Basic Data Structures in Javascript
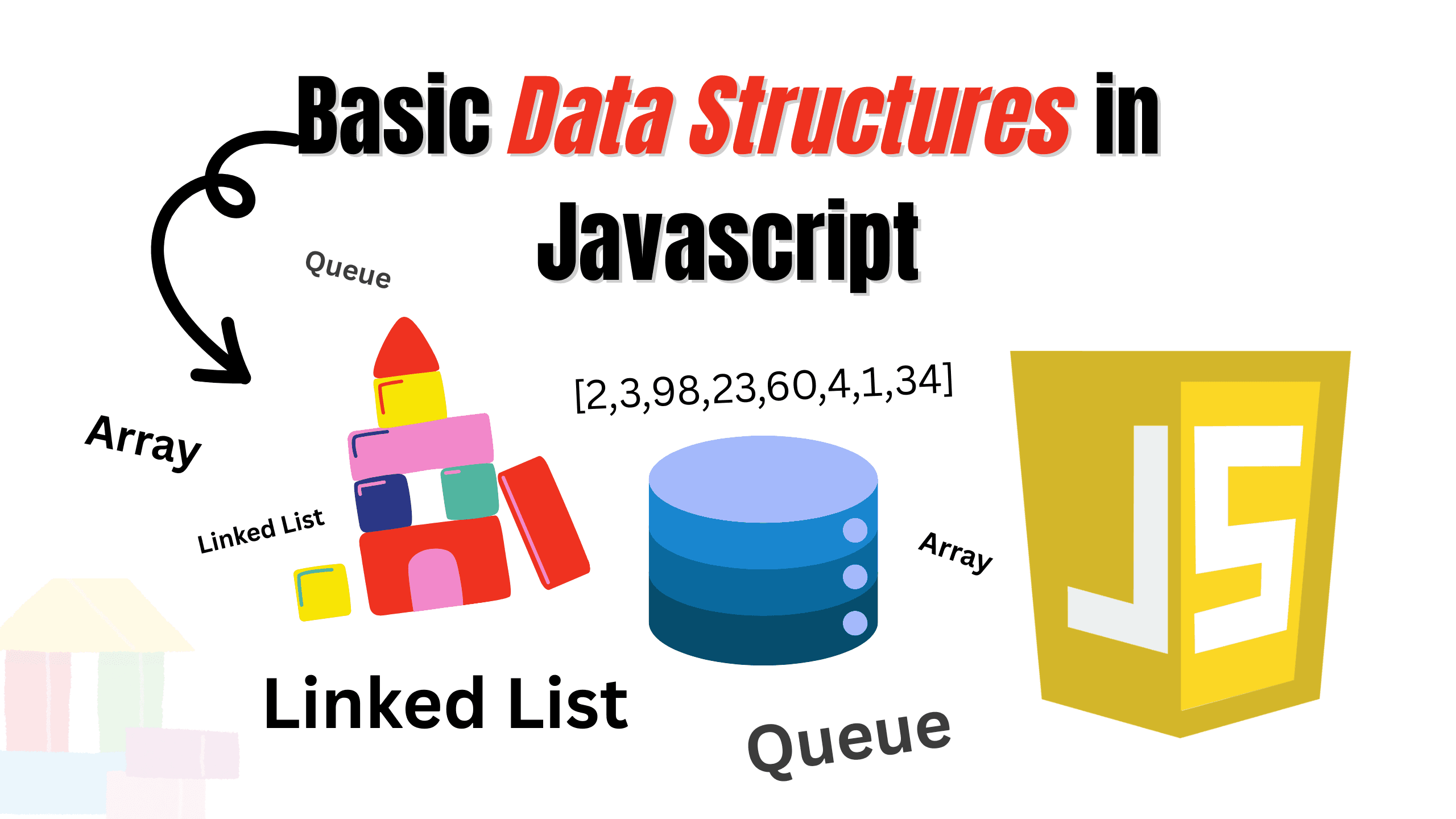
Data structures are the backbone of efficient programming—they provide organized methods for storing, modifying, and accessing data. In this blog, we'll explore key JavaScript data structures and their practical applications, complete with engaging explanations and code examples.
Why Data Structures Matter?
