Adding a 3D model in Three.js (Beginner Guide 02)
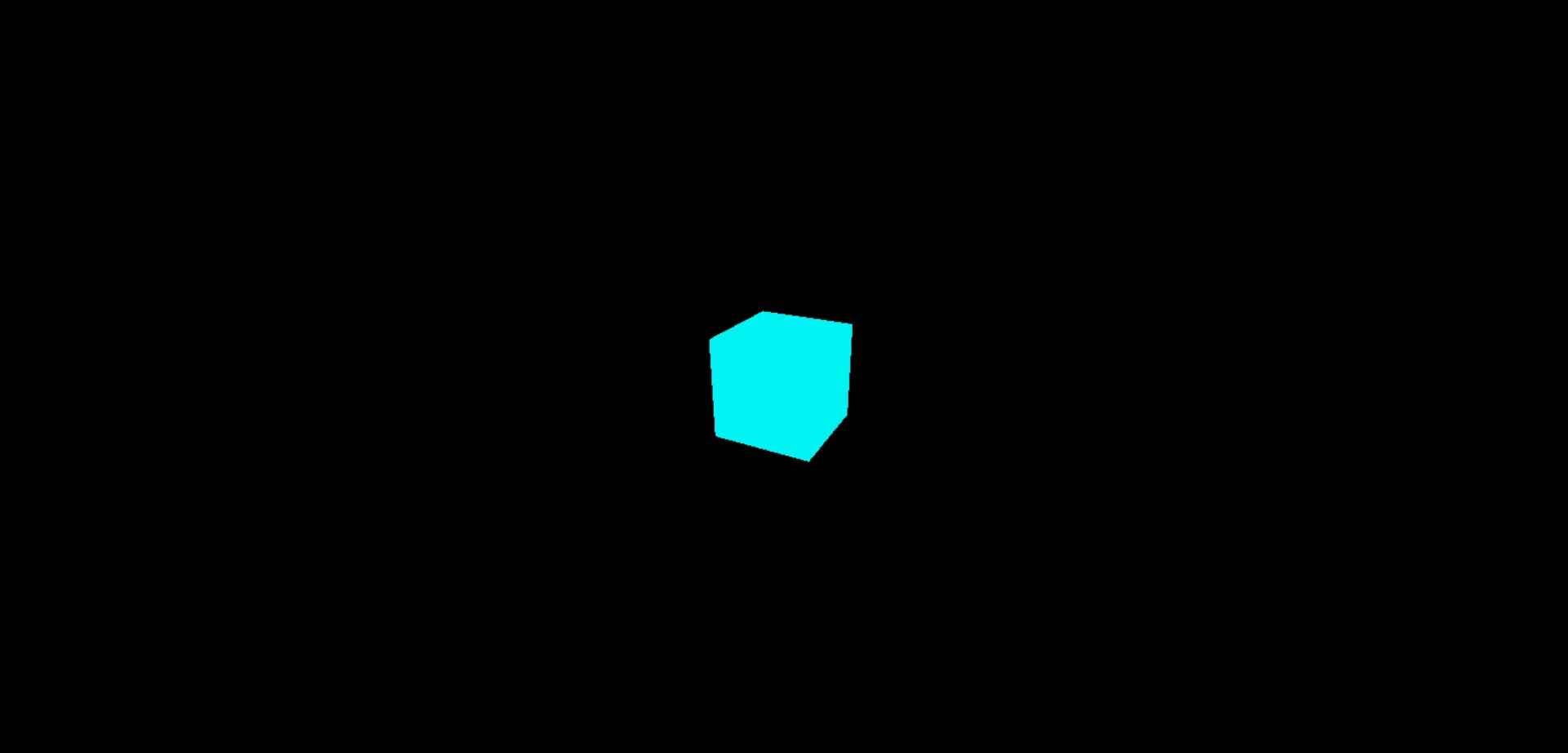
Getting Started
Three.js is a powerful library that allows for easy creation of 3D models in JavaScript. In this blog, we'll walk through the process of creating a simple 3D model using Three.js.
Before you begin, you need to ensure you have a basic understanding of Three.js, as well as a basic setup for a Three.js project. If you haven't already, please refer to our previous blog on setting up a Three.js project.
Creating a 3D Model
To create a 3D model in Three.js, you need to create a mesh. A mesh in Three.js is created by combining a geometry with a material. In this tutorial, we'll create a basic 3D sphere.
js// Create a geometry let geometry = new THREE.BoxGeometry(1, 1, 1); // Create a material let material = new THREE.MeshBasicMaterial({ color: 0xf2f2 }); // Create a mesh let cube = new THREE.Mesh(geometry, material); // Add the cube to the scene scene.add(cube);
This will create a sphere at the center of the scene. You can adjust the size, color, and position of the sphere by adjusting the parameters in the SphereGeometry and MeshBasicMaterial constructors.
Adding Lighting
To add lighting to the scene, we need to create a light source. In this tutorial, we'll create an ambient light.
js// Create an ambient light let ambientLight = new THREE.AmbientLight(0xfffff, 5); // Add the light to the scene scene.add(ambientLight);
Full Code
Check below How the full code will look like:
jsimport './style.css' import * as THREE from 'three'; // Initialize Three.js const scene = new THREE.Scene(); const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000); camera.position.set(2, 2, 4); camera.lookAt(0, 0, 0); const renderer = new THREE.WebGLRenderer(); renderer.setSize(window.innerWidth, window.innerHeight); document.body.appendChild(renderer.domElement); // Create a geometry let geometry = new THREE.BoxGeometry(1, 1, 1); // Create a material let material = new THREE.MeshBasicMaterial({ color: 0xf2f2 }); // Create a mesh let cube = new THREE.Mesh(geometry, material); // Add the cube to the scene scene.add(cube); // Create an ambient light let ambientLight = new THREE.AmbientLight(0xfffff, 5); // Add the light to the scene scene.add(ambientLight); // Render the scene renderer.render(scene, camera);
Conclusion
In this tutorial, we learned how to create a simple 3D model in Three.js. For more details see the other tutorials and guide. If you have any questions or need further clarification, don't hesitate to reach out. Happy coding!
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!