Time Events in JavaScript: A Guide
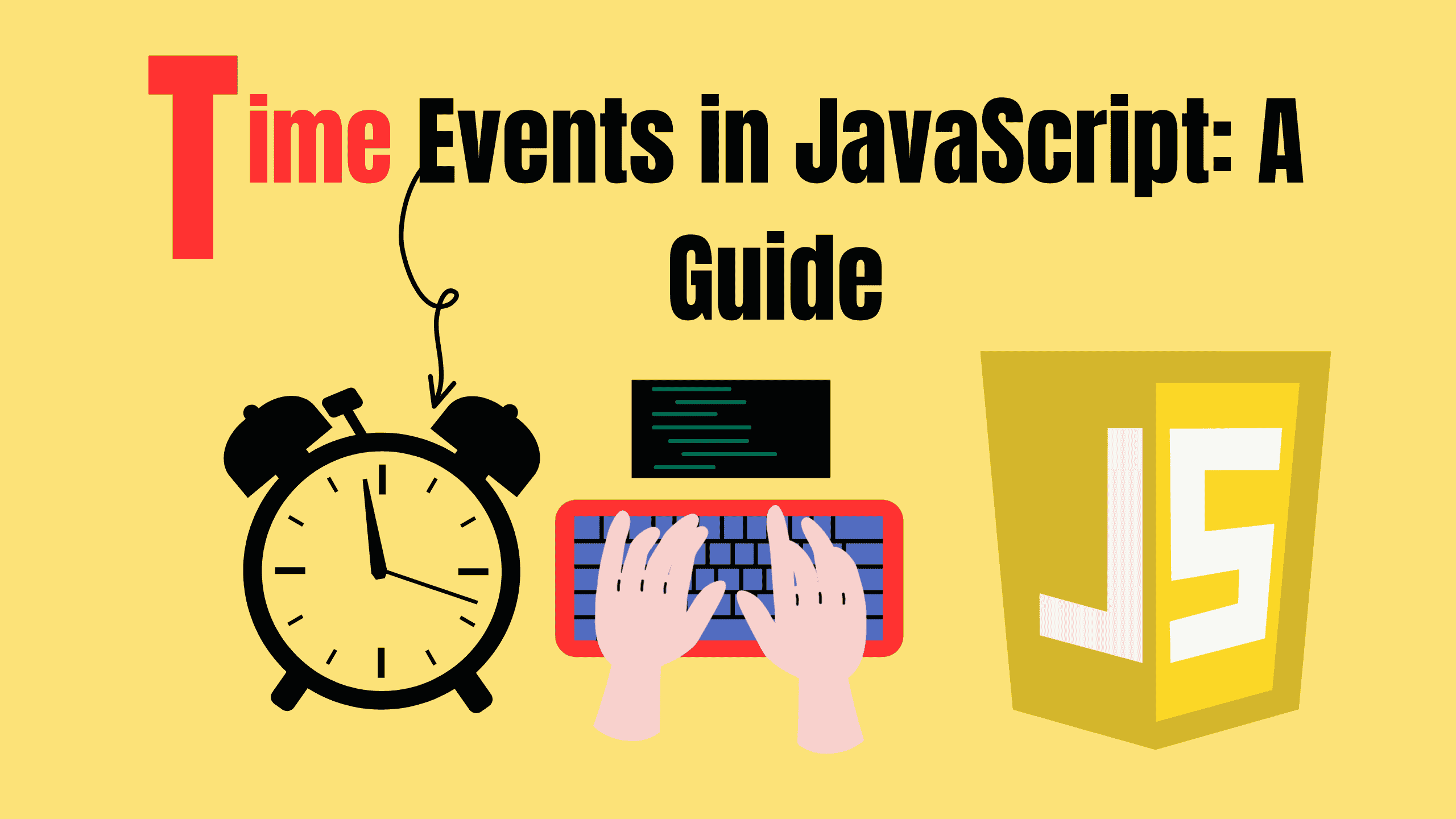
JavaScript is amazing, isn’t it? It makes websites interactive and alive. One of its coolest features is Time Events. Don’t worry if it sounds technical; I’ll break it down.
Let’s explore how you can make things happen after some time or repeatedly. It’s easier than you think!
What Are Time Events?
The window object allows execution of code at specified time intervals. These time intervals are called timing events. Imagine you want something to happen after a few seconds, like showing a message. Or maybe you want something to repeat, like a ticking clock. JavaScript has got your back with setTimeout() and setInterval().
Time waits for no one. — Folklore
1. setTimeout(): Do It Once, After a While
This one is simple. It waits for a specific time, then does something.
jssetTimeout(() => { console.log("Hello after 3 seconds!"); }, 3000); // Time in milliseconds (3 seconds)
Cool, right? It’s like saying, “Hey JavaScript, wait for 3 seconds, then run this.”