Data structures are essential in almost every facet of computer science, from operating systems to cutting-edge artificial intelligence. They allow us to:
- Manage and process large datasets efficiently
- Quickly search and retrieve specific data
- Design tailored algorithms for specialized programs
- Handle multiple user requests simultaneously
- Simplify and speed up overall data processing
A robust understanding of data structures is crucial not only for optimizing performance but also for tackling real-world programming challenges. It’s no wonder that many top tech companies place a strong emphasis on this knowledge during interviews.
Bad programmers worry about the code. Good programmers worry about data structures and their relationships. Linus Torvalds
JavaScript Data Structures
JavaScript inherently supports both primitive and non-primitive data structures. Primitive types—such as Boolean, null, number, and string—are built into the language. In contrast, non-primitive data structures are often crafted by developers to manage complex tasks. This guide focuses on three essential non-primitive structures: arrays, queues, and linked lists.
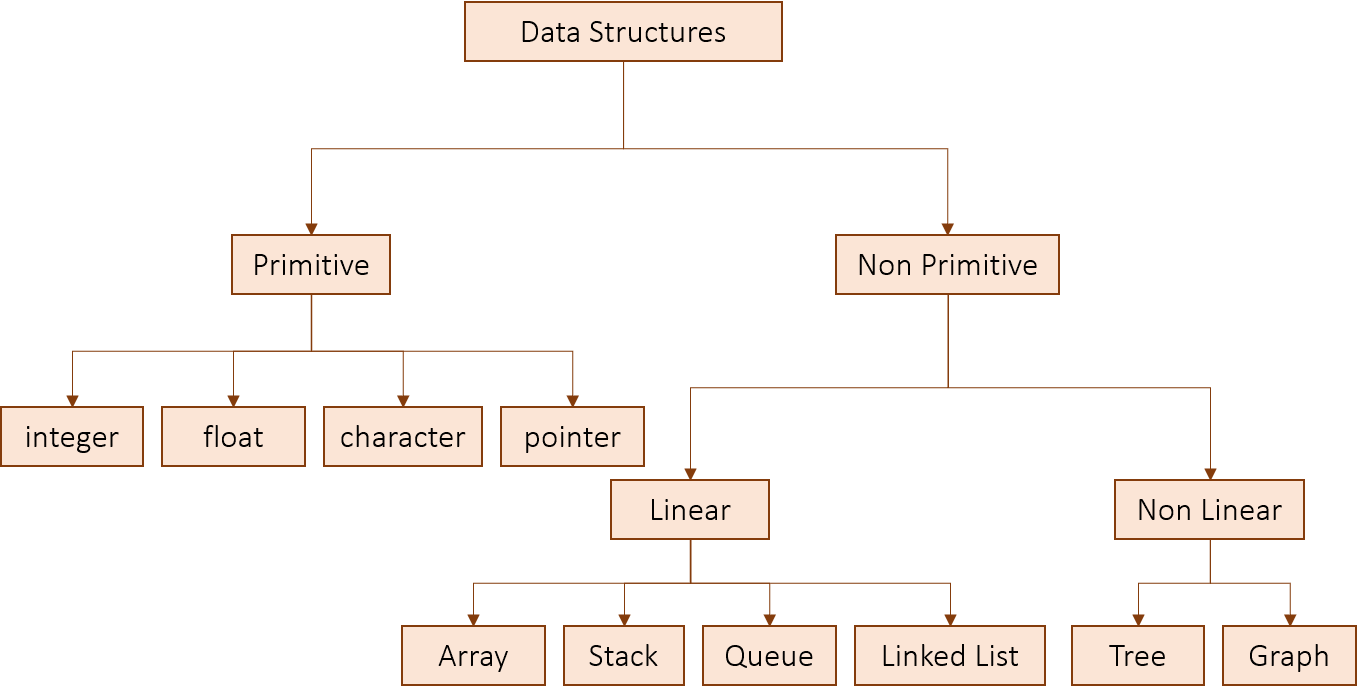
Arrays
Arrays are one of the most fundamental data structures in computer science, and they play a crucial role in JavaScript programming. In JavaScript, an array is used to store a collection of elements, each accessible via its numeric index. This design offers several advantages that contribute to both the performance and flexibility of your code.
