How to ask for user input in the JavaScript Console
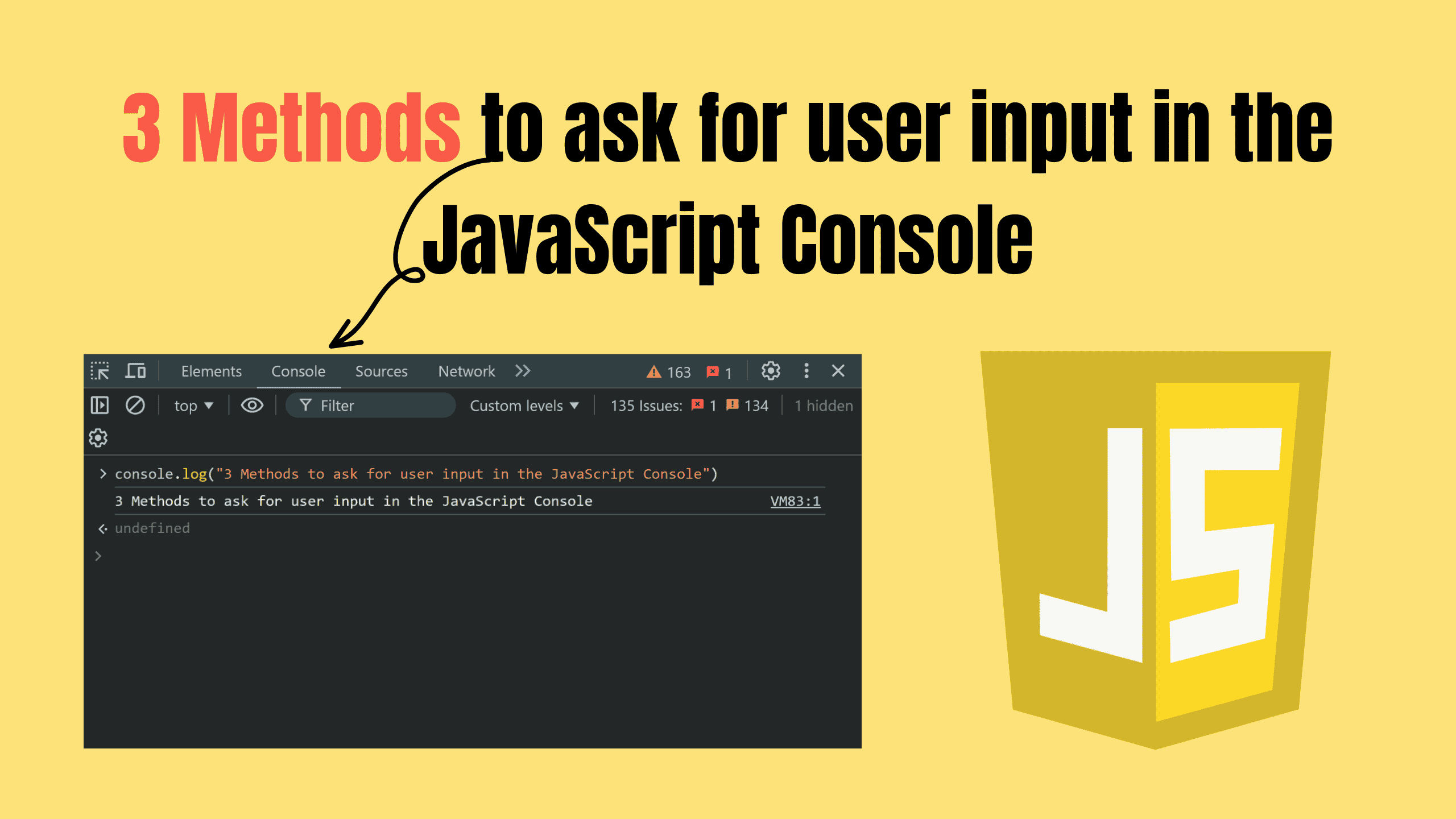
The JavaScript Console is a powerful tool for developers. It’s commonly used for debugging and testing but can also asking user input during development. This is particularly useful for prototyping, debugging, or testing without creating a user interface. This powerful tool is commonly used for:
- Debugging: Identifying and fixing issues in your code.
- Testing: Experimenting with JavaScript code snippets directly.
- Prototyping: Trying out ideas quickly without needing a full development setup.
The console is part of a browser's Developer Tools and can be accessed via shortcuts like
jsconsole.log("This is a message logged to the console.");
But the JavaScript Console isn't just for logging. It can also gather user input, a handy feature when testing, prototyping, or building lightweight scripts. In this blog, we'll explore how to use the JavaScript Console for user input effectively.
What is JavaScript?
JavaScript is one of the most widely used programming languages for building interactive and dynamic websites. Browsers primarily use it to add interactivity, manipulate the DOM (Document Object Model), and enhance user experiences.
