Learn 5 Types of Nesting in JavaScript with Examples.
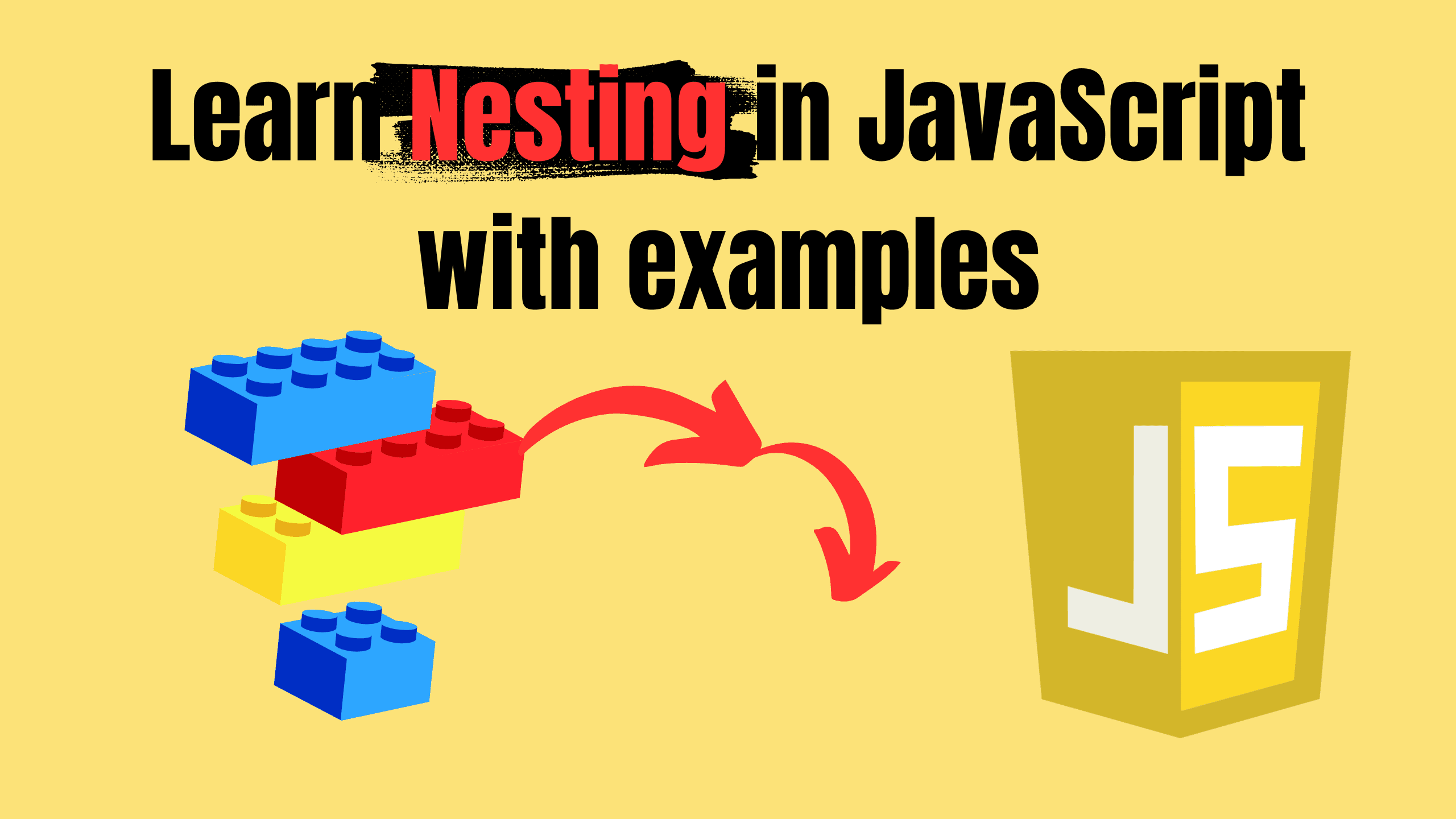
JavaScript is a flexible language that lets you organize your code in creative and effective ways. One technique that can truly elevate your code’s clarity and efficiency is nesting. In this post, we’ll explore what nesting means in JavaScript, dive into its many benefits, and walk through real examples to help you implement it with confidence. Whether you’re just starting out or you’ve been coding for years, understanding how to nest your code properly can make a huge difference.