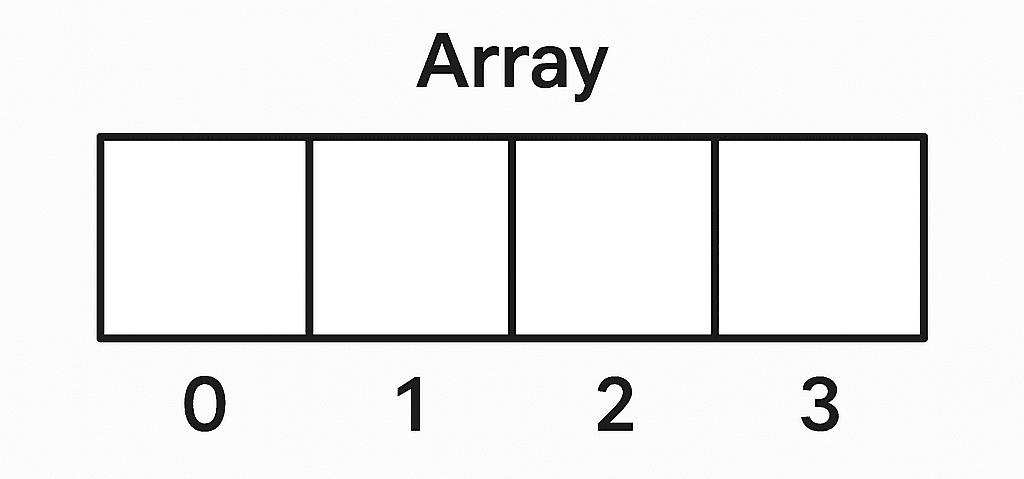
Why Arrays Are Essential
Arrays are designed to hold data in a contiguous block of memory, which means that each element is stored right next to the others. This property enables several benefits:
- Rapid Index-Based Access: Since elements are stored sequentially, accessing an element by its index is extremely fast. For instance, retrieving the third element in an array can be done directly without the need to traverse other elements.
- Efficient Data Manipulation: Arrays allow for straightforward addition, removal, and modification of elements. With built-in methods like push(), pop(), shift(), and unshift(), managing collections of data becomes much more intuitive.
- Predictable Performance: The contiguous storage of arrays ensures predictable memory allocation and access times, which is particularly beneficial for performance-critical applications.
- Versatility in Use Cases: Arrays are not only used for simple lists but also form the basis for more complex data structures. They are fundamental in implementing stacks, queues, and even matrices.
js// Creating and using an array in JavaScript const fruits = ['apple', 'banana', 'cherry']; // Accessing an element by index console.log(fruits[1]); // Output: banana // Adding an element fruits.push('date'); console.log(fruits); // Output: ['apple', 'banana', 'cherry', 'date'] // Iterating over an array fruits.forEach((fruit, index) => { console.log(`Index ${index}: ${fruit}`); });
Queues
A queue is an example of a linear data structure, or more abstractly a sequential collection. Queues are common in computer programs, where they are implemented as data structures coupled with access routines, as an abstract data structure or in object-oriented languages as classes.
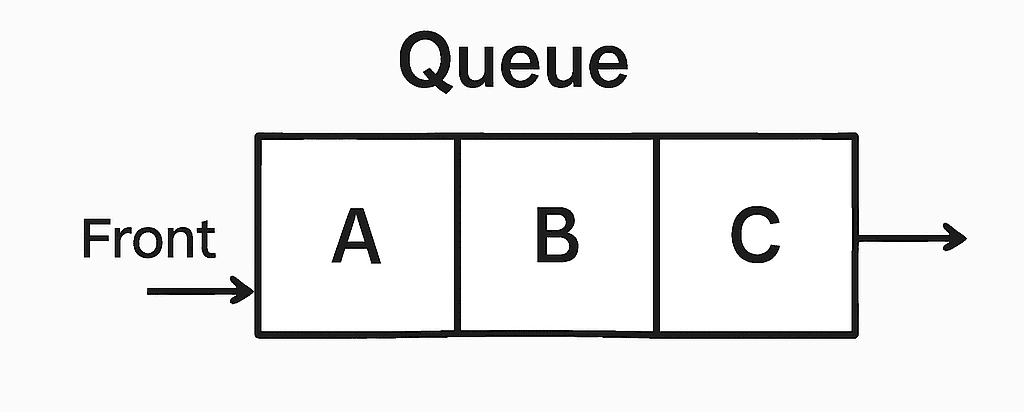
Key Characteristics of Queues
Queues have several defining features that make them particularly useful in many programming scenarios:
- FIFO Principle: Elements are processed in the exact order they are added, ensuring fairness in task management.