However, JavaScript isn’t limited to browsers; it can also run on servers using Node.js.
What is Node.js?
Node.js is a runtime environment that allows developers to execute JavaScript code outside the browser, typically on servers. It’s popular for building scalable and high-performance applications, such as APIs and command-line tools.
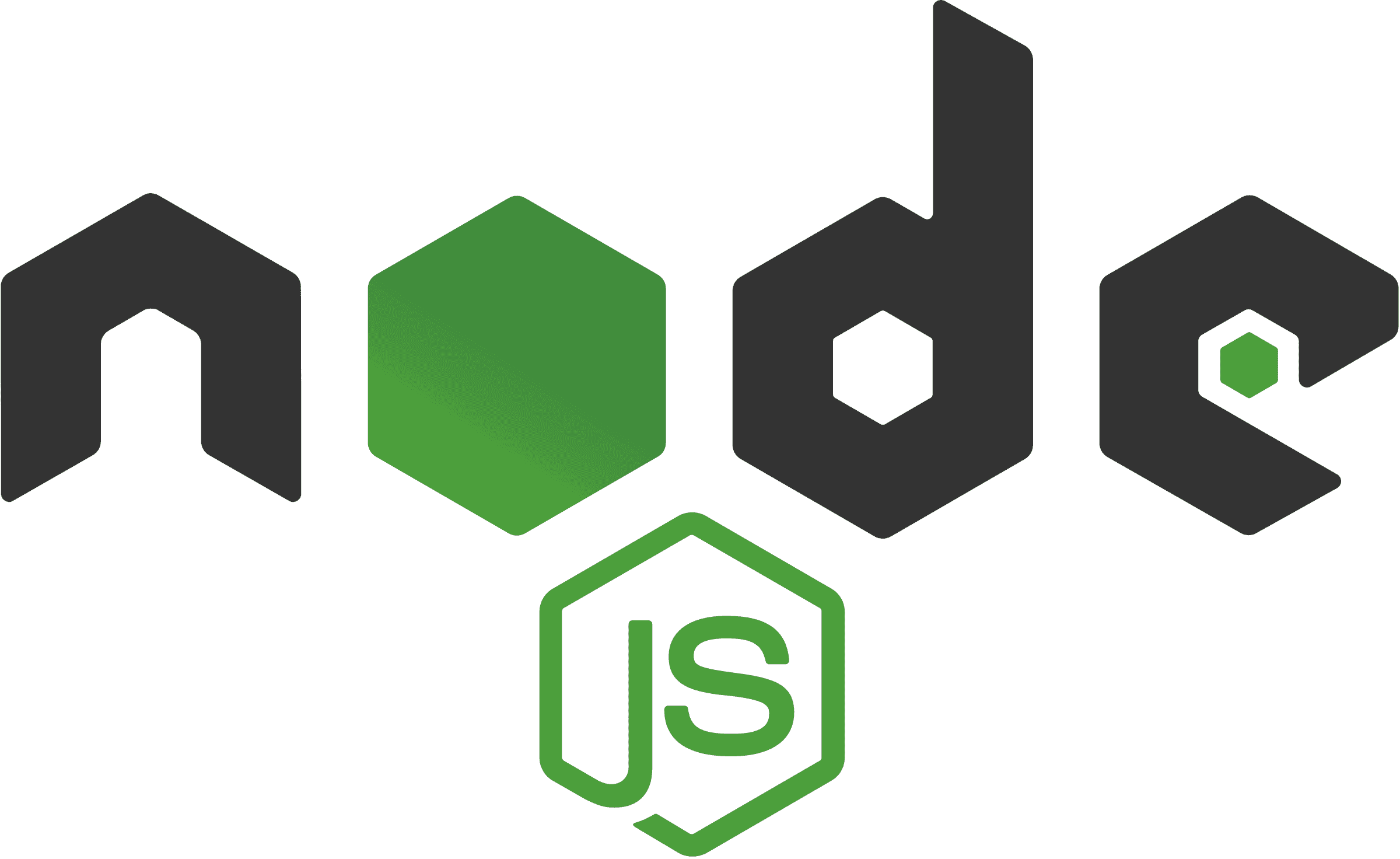
This blog will focus on how JavaScript can gather user input in two environments:
- Browsers
- Node.js
Let’s dive into 3 effective methods for asking users for input using the javascript console.
I’m going to give input when I need to. When it needs to be said. — Kyrie Irving
1. Using prompt() (Browser-Based)
The prompt() function is a simple way to ask for user input directly in the browser. It shows a dialog box with a message and an input field where users can type their responses.
jslet name = prompt("What is your name?"); console.log(`Hello, ${name}!`);
- The browser displays a dialog box with the text “What is your name?” and an input field.
- The user enters their response and clicks “OK.”