JavaScript nesting is all about placing one structure inside another—much like fitting smaller boxes within a larger one. This approach allows you to group related functionalities, making your code easier to read and maintain. When you nest functions, arrays, objects, loops, or even conditional statements, you’re essentially building a hierarchy that organizes your logic in a natural and intuitive way.
What is Nesting in JavaScript?
At its simplest, nesting in JavaScript means embedding one code block inside another. Imagine a set of Russian dolls where each doll fits neatly inside a larger one—this is how nesting works in programming. You can nest functions inside other functions, arrays within arrays, and objects inside objects, all to create a more organized and modular codebase.
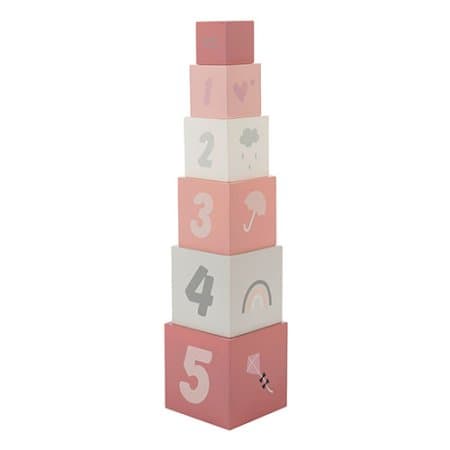
This method of structuring code not only mirrors how we think about problems in the real world but also leverages JavaScript’s scoping rules. By doing so, you can keep related pieces of code together and reduce potential naming conflicts, which makes your overall program much easier to follow and debug.
Why Embrace Nesting? The Benefits
Nesting isn't just about making code look fancy; it offers significant advantages for JavaScript developers:
- Improved Code Organization: Nesting helps group related code together, making it easier to understand the flow and structure of your program. Imagine trying to read a document with all ideas jumbled together versus one organized into sections and sub-sections – nesting brings that much-needed structure to your code.
- Enhanced Readability: Well-nested code is generally more readable. By visually indenting nested blocks, you can quickly grasp the hierarchy and relationships within your code, making it easier for you and other developers to understand and maintain.
- Scope Management: Nesting plays a crucial role in defining scope in JavaScript, particularly with functions. Nested functions have access to the scope of their outer functions, enabling powerful patterns like closures and data encapsulation.
- Code Reusability (in some cases): While not always directly for reusability, nested structures can help create modular and self-contained units of code. For instance, a nested function can act as a helper function specifically for its outer function, reducing namespace pollution.
- Creating Complex Data Structures: Nesting is essential for building complex data structures like multidimensional arrays and objects with intricate relationships, allowing you to model real-world scenarios and manage data effectively.
Types of Nesting in JavaScript: A Detailed Exploration
JavaScript offers several ways to nest your code, each with its own use cases and benefits. In the sections below, we’ll take a closer look at different types of nesting and illustrate them with practical examples.
1. Nesting Functions (Inner Functions)
One of the most common forms of nesting is placing one function inside another. This technique not only keeps helper functions close to where they’re used but also leverages JavaScript’s closure feature, allowing inner functions to access the outer function’s variables.
javascriptfunction counter() { let count = 0; // Private variable (encapsulated) function increment() { // Inner function (private method) count++; return count; } return increment; // Return the inner function to access it from outside } const myCounter = counter(); console.log(myCounter()); // Output: 1 console.log(myCounter()); // Output: 2 console.log(myCounter()); // Output: 3 // console.log(count); // Error! count is not accessible outside counter
This example shows a counter function returning an inner increment function that preserves a private count variable, letting you increase the count with each call without exposing the variable externally.
