7 most common questions about JavaScript
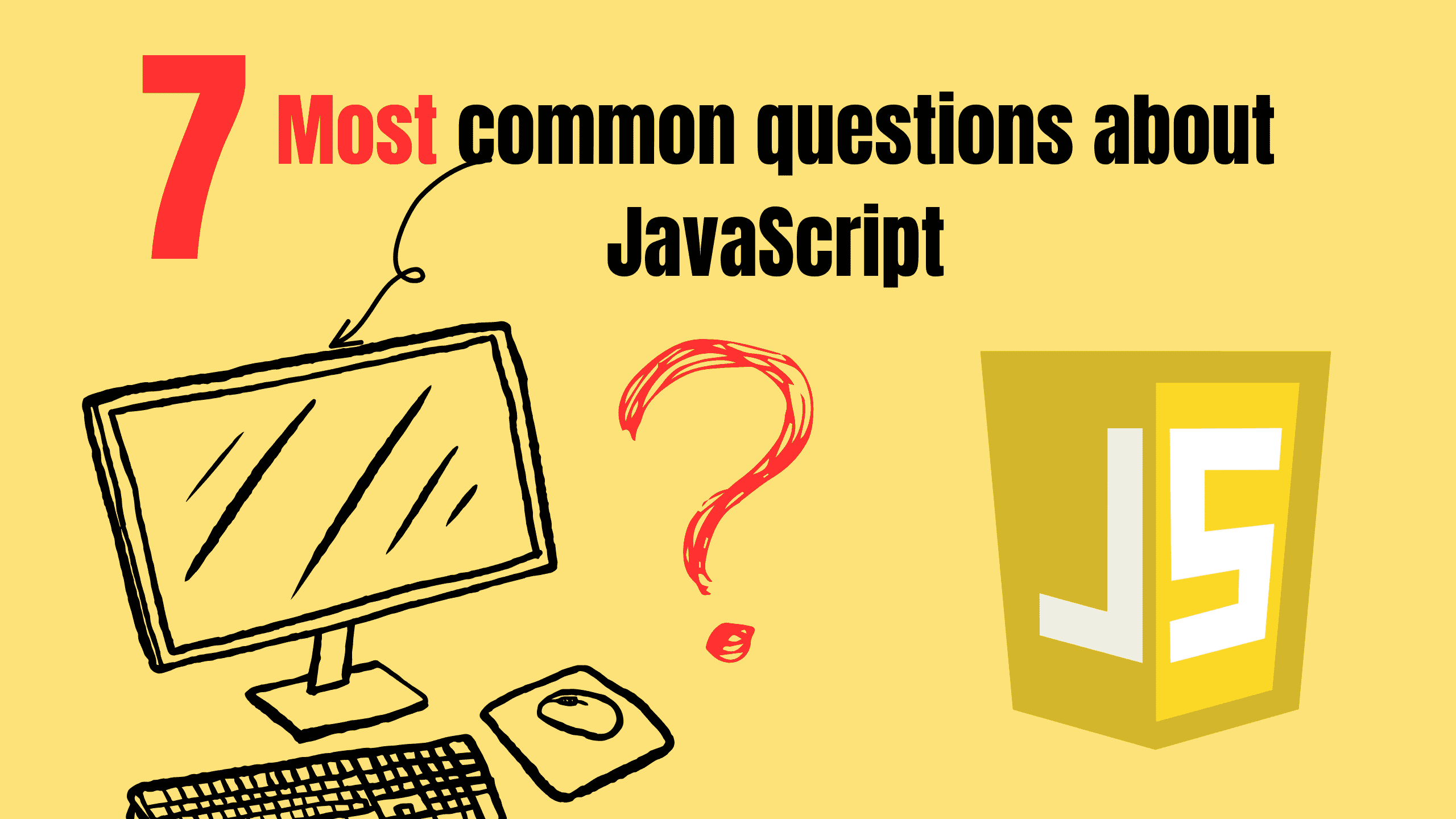
JavaScript is one of the most popular and versatile programming languages in the world today. As a fundamental part of web development, JavaScript powers interactive web pages and applications. However, as with any complex language, developers often have questions, especially when starting out or delving into advanced concepts.