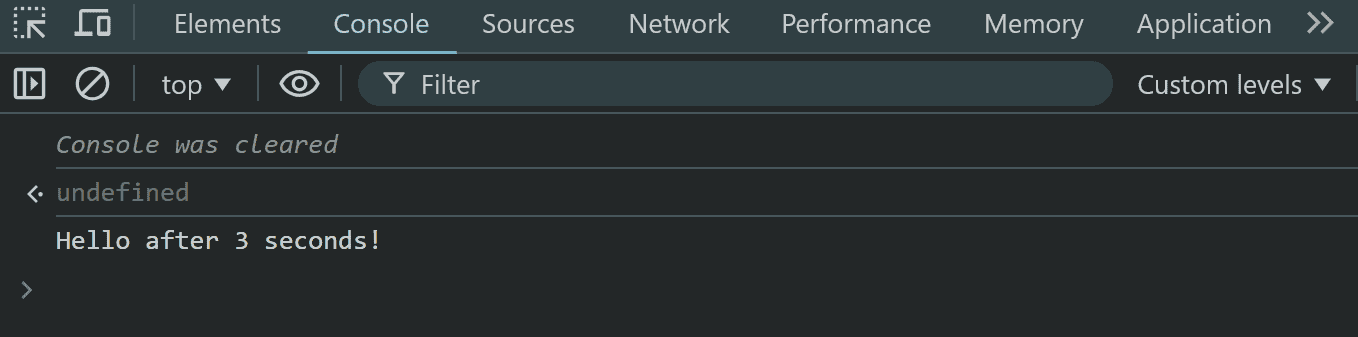
Use Cases:
- Updating a live clock.
- Auto-refreshing data.
2. setInterval(): Do It Again and Again
Want something to repeat? Use setInterval(). It keeps running your code at regular intervals.
jssetInterval(() => { console.log("Tick-tock, every 2 seconds!"); }, 2000); // Runs every 2 seconds
It’s like a loop with a timer. Just remember, it won’t stop on its own unless you tell it to.
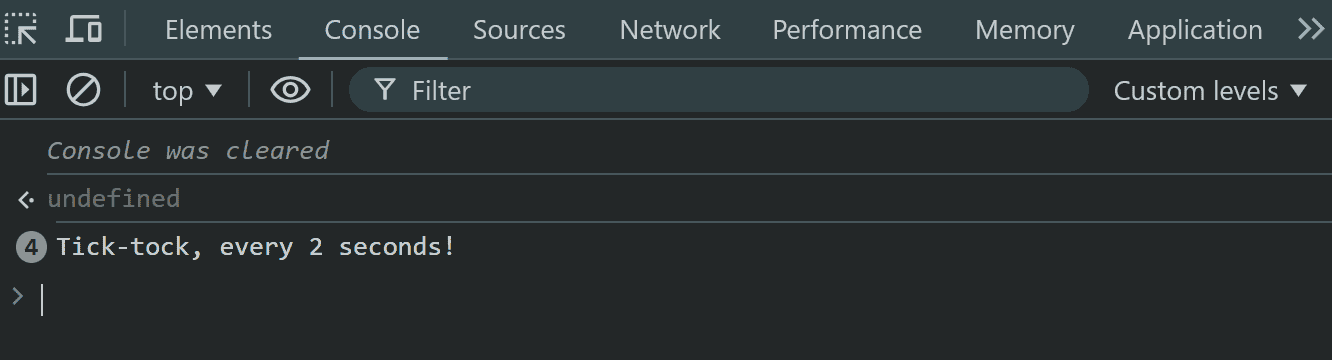
Use Cases:
- Updating a live clock.
- Auto-refreshing data.
3. Stopping Intervals with clearInterval()
Sometimes, you need to stop the madness. Here’s how you can stop a repeating task:
jsconst intervalId = setInterval(() => { console.log("This will stop soon!"); }, 1000); setTimeout(() => { clearInterval(intervalId); console.log("Stopped!"); }, 5000); // Stops after 5 seconds
Here, we save the interval’s ID and use clearInterval() to stop it after 5 seconds. Simple!
Real-life example: A Countdown Timer
Let’s combine these to create a countdown timer and start with any number (5 4 3 2 1):
jslet countdown = 5; const timer = setInterval(() => { console.log(countdown); countdown--; if (countdown < 0) { clearInterval(timer); console.log("Time’s up!"); } }, 1000); // Runs every second
Doesn’t it feel rewarding to see how these pieces come together?
A Quick Warning ⚠️
- setTimeout() and setInterval() aren’t precise. They might lag if the browser is busy.
- Don’t overuse them; too many timers can slow things down.
Can I stop setTimeout()?
While you can’t stop a setTimeout() outright, you can cancel it before it executes by using its ID with clearTimeout().
jsconst timeoutId = setTimeout(() => { console.log("This won't run if we clear the timeout."); }, 3000); // Cancel the timeout clearTimeout(timeoutId);
If you call clearTimeout() before the 3 seconds are up, the setTimeout function is stopped, and the code won’t execute.
Other Key Points:
- The setTimeout() and setInterval() methods share the same pool for storing IDs, which means that we can use the clearTimeout() and clearInterval() methods interchangeably. However, we should avoid doing so.
- The browsers that support the setTimeout() and setInterval() methods are mainly, Google Chrome, Internet Explorer, Safari, Opera, and Firefox.
- When you don’t need to use the clearTimeout() or clearInterval() method, then there is no need to store the ID returned by the setTimeout() or setInterval() method respectively.
Conclusion
Time events are like JavaScript’s secret superpower. They let you control when things happen, making your site feel dynamic and alive. Try them out, experiment, and have fun!
What will you create next? 😊
Follow and Support me on Medium and Patreon. Clap and Comment on Medium Posts if you find this helpful for you. Thanks for reading it!!!