Key Points about Nested Functions:
- Scope: Inner functions have access to the scope of the outer function, including variables declared in the outer function. This is the concept of closure.
- Accessibility: Inner functions are only accessible within the scope of the outer function. They cannot be called directly from outside the outer function.
- Use Cases:
- Helper Functions: Creating inner functions to perform specific tasks that are only relevant to the outer function, keeping your code organized.
- Encapsulation and Data Hiding: Using inner functions to create private methods and variables, controlling access and preventing accidental modification from outside.
2. Nesting Arrays (Multidimensional Arrays)
Arrays in JavaScript are versatile and can store various data types, including other arrays. This nesting creates multidimensional arrays that are ideal for representing matrices, grids, or any tabular data.
javascriptconst studentGrades = [ ["Alice", [85, 92, 78]], // Student 1: Name and grades ["Bob", [90, 88, 95]], // Student 2: Name and grades ["Charlie", [70, 80, 75]] // Student 3: Name and grades ]; console.log(studentGrades[1][0]); // Output: "Bob" (Name of the second student) console.log(studentGrades[0][1][2]); // Output: 78 (3rd grade of the first student)
Here, each element in the nested array holds a student’s name paired with their grade array, so you can easily access specific details like a student’s name or an individual grade using multiple indices.
Key Points about Nested Arrays:
- Structure: Nested arrays are used to represent tabular data, matrices, or hierarchical structures.
- Accessing Elements: You access elements in nested arrays using multiple indices, one for each level of nesting.
- Use Cases:
- Representing Matrices: Mathematical matrices, game boards, or any grid-like data.
- Hierarchical Data: Storing data with multiple levels of categories or subcategories.
3. Nesting Objects (Objects within Objects)
JavaScript objects are collections of key-value pairs that can also contain other objects. This type of nesting is perfect for modeling real-world entities that have multiple layers of information.
javascriptconst product = { id: "P123", name: "Laptop", details: { // Nested object for details brand: "TechCorp", model: "ProBook X1", specifications: { // Further nested object for specs processor: "Intel i7", memory: "16GB RAM", storage: "512GB SSD" } } }; console.log(product.details.specifications.processor); // Output: "Intel i7"
This snippet demonstrates how to nest objects by embedding a details object (with further nested specifications) inside a product object, making it straightforward to access deep properties like the processor type.
Key Points about Nested Objects:
- Structure: Nested objects are used to represent complex entities with hierarchical properties and relationships.
- Accessing Properties: You access nested properties using dot notation (.) or bracket notation ([]) chained together.
- Use Cases:
- Representing Complex Data: User profiles, configuration settings, API responses, or any data with intricate structures.
- Organizing Related Data: Grouping related properties under logical sub-objects for better organization.
4. Nesting Loops (Loops within Loops)
When you need to perform repeated operations over complex data, nesting loops is a natural solution. A nested loop allows an inner loop to run completely for every iteration of the outer loop, which is particularly useful for iterating over multidimensional arrays.
javascriptconst array1 = [1, 2, 3]; const array2 = [2, 4, 6]; for (let i = 0; i < array1.length; i++) { for (let j = 0; j < array2.length; j++) { if (array1[i] === array2[j]) { console.log(`Common element found: ${array1[i]}`); } } } // Output: // Common element found: 2
In this code, nested loops compare two arrays element by element, printing out a message whenever a common element is found between the two arrays.
Key Points about Nested Loops:
- Iteration: The inner loop runs to completion for every single iteration of the outer loop.
- Time Complexity: Nested loops can increase the time complexity of your code, especially for large datasets. Be mindful of performance implications.
- Use Cases:
- Iterating over 2D Arrays: Processing each element in a matrix or grid.
- Comparing Elements in Two Arrays: Finding common elements or performing pairwise operations between two datasets.