- The value is stored in the name variable and logged to the console.
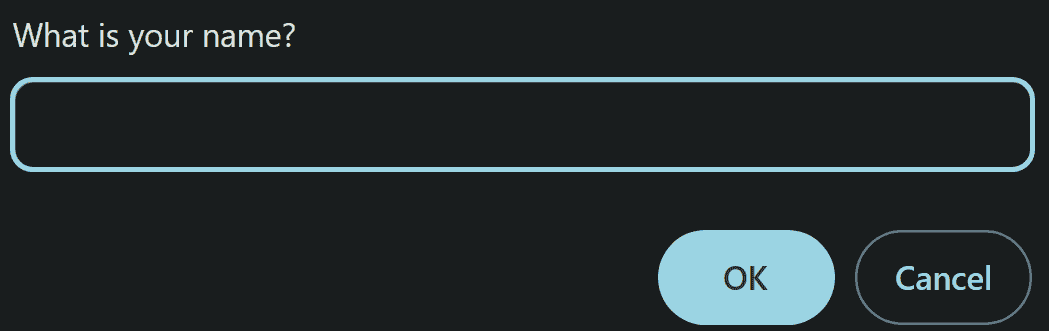
Numeric Input:
Since prompt() returns a string, you need to convert the input if you expect a number.
jslet age = prompt("Enter your age:"); age = parseInt(age, 10); // Converts the string to an integer if (!isNaN(age)) { console.log(`You are ${age} years old.`); } else { console.log("Invalid input. Please enter a valid number."); }
2. Using Event Listeners for Dynamic Input (Browser-Based)
For more interactive input gathering in browsers, you can use event listeners to capture user actions like key presses. This approach is non-blocking and works dynamically during runtime.
jslet input = ""; document.addEventListener("keydown", (event) => { if (event.key === "Enter") { console.log(`Final input: ${input}`); input = ""; // Reset the input after pressing Enter } else { input += event.key; } });
- The addEventListener() method listens for the keydown event.
- Each time a key is pressed, the event object is passed to the callback function.
- The key pressed is logged in the console using event.key.
3. Using readline in Node.js (Server-Based)
In server-side JavaScript, input from the terminal is handled differently. Node.js provides the readline module, which is designed to gather input interactively in a command-line interface.
jsconst readline = require("readline"); const rl = readline.createInterface({ input: process.stdin, output: process.stdout }); rl.question("What is your favorite programming language? ", (language) => { console.log(`You love ${language}.`); rl.close(); });
- The readline.createInterface() function initializes an input/output stream.
- The rl.question() method displays a prompt in the terminal and waits for the user’s response.
- The response is passed as an argument to a callback function.
- The rl.close() method closes the input stream after collecting input.
Summary
Different JavaScript environments offer various methods for handling user input. Each method has its own strengths and weaknesses depending on the environment and use case.
Method | Environment | Advantages | Limitations |
---|---|---|---|
prompt() | Browser | Quick, simple, no setup needed. | Blocks code execution, outdated UI. |
Event Listeners | Browser | Real-time, interactive, dynamic handling. | Requires setup, limited to browser. |
readline (Node.js) | Node.js | Flexible, non-blocking, robust for CLI. | Not browser-compatible. |
Best Practices
- Use Input Validation: Always validate user input to ensure it meets the expected format or type.
- Separate Development and Production Methods: These methods are great for testing or prototyping but are not ideal for production. Use input fields, forms, or UI components in user-facing applications.
- Choose the Right Approach: For browser environments, use prompt() or event listeners depending on the complexity. For Node.js, use the readline module for structured command-line input.
Why Direct Input in the Console Isn’t Possible
Many developers wonder if they can take input directly within the JavaScript Console in a browser, similar to how a command-line interface (CLI) works. While the console is a powerful tool for debugging and executing JavaScript code in real-time, it does not have the capability to capture user input directly in the console itself.
Why This Limitation Exists
- Designed for Output, Not Interaction: The primary purpose of the JavaScript Console is to log outputs, debug code, and provide insights into the running application. It’s not meant to function as CLI.
- No Input Event Handling: Unlike terminal-based applications, the console in browsers doesn’t support input events (like stdin in Node.js) for capturing user responses dynamically. It operates within the context of the browser's runtime and relies on external mechanisms like prompt() dialogs or DOM-based input fields for user interaction.
- Security Considerations: Allowing direct input in the console could introduce potential security risks, such as encouraging users to execute arbitrary code, which malicious actors might exploit.
Alternatives for Browser Input are explained above, by combining such methods, developers can achieve the same functionality without relying on direct console input.
Conclusion
JavaScript offers flexible methods for gathering user input in both browser and Node.js environments. Whether you use the simple prompt() function, interactive event listeners, or the robust readline module, each method has unique strengths tailored for specific scenarios.
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!