programming.
- Data Manipulation: In certain cases, reversing strings can be used to process data in specific formats.
- Learning Exercise: It helps beginners understand string manipulation and working with JavaScript methods like arrays, loops, and recursion.
Understanding of Strings in JavaScript
A string in JavaScript is a sequence of characters. Strings are immutable, meaning once a string is created, it cannot be changed. Instead, operations like reversal create a new string.
jslet originalString = "hello"; // Strings are immutable, so methods like substring or slice return new strings. let newString = originalString.slice(0, 3); // "hel"
Understanding this concept is essential because any method to reverse a string will involve creating a new string.
Different Methods to Reverse a String
There are several ways to reverse a string in javascript. The most common methods include using loops, recursion, and built-in functions or libraries.
1. Using Built-In Methods
Using built-in methods is the simplest and most common way to reverse a string in JavaScript. This approach involves three main steps:
- Convert the String to an Array: The split('') method breaks the string into an array of individual characters. For example, "hello" becomes ['h', 'e', 'l', 'l', 'o'].
- Reverse the Array: The reverse() method reorders the elements of the array from the last to the first.
- Join the Array Back to a String: The join('') method combines the elements of the array back into a single string.
jsfunction reverseString(str) { return str.split('').reverse().join(''); } console.log(reverseString("hello")); // Output: "olleh"
This method is concise, easy to read, and suitable for most use cases. However, it does involve creating an intermediate array, which could impact performance for very large strings.
2. Using a For Loop
A for loop is a more manual way to reverse a string. By iterating through the string from the end to the beginning, you can build the reversed string character by character.
Here are the steps:
- Initialize an empty string to store the reversed string.
- Use a for loop to iterate over the string starting from the last character.
- Append each character to the new string during each iteration.
jsfunction reverseString(str) { let reversed = ""; for (let i = str.length - 1; i >= 0; i--) { reversed += str[i]; } return reversed; } console.log(reverseString("hello")); // Output: "olleh"
This method provides a clear and step-by-step way to reverse a string, making it ideal for understanding the mechanics of loops.
3. Using Recursion
Recursion reverses a string by repeatedly breaking it into smaller parts and combining the results. It’s a concept where a function calls itself to solve a smaller instance of the problem.
Here are the steps:
- Define the base case. If the string is empty or has only one character, return it as it is already reversed.
- Slice the string to remove the first character and call the function on the remaining string.
- Append the removed character to the result of the recursive call.
jsfunction reverseString(str) { if (str === "") return ""; return reverseString(str.slice(1)) + str[0]; } console.log(reverseString("hello")); // Output: "olleh"
Recursion demonstrates an elegant approach but may not be suitable for very long strings due to the risk of stack overflow.
4. Using the Reduce Method
The reduce method is a functional approach to reversing a string. It works by accumulating a reversed version of the string one character at a time.
Here are the steps:
- Convert the string into an array using split('').
- Use reduce to iterate over each character and prepend it to the accumulated result.
jsfunction reverseString(str) { return str.split('').reduce((reversed, char) => char + reversed, ''); } console.log(reverseString("hello")); // Output: "olleh"
This method is concise but may be less intuitive for beginners unfamiliar with higher-order functions.
5. Using Spread Syntax (ES6+)
The spread syntax (...) provides a modern way to reverse a string by treating it as an array of characters.
Here are the steps:
- Use the spread syntax to convert the string into an array of characters.
- Reverse the array using reverse().
- Join the array back into a string with join('').
jsfunction reverseString(str) { return [...str].reverse().join(''); } console.log(reverseString("hello")); // Output: "olleh"
This method is functionally similar to the split-reverse-join approach but utilizes modern JavaScript syntax.
6. Without Using Built-In Methods
For an approach without any built-in methods, you can reverse a string manually by iterating through it and constructing the reversed version character by character.
Here are the steps:
- Initialize an empty string to store the result.
- Use a loop to traverse each character in the string.
- Add each character to the beginning of the result string.
jsfunction reverseString(str) { let reversed = ""; for (let char of str) { reversed = char + reversed; } return reversed; } console.log(reverseString("hello")); // Output: "olleh"
This approach offers a clear understanding of string reversal without relying on built-in functions.
Performance Considerations
Each method has its own performance characteristics:
- Built-In Methods: split-reverse-join is fast and concise but uses additional memory for the intermediate array.
- For Loop: Efficient and straightforward with minimal memory overhead.
- Recursion: Elegant but can cause stack overflow for large strings.
- Reduce Method: Concise but may have slightly higher overhead due to the use of a higher-order function.
- Spread Syntax: Modern and clean but functionally similar to split-reverse-join.
For most real-world applications, the split-reverse-join method strikes the best balance between simplicity and performance.
FAQs
- Can I reverse a string in-place in JavaScript? No, strings in JavaScript are immutable, which means they cannot be modified after they are created. Any reversal method will create a new string.
- What is the fastest way to reverse a string? The split-reverse-join method is generally considered the fastest for most cases, thanks to JavaScript's optimized implementation of these methods.
- Why does recursion cause stack overflow for large strings? Recursion uses the call stack, and each recursive call adds a new frame to the stack. For very large strings, the stack can exceed its limit, causing an overflow.
- Should I always use built-in methods to reverse a string? It depends on your use case. Built-in methods are concise and easy to use, but exploring other methods like loops and recursion is beneficial for learning and understanding JavaScript.
- Is reversing a string a common real-world task? While reversing strings is not a frequent task in real-world applications, it is an excellent way to practice and understand string manipulation, loops, and recursion.
Conclusion
Reversing a string in JavaScript is a fundamental exercise that can be approached in various ways, each with its own benefits and trade-offs. The built-in split-reverse-join method is quick and straightforward, while loops and recursion offer deeper insights into programming concepts. Understanding the performance characteristics and use cases of these methods will help you choose the best approach for your specific needs. By mastering string reversal, you not only gain a valuable skill but also build a strong foundation in JavaScript programming.
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!