- Generating Combinations or Permutations: Creating all possible combinations of elements from multiple sets.
5. Nesting Conditional Statements (if...else)
Nesting conditional statements can help create more refined decision trees in your code. By embedding one conditional inside another, you can handle multiple scenarios with clarity and precision.
javascriptlet userRole = "admin"; let accessLevel = 3; if (userRole === "admin") { if (accessLevel >= 2) { console.log("Admin access granted."); if (accessLevel === 3) { console.log("Full admin privileges."); } } else { console.log("Insufficient admin access level."); } } else { console.log("Unauthorized access."); }
This conditional structure uses nested if statements to check user role and access level, ensuring that only users with the right privileges receive messages about granted or full admin access.
Key Points about Nested Conditionals:
- Decision Trees: Nested if...else statements create decision trees, allowing you to handle multiple conditions and outcomes.
- Readability Concerns: Deeply nested conditionals can become difficult to read and understand. Strive for shallow nesting or consider alternative control flow structures for complex logic.
- Use Cases:
- Multi-level Validation: Checking multiple conditions in sequence to validate user input or data.
- Complex Decision Logic: Implementing intricate rules and scenarios where different actions are needed based on various factors.
Best Practices for Effective Nesting
While nesting is powerful, excessive or poorly managed nesting can lead to code that is hard to read and debug. Here are some best practices to follow:
- Keep Nesting Levels Shallow: Aim for minimal levels of nesting whenever possible. Deeply nested code can become a "pyramid of doom," making it difficult to follow the logic. Refactor complex nested structures into smaller, more manageable functions or modules.
- Use Meaningful Names: Employ descriptive variable and function names to clearly indicate the purpose of each nested block. This significantly improves readability.
- Break Down Complex Logic: If you find yourself nesting deeply, consider breaking down the inner logic into separate, well-named functions. This promotes modularity and reduces nesting depth.
- Comment Your Code: When nesting is necessary, use comments to explain the purpose and logic of each nested block, especially for complex conditional statements or loops.
- Consider Alternatives to Deep Nesting: For complex conditional logic, explore alternatives like switch statements, lookup tables (objects or maps), or early returns to reduce nesting. For deeply nested loops, consider if there are more efficient algorithms or data structures.
FAQs
- What is nesting in JavaScript? Nesting in JavaScript is the practice of placing one code structure (like functions, arrays, objects, loops, or conditionals) inside another. It's a way to organize code hierarchically.
- Why is nesting useful in JavaScript? Nesting improves code organization, readability, scope management, and enables the creation of complex data structures and functionalities.
- What types of structures can be nested in JavaScript? You can nest functions, arrays, objects, loops (like for and while), and conditional statements (if...else, try...catch) in JavaScript.
- How deep should nesting be? Aim for shallow nesting. Deeply nested code can become hard to read and debug. Refactor complex logic into smaller functions to reduce nesting levels.
- How do I access elements in nested arrays and objects? Use multiple indices for nested arrays (e.g., array[0][1]) and chained dot or bracket notation for nested objects (e.g., object.property1.property2 or object['property1']['property2']).
- Are there performance implications of nesting? Deeply nested loops can increase time complexity. Be mindful of performance, especially with large datasets. Function nesting and object/array nesting generally don't have significant performance overhead unless excessively deep or complex.
- How can I avoid deeply nested code? Break down complex logic into smaller functions, use alternative control flow structures (like switch statements or early returns), and consider if there are more efficient data structures or algorithms for your task.
Conclusion
Nesting is a powerful tool in JavaScript for structuring your code, creating complex data representations, and managing scope effectively. By understanding the different types of nesting and following best practices, you can write JavaScript code that is not only functional but also clean, readable, and maintainable.
Embrace the power of nesting to elevate your JavaScript development skills and create more elegant and efficient applications. Start practicing nesting in your projects today and unlock a new level of code organization and clarity!
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!