In this blog, you'll get answer of the most common questions about JavaScript, providing clear and concise explanations to help you better understand the language.
- What is JavaScript, and why is it so important?
- How to capitalize first letter in a string javascript?
- How to add attribute to json object in javascript?
- How to separate the digits of a number in javascript?
- How to draw Pacman ghost in javascript?
- How to play a .mp3 file in javascript?
- How to delete a task row in javascript?
1. What is JavaScript, and why is it so important?
JavaScript is a dynamic, high-level, interpreted programming language that is primarily used for creating interactive effects within web browsers. It enables developers to manipulate HTML and CSS, handle events, validate forms, create animations, and even interact with servers without reloading the entire page (thanks to AJAX).
JavaScript is important because:
- Interactivity: Without JavaScript, websites would be static. It allows for dynamic content such as animations, form validation, and much more.
- Versatile: While it's most commonly used for client-side scripting in browsers, JavaScript is also used for server-side development (Node.js), mobile apps (React Native), and even desktop applications.
- Support: Virtually all modern web browsers support JavaScript, making it the standard language for client-side scripting.
2. How to capitalize first letter in a string javascript?
To capitalize the first letter of a string in JavaScript, Use the following code snippet:
jsfunction capitalizeFirstLetter(str) { if (!str) return ""; // Handle empty strings return str.charAt(0).toUpperCase() + str.slice(1); } // Example usage: const input = "hello world"; const output = capitalizeFirstLetter(input); console.log(output); // Output: "Hello world"
Explanation
- str.charAt(0): Gets the first character of the string.
- .toUpperCase(): Converts the first character to uppercase.
- str.slice(1): Gets the rest of the string starting from the second character.
- Concatenation: Combines the uppercase first letter with the rest of the string.
3. How to add attribute to json object in javascript?
To add an attribute (key-value pair) to a JSON object in JavaScript, you can use the dot (.) or bracket ([]) notation.
jslet jsonObject = { name: "John", age: 30 }; // Adding an attribute using dot notation jsonObject.city = "New York"; // Adding an attribute using bracket notation jsonObject["country"] = "USA"; // Adding an dynamic key using a variable let key = "color"; jsonObject[key] = "blue"; console.log(jsonObject); // Output: { name: "John", age: 30, city: "New York", country: "USA" }
Explanation:
- Dot Notation: Use jsonObject.newKey = value; to add a new key. The key must be a valid JavaScript identifier (no spaces or special characters except _ and $).
- Bracket Notation: Use jsonObject["newKey"] = value; to add a key dynamically. This is useful if the key name is stored in a variable or contains spaces or special characters.
4. How to separate the digits of a number in javascript?
To separate the digits of a number in JavaScript, convert the number to a string and then split it into individual characters. Here's how:
i. Using split() after converting to a string
jslet number = 12345; let digits = number.toString().split("").map(Number); console.log(digits); // Output: [1, 2, 3, 4, 5]
Explanation
- toString(): Converts the number to a string so it can be split.
- split(""): Splits the string into an array of individual characters.
- map(Number): Converts each character back to a number.
ii. Using Mathematical Operations
jslet number = 12345; let digits = []; while (number > 0) { digits.unshift(number % 10); // Extract the last digit number = Math.floor(number / 10); // Remove the last digit } console.log(digits); // Output: [1, 2, 3, 4, 5]
Explanation:
- number % 10: Extracts the last digit of the number.
- Math.floor(number / 10): Removes the last digit by integer division.
- digits.unshift(): Adds the digit to the start of the array to preserve the order.
iii. Using ES6 Spread Syntax
jslet number = 12345; let digits = [...number.toString()].map(Number); console.log(digits); // Output: [1, 2, 3, 4, 5]
Explanation:
- [...number.toString()]: Spreads the characters of the string into an array.
- map(Number): Converts the characters back into numbers.