- Linear Structure: The data is arranged in a straight line, where every element has a specific position.
- Dynamic Management: Queues can easily accommodate varying sizes of datasets, making them adaptable to changing workloads.
- Efficient Operation: With simple operations for insertion (enqueue) and deletion (dequeue), queues are efficient in both time and space.
jsclass Queue { constructor() { this.items = []; } // Add an element to the queue enqueue(element) { this.items.push(element); } // Remove an element from the queue dequeue() { if (this.isEmpty()) { return "Underflow"; } return this.items.shift(); } // Check if the queue is empty isEmpty() { return this.items.length === 0; } // View the first element front() { if (this.isEmpty()) { return "No elements in Queue"; } return this.items[0]; } } // Usage const queue = new Queue(); queue.enqueue(10); queue.enqueue(20); console.log(queue.front()); // Output: 10 console.log(queue.dequeue()); // Output: 10
Linked Lists
Linked lists are a powerful, dynamic data structure that allow for flexible memory management and efficient modifications. Unlike arrays, which rely on contiguous memory allocation, linked lists consist of individual nodes that are connected via pointers. Each node contains data and a reference to the next node, enabling the list to grow or shrink as needed without having to allocate or reallocate a large block of memory.
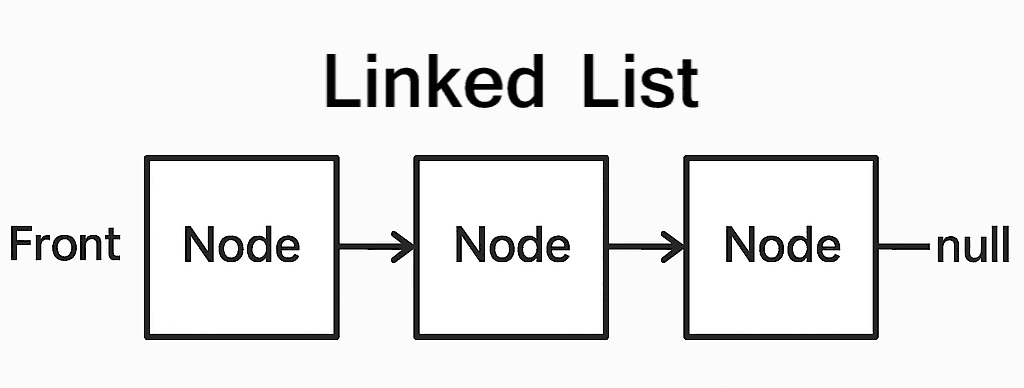
Why Linked Lists Are Valuable
Linked lists offer several advantages that make them a preferred choice in scenarios where data is frequently inserted or removed. Here are some key benefits:
- Dynamic Memory Allocation: Nodes can be allocated or deallocated on the fly and no need for large contiguous memory spaces.
- Efficient Insertions and Deletions: Adding or removing a node does not require shifting the rest of the elements. Ideal for applications where the dataset size is constantly changing.
- Flexible Data Management: Nodes can be easily rearranged, making linked lists adaptable for various data organization tasks. They serve as the basis for other complex data structures, such as stacks and queues
js// Node class for Linked List class Node { constructor(data) { this.data = data; this.next = null; } } // Linked List class class LinkedList { constructor() { this.head = null; } // Insert a node at the end append(data) { const newNode = new Node(data); if (!this.head) { this.head = newNode; return; } let current = this.head; while (current.next) { current = current.next; } current.next = newNode; } // Print the linked list printList() { let current = this.head; while (current) { console.log(current.data); current = current.next; } } } // Usage const list = new LinkedList(); list.append(5); list.append(10); list.append(15); list.printList(); // Output: 5, 10, 15 (each on a new line)
Conclusion
JavaScript's data structures—arrays, queues, and linked lists—each offer unique advantages for managing data. Whether you're handling user requests, processing large datasets, or building complex algorithms, mastering these structures is key to efficient and effective programming.
By integrating these data structures into your projects, you can significantly improve performance and create scalable, robust applications. Keep experimenting with the examples above and explore further to unlock the full potential of JavaScript in solving real-world problems.
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!