5. How to draw Pacman ghost in javascript?
To draw a Pac-Man ghost in JavaScript, use the HTML5 canvas element along with the CanvasRenderingContext2D API. Here's how can do it:
html<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Pac-Man Ghost</title> </head> <body> <canvas id="pacman-ghost" width="200" height="200"></canvas> <script> const canvas = document.getElementById("pacman-ghost"); const ctx = canvas.getContext("2d"); function drawGhost(x, y, color) { const width = 100; // Ghost width const height = 100; // Ghost height const eyeSize = 10; const pupilSize = 5; // Draw the body ctx.beginPath(); ctx.arc(x + width / 2, y + height / 2, width / 2, Math.PI, 0, false); // Head (top half-circle) ctx.lineTo(x + width, y + height); // Right side const step = width / 8; for (let i = 0; i < 8; i++) { // Bottom zigzag ctx.lineTo(x + width - i * step - step / 2, y + height + (i % 2 === 0 ? 10 : 0)); } ctx.closePath(); ctx.fillStyle = color; ctx.fill(); // Draw eyes ctx.fillStyle = "white"; ctx.beginPath(); ctx.arc(x + width / 3, y + height / 3, eyeSize, 0, 2 * Math.PI); // Left eye ctx.arc(x + (2 * width) / 3, y + height / 3, eyeSize, 0, 2 * Math.PI); // Right eye ctx.fill(); // Draw pupils ctx.fillStyle = "black"; ctx.beginPath(); ctx.arc(x + width / 3, y + height / 3, pupilSize, 0, 2 * Math.PI); // Left pupil ctx.arc(x + (2 * width) / 3, y + height / 3, pupilSize, 0, 2 * Math.PI); // Right pupil ctx.fill(); } // Draw a red ghost at (50, 50) drawGhost(50, 50, "red"); </script> </body> </html>
Explanation:
- Canvas Setup: The canvas element is used to render graphics.
- Drawing the Body: The top part of the ghost is drawn as a half-circle using ctx.arc() and the bottom part is a zigzag pattern created with alternating lineTo() calls.
- Color: Change the ghost’s color by passing a different color value to the drawGhost function.
- Eyes and Pupils: The eyes are white circles (ctx.arc()), and the pupils are smaller black circles.
6. How to play a .mp3 file in javascript?
To play a .mp3 file in JavaScript, use the HTML audio element and the JavaScript Audio API. Here's how:
i. Using audio element
Fetch audio element and play the file directly in JavaScript.
html<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Play MP3</title> </head> <body> <audio id="audioPlayer" src="example.mp3"></audio> <button onclick="playAudio()">Play</button> <button onclick="pauseAudio()">Pause</button> <script> const audio = document.getElementById("audioPlayer"); function playAudio() { audio.play(); // Starts playback } function pauseAudio() { audio.pause(); // Pauses playback } </script> </body> </html>
ii. Using audio Constructor
Dynamically create an audio object and play the file directly in JavaScript.
html<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Play MP3</title> </head> <body> <button onclick="playAudio()">Play</button> <button onclick="pauseAudio()">Pause</button> <script> const audio = new Audio("example.mp3"); function playAudio() { audio.play(); // Starts playback } function pauseAudio() { audio.pause(); // Pauses playback } </script> </body> </html>
7. How to delete a task row in javascript?
To delete a task row in JavaScript, you can use the remove() method or manipulate the DOM to remove the specific row from its parent element. Here's how:
html<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Delete Task Row</title> </head> <body> <table id="taskTable" border="1"> <thead> <tr> <th>Task</th> <th>Action</th> </tr> </thead> <tbody> <tr> <td>Task 1</td> <td><button onclick="deleteRow(this)">Delete</button></td> </tr> <tr> <td>Task 2</td> <td><button onclick="deleteRow(this)">Delete</button></td> </tr> <tr> <td>Task 3</td> <td><button onclick="deleteRow(this)">Delete</button></td> </tr> </tbody> </table> <script> function deleteRow(button) { // Get the parent <tr> of the button const row = button.parentNode.parentNode; // Remove the row from the table row.remove(); } </script> </body> </html>
Explanation
- button.parentNode.parentNode: Traverses the DOM to find the tr containing the button.
- .remove(): Removes the tr element from its parent tbody.
Conclusion
JavaScript is a rich and powerful language, and understanding these common questions will help you build a strong foundation for more advanced concepts. Whether you are just starting out or are already working on complex web applications, a clear understanding of JavaScript’s core concepts will enhance your development experience